Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial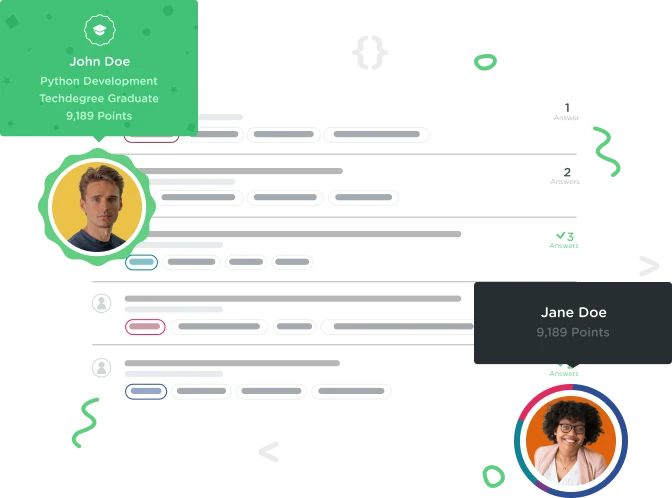
Chuen Hong See
Courses Plus Student 2,693 PointsExtra brainwork: The Student Record Search Challenge
I have tried my best to make this as efficient as possible. It combines listing all students with listing only queried students , and showing them separately. Please advice if I have made any mistake or you guys have a better idea. Thank you.
/*******************************
FUNCTION
*******************************/
/* Capitalize the first letter */
function capFirst(string) {
return string.charAt(0).toUpperCase() + string.slice(1);
}
/* Generate and return HTML code */
function printHTML(i) {
var html = "";
html += '<h2>Student No.' + (i + 1) + ':</h2>'; /* Student ID based on the array */
html += '<ol>';
for (var key in students[i]) {
html += '<li>' + capFirst(key) + ': ' + students[i][key] + '</li>';
}
html += '</ol>';
return html;
}
/* ---- Logic Part -------
Scan through all elements in the array
IF input is 'list' (means listing all students)
Generate all students info
OR input is a valid student name
Generate specific student's info
ELSE
Variable html remains blank
END IF
Return variable: html
---------- END -------- */
function search(input) {
var html = "";
for (var i = 0; i < students.length; i++) {
if ((input !== 'list' && input === students[i].name) || input === 'list') {
html += printHTML(i);
}
}
return html;
}
/* Print out HTML code to div left (outputList) or div right (outputSearch) */
function print(output, message) {
var output = document.getElementById(output);
output.innerHTML = message;
}
/*******************************
EXECUTE
*******************************/
var outputHTML = "";
/* List down all students info */
print('outputList', search('list'));
/* Search student's profile */
while(true) {
var input = prompt("Search student records: type a name [Jody] (or type 'quit' to end)");
if (input === 'quit') { /* Exit query */
break;
}
outputHTML += search(input);
print('outputSearch', outputHTML);
}
1 Answer

Iain Simmons
Treehouse Moderator 32,305 PointsGood work with the functions. I had something similar, though I was allowing for names that contained the search term, and had separate functions for building the HTML of both the overall list of matching students and an individual student.
Here's mine:
var html = '';
var query;
function print(nodeID, message) {
document.getElementById(nodeID).innerHTML = message;
}
function buildDefinitionList(obj) {
var prop;
var dListHTML = '<dl>';
for (prop in obj) {
dListHTML += '<dt>' + prop.charAt(0).toUpperCase() + prop.slice(1) + '</dt>';
dListHTML += '<dd>' + obj[prop] + '</dd>';
}
dListHTML += '</dl>';
return dListHTML;
}
function buildStudentList(list) {
var i;
var uListHTML = '<ul>';
for (i = 0; i < list.length; i++) {
uListHTML += '<li>' + buildDefinitionList(list[i]) + '</li>';
}
uListHTML += '</ul>';
return uListHTML;
}
function getMatchingStudents(list) {
var i;
var resultList;
// keep looping until the user quits
while(true) {
// reset the result list
resultList = [];
// prompt and convert to lowercase
query = prompt("Search student records: type a name [Iain] (or type 'quit' to end)").toLowerCase();
// check if they want to quit
if (query === 'quit') {
break;
}
// loop through students and check if the name contains the search query
for (i = 0; i < list.length; i++) {
if (list[i].name.toLowerCase().indexOf(query) > -1) {
// if so, push to the result list
resultList.push(list[i]);
}
}
// if there are results
if (resultList.length > 0) {
// build the list of matching students
html = buildStudentList(resultList);
} else {
// otherwise print a friendly not found message
html = '<p>Sorry, there are no students matching that name</p>';
}
// print html to output div
print('output', html);
}
}
getMatchingStudents(students);