Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial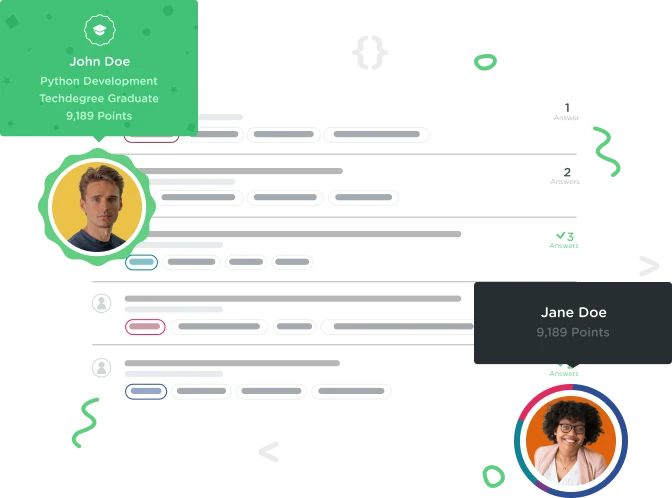

Liz Karaffa
17,576 PointsExtra Credit: Adding a Search Bar to Every Page
Below is the extra credit I'm working on.
"Mike would like us to add a search box on every page.
Determine the best place in the design for this (header? footer? somewhere else?).
Add the HTML and CSS to create the form in the appropriate place in the template.
Code the form so that it is still submitted to [/search/] with a GET variable."
I turned the html code for the search page into an include file and included it on each of the pages where I want it. All I have left to do is to code the form so that it submits to the search page with a GET variable. I'm unsure where to start.
Right now the search items appear below the search bar exactly as they do on the search page.
4 Answers
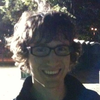
Ben Rubin
Courses Plus Student 14,658 PointsI assume you have something like
<form method="get" action=[what goes here?]>
<input type="text" name="s" value="<?php echo htmlspecialchars($search_term) ?>">
<input type="submit" value="Go">
</form>
in your header.php (or footer.php), right? To put the search term into the search page URL, you just need to append /?s=your_term
to the end of the search page's URL. You can do that like this:
<form method="get" action=<?php echo BASE_URL . "search/?s=" . $search_term; ?>>
<input type="text" name="s" value="<?php echo htmlspecialchars($search_term) ?>">
<input type="submit" value="Go">
</form>
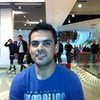
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsHi LIz
Ben's example code would work just fine . If i were you I would have the search box in the header and may be floated to right. Then just point the form's action to your search page passing in the get variable eg yourform?s=textbox value where s is the get variable you will access on your search page.

Liz Karaffa
17,576 PointsAndreas,
Thanks for commenting as well. I'm still not super confident in how $_GET works and what it does. I know $_POST is better for sending data and $_GET for retrieving, but unless I'm told to where and how to use it, I don't really know what to do with it. When read about it on php.net I didn't get much clarity.
Is this what you were explaining?
<form method="get" action=/search/$_GET["s"]>
<input type="text" name="s" value="<?php echo htmlspecialchars($search_term) ?>">
<input type="submit" value="Go">
</form>
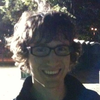
Ben Rubin
Courses Plus Student 14,658 Points$_GET is a PHP method that lets you retrieve the parameters that were passed in the URL. For example, the search/index.php page uses $_GET["s"] to get the "s" parameter from the URL. If your URL is http://localhost/shirts4mike/search/index.php?s=blue then $_GET["s"] gives you the value "blue".
All of that logic is already taken care of in the code behind the search/index.php page. The search page has this code:
$search_term = "";
if (isset($_GET["s"]))
{
$search_term = trim($_GET["s"]);
if ($search_term != "")
{
require_once(ROOT_PATH . "inc/products.php");
$products = get_products_search($search_term);
}
}
That code gets the s parameter from the URL, grabs the matching products from the products array, and populates $products with those products. So all you need to do to make it work for the search bar is to have the form that is part of the search bar go to the appropriate URL (http://localhost/shirts4mike/search/index.php or whatever) and append the s parameter to the end of the URL. That's what action=<?php echo BASE_URL . "search/?s=" . $search_term; ?>
does.
Since the file is the default index.php file, it can be left out of the URL. The URL http://localhost/shirts4mike/search/?s=blue will give you the same results as http://localhost/shirts4mike/search/index.php?s=blue

Liz Karaffa
17,576 PointsThanks Ben! I am truly super grateful for all your help! I wrote you a comment earlier to your initial solution [it's the code I'm using now :)]. However, I don't see it posted above so I'm not sure if you got it. I'm finding that I need a lot of practice because while I understood what was happening in the videos, now that I'm on my own, unless it's spelled out for me, I don't really know how to do it. I've definitely got a lot of holes in my abilities to produce.
The code you provided makes complete sense to me, all it's really doing is adding the search index.php before the "?s=[whatever the user searched]." It's simple concatenation to splice in that file. I've finally grasped that action in a form is where the website will direct to after the form is submitted.
What would be the purpose of using a $_GET to go to the search page instead of the code you provided? Your way seems the most simple and straight forward. And how would you use a $_GET variable to go the search page.
Additionally, when you see ?= in a URL that means that either a $_GET or a $_POST was used at some point in the code correct?
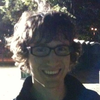
Ben Rubin
Courses Plus Student 14,658 PointsI never got what you wrote, so it must have gotten lost. Oh well. And don't worry that it doesn't make perfect sense the first time through. No one understand everything completely the first time through anything new. It's like math class in school. I understood what the teacher was doing in class, but I was completely lost when I had to do it myself.
You can't use $_GET to go somewhere. $_GET doesn't actually do anything on your page; it's just a function that lets you get parameters that were passed into the URL for your page. When you see the ? in a URL, such as http://localhost/shirts4mike/search/index.php?s=blue
, each thing after the ? is a GET parameter along with its value. In the URL http://localhost/shirts4mike/search/index.php?s=blue
, you have one parameter called "s" with a value of "blue". You can also have multiple parameters separated by an ampersand, such as http://localhost/shirts4mike/search/index.php?s=blue&something=red&somethingelse=5
. In that example, you have a parameter called "s" that has the value "blue", a parameter called "something" that has the value "red", and a parameter called "somethingelse" that has the value "5".
When you submit a form, it can either be submitted using a GET or a POST. Doing a GET will put each parameter in the URL, as we've been doing. Using POST means that your parameters get submitted in the request, but you won't see them in the URL. PHP has a corresponding $_POST method that will let you get parameters that were submitted using POST.
One of the main differences with GET and POST is that you can bookmark a page that uses GET since the parameters are in the URL. For example, you could bookmark http://localhost/shirts4mike/search/index.php?s=blue
and that page would show you all the blue shirts when you go to it from your bookmarks. You can't bookmark the results of a POST because the data exists only for that request. You've probably been on some web page and refreshed it and the browser said something like "are you sure you want to resubmit this form", right? That's because the form was submitted using a POST.
I hope that explains things a little better.