Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial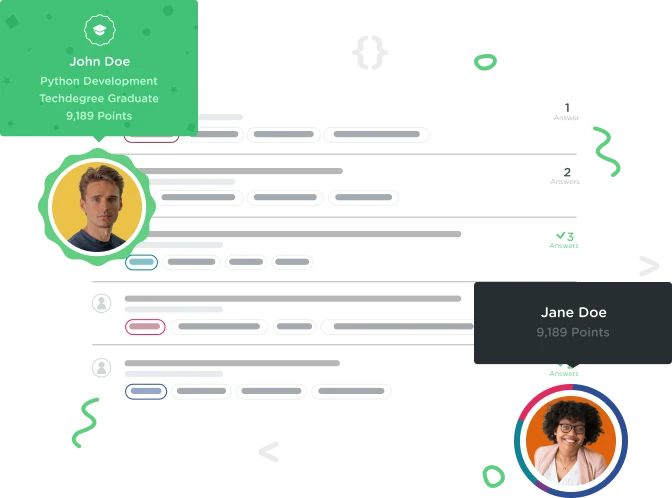

Liz Karaffa
17,576 PointsExtra Credit: Adding algorithm to search SKU as well as name.
In doing this challenge, I used the below code to make it work.
function get_products_search($s) {
$results = array();
$all = get_products_all();
foreach ($all as $product) {
if(stripos($product["name"],$s) !== false) {
$results[] = $product;
}
}
foreach ($all as $product) {
if(stripos($product["sku"],$s) !== false) {
$results[] = $product;
}
}
return $results;
}
But I was wondering if this is the best way or if I can check both the SKU and name in one foreach loop. I tried using both AND and OR but that broke the search feature.
7 Answers
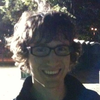
Ben Rubin
Courses Plus Student 14,658 PointsOops, I was missing one of the stripos
. Try this.
function get_products_search($s) { $results = array(); $all = get_products_all();
foreach ($all as $product) {
if(stripos($product["name"], $s) !== false OR stripos($product["sku"], $s) !== false) {
$results[] = $product;
}
}
return $results;
}
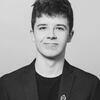
John MacDonald
8,593 PointsHey Guys!
I thought I might add an improvement in this old thread
I did try some of this code once by for instance if I searched up the number 1 it would return every product with the number 1 in it.
I reverted to checking if the SKU was equal to my search term and if it was appended it to the array.
Now if I search up a SKU like the number 1 it will return nothing but if I search a SKU of 102 it returns the product.
Check the code here:
function get_products_search($s){
$results = array();
$all = get_products_all();
foreach($all as $product){
if (stripos($product["name"], $s) !== false || $product["sku"] == $s){
$results[] = $product;
}
}
return $results;
}
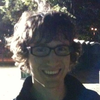
Ben Rubin
Courses Plus Student 14,658 PointsIt should work with a single foreach
loop if you combine the conditions with an OR, and you're correct in thinking that's a better way of doing it. What was it doing when you had both conditions inside a single loop?

Liz Karaffa
17,576 PointsIt acted as if there were no search results available. This is the condition with OR I tried but it didn't work.
function get_products_search($s) {
$results = array();
$all = get_products_all();
foreach ($all as $product) {
if(stripos($product["name"] OR $product["sku"],$s) !== false) {
$results[] = $product;
}
}
return $results;
}
What should I be doing differently?
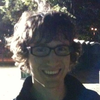
Ben Rubin
Courses Plus Student 14,658 PointsYou need a !== false
after stripos($product["name"]
. So it should be
function get_products_search($s) {
$results = array();
$all = get_products_all();
foreach ($all as $product) {
if(stripos($product["name"] !== false OR $product["sku"],$s) !== false) {
$results[] = $product;
}
}
return $results;
}
You have to put the value you are comparing to after each comparison. It has to be something like
if (first_value !== false AND second_value !== false AND third_value !== false)
You can't compare all of your conditions to a single value. Don't do this:
if (first_value AND second_value AND third_value !== false)

Liz Karaffa
17,576 PointsI also tried this, and while it didn't break the page, it showed no results.
function get_products_search($s) {
$results = array();
$all = get_products_all();
foreach ($all as $product) {
if(stripos($product["name"] OR ["sku"],$s) !== false) {
$results[] = $product;
}
}
return $results;
}
This broke the page.
function get_products_search($s) {
$results = array();
$all = get_products_all();
foreach ($all as $product) {
if(stripos($product["name" OR "sku"],$s) !== false) {
$results[] = $product;
}
}
return $results;
}

Liz Karaffa
17,576 PointsGreat! Thanks so much! I didn't know that syntax! Alas, when I copied and pasted exacted what you provided I still have the same result. The page isn't broken, but it acts as if there are no matching results.

Liz Karaffa
17,576 PointsAhh I see, so it's essentially the entire statement twice with the OR btwn a complete idea. Makes sense. Put this in my code and it worked. Thanks again for your help! Definitely learned a valuable tip!