Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial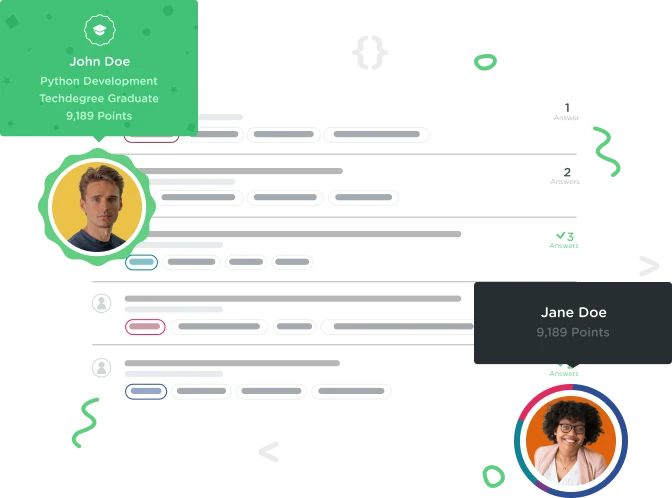

Chepu Lee
1,007 PointsExtra Credit
Hello everyone, I've tried to solve this Extra Credit problem, but still don't have any idea...
"Write a function that accepts a Dictionary as parameter and returns a named tuple. The dictionary should contain the following keys: title, artist and album."
Would you please help me or give me some clues? Thanks a lot :)
1 Answer
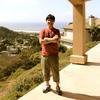
Richard Lu
20,185 PointsHey Chepu,
Here's my approach to the problem assuming that the dictionary passed from the parameter has all three of these keys, and the dictionary is of type [String: String].
func afunction(aDict: [String: String]) -> (title: String, artist: String, album: String) {
// This function could of been written in one line, but i've separated it to make it more clear.
// if it were to be in one line it would look like this
// return (aDict["title"]!, aDict["artist"]!, aDict["album"]!)
let title = aDict["title"]!
let artist = aDict["artist"]!
let album = aDict["album"]!
return (title, artist, album)
}
otherwise, another solution would be
func afunction(aDict: [String: String]) -> (title: String?, artist: String?, album: String?) {
// This function could of been written in one line, but i've separated it to make it more clear.
// if it were to be in one line it would look like this
// return (aDict["title"], aDict["artist"], aDict["album"])
let title = aDict["title"]
let artist = aDict["artist"]
let album = aDict["album"]
return (title, artist, album)
}
if there are any questions, feel free to ask! :)
Ryan Watson
1,472 PointsRyan Watson
1,472 PointsQuick question - the first solution includes a "!" after each of the declared constants in the function, and the second a "?" after the tuple names. Just not following the rationale there (possible we've just not covered it yet in the course).
Richard Lu
20,185 PointsRichard Lu
20,185 PointsHey Ryan,
Short answer:
! - never nil and ? - may either be a nil or some value
Here's a simple example
Note this since they do speak of it in the videos
var (x, y) : (Int?, Int?)
and
var z : (Int, Int)?
are not the same
Let me know if that makes sense. Happy coding! :)