Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial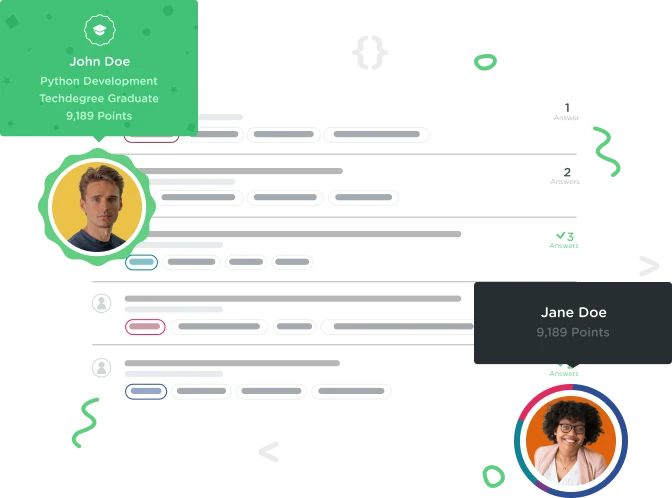
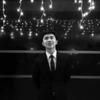
Raphael Chen
Front End Web Development Techdegree Student 8,751 PointsExtra Coding Challenge
It seems that the program doesn't print out the correct message when the correct name has been keyed in. I'm trying to figure out the approach to code for when an incorrect name is keyed in, a message will be printed saying that the incorrect name is not found in the records.
This is the code that I have written:
var message = '';
var student;
var studentName;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
studentName = prompt("Search student records: type a name [Jody] (or type 'quit' to end");
if (studentName === null || studentName.toLowerCase() === "quit") {
break;
} else if (studentName === "") {
message = 'No student name " ' + studentName + '" found in the student records. Try again.';
print(message);
} else {
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name === studentName) {
message = getStudentReport(student);
print(message);
} else {
message = 'No student name "' + studentName + '" found in the student records. Try again.';
print(message);
}
}
}
}
1 Answer

KRIS NIKOLAISEN
54,971 PointsYou could set a flag to false before your loop and update to true if a student was found. Then after the loop check the flag to determine if you should print the not found message.
var message = '';
var student;
var studentName;
var wasFound = false
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
studentName = prompt("Search student records: type a name [Jody] (or type 'quit' to end");
if (studentName === null || studentName.toLowerCase() === "quit") {
break;
} else if (studentName === "") {
message = 'No student name " ' + studentName + '" found in the student records. Try again.';
print(message);
} else {
wasFound=false
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name === studentName) {
message = getStudentReport(student);
print(message);
wasFound=true
}
}
if (!wasFound) {
message = 'No student name "' + studentName + '" found in the student records. Try again.';
print(message);
}
}
}
Raphael Chen
Front End Web Development Techdegree Student 8,751 PointsRaphael Chen
Front End Web Development Techdegree Student 8,751 PointsThank you very much for your help, Kris.