Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial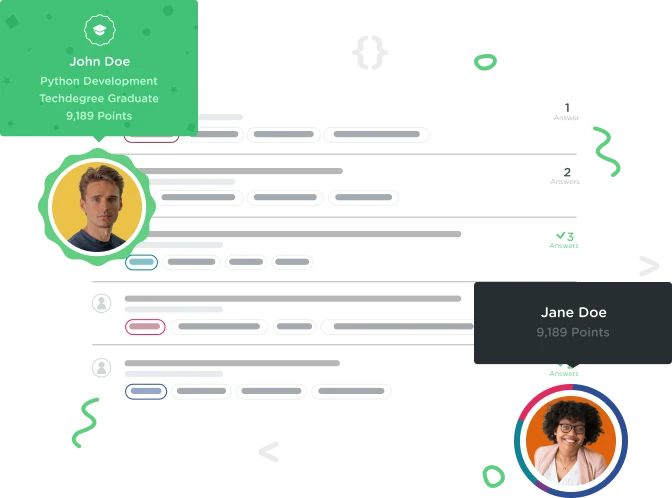

Diego Marrs
8,243 Points'Extends'
Hello,
I know I should have asked this a long time ago but the 'Extends' keyword in Java has been bothering me. I still don't understand what it does and when you could use it in a program.
Thanks!
1 Answer

Tom Finet
7,027 PointsSay we have two classes, MainClass and SubClass in separate files:
public class MainClass {
public MainClass() {
// Do something in constructor.
}
public int add(int a, int b) {
return a + b;
}
}
public class SubClass extends MainClass {
public SubClass() {
super(); // Calls the superclass constructor, in this case the constructor in MainClass.
}
public int multiply(int a, int b) {
return a * b;
}
public static void main(String args[]){
SubClass subClass = new SubClass;
int sum = subClass.add(2, 7);
int product = subClass.multiply(3, 9);
System.out.println("sum: "+ sum + " product: " + product);
}
}
Now imagine that MainClass defines properties and behaviours that SubClass wants to inherit. SubClass will extend MainClass making it a child class of MainClass. A subclass, like SubClass, inherits all the members (fields, methods, and nested classes) from its superclass. It does not inherit constructors as these are not members, so they are not inherited by subclasses, but the constructor of the superclass can be invoked from the subclass using the keyword super.
An example of this in the android framework is the View class and the TextView class. In this example, TextView extends View and inherits all of its members. The View class only holds general members that all views share in common and the TextView is a more specific view so should still extend View and contain more members that are more specific to TextView.
Hope this helps, happy coding!