Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial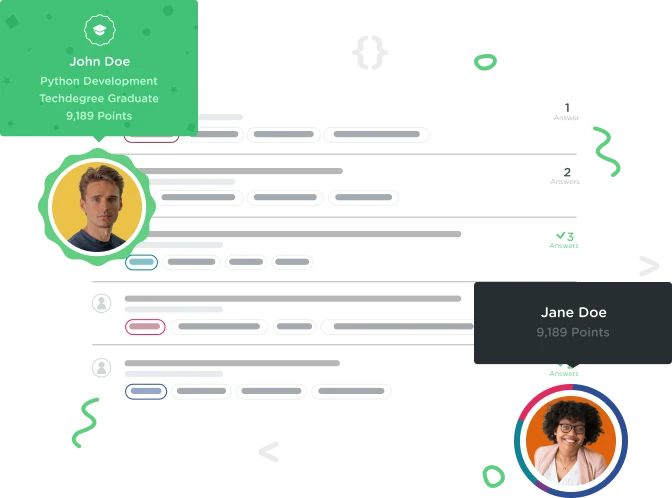
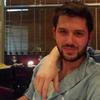
Nir Frank
3,578 PointsExtra Credit Assignment
Complete beginner here, only learned HTML and CSS beforehand :)
Anyway I'm at the extra credit assignment under "Introduction to Programming" which is as follows:
"Create a function that takes 3 arguments, a, b, and c. If a is an even number have the function return the string "even". Otherwise have the function return the string "odd""
This is my solution. I know it works, but I really want to get this stuff down right, and I was wondering if this is the best way to go about it:
var evenOdd = function (a, b, c) {
var evenNum = a % 2;
if (evenNum === 0) {
return "Even";
} else {
return "Odd";
}
}
Thanks!
3 Answers
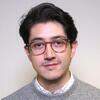
Jose Morales-Mendizabal
19,175 PointsGood Job using the modulus operator! Your function works well, and the only way it could be fixed is by shortening it by one line, as such:
var evenOdd = function (a, b, c) {
if ((a % 2) === 0) {
return "Even";
} else {
return "Odd";
}
};
Same thing, but in the code above you're not storing the parameter in a variable. I'd say in this function it is not necessary to add an extra variable, but my change change is just nit picky. Also you're missing a semi-colon after the last curly brace, just to be syntactically correct ;)

Mark Burns
1,922 Pointsthe change would though allocate a "var evenNum" and take up more memory for little gain even in readability
It is possible that the code would optimize by storing the value of a in the register rather than make memory moves before comparing as well
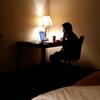
Michael Bianchi
2,488 PointsThis was my solution for the very first extra credit. I didn't consider using a function for it and I will try that too.
for (var i = 1; i < 101; i++){
if((i % 3 == 0)){
console.log("fizz")}
else if(i % 5 == 0){
console.log("buzz");}
else {
console.log(i);}
}
Nir Frank
3,578 PointsNir Frank
3,578 PointsThanks Jose! I'd think that once you start writing some actual programs (I'm aiming for web app development with AngularJS) this kind of stuff matters because it affects performance (also, would this affect a minimizer if it were to be used?). Am I right?
(Also also, why yes! I have indeed been practicing my Google-Fu ;P )
Jose Morales-Mendizabal
19,175 PointsJose Morales-Mendizabal
19,175 PointsNir Frank , In terms of performance, getting rid of one line of code is very negligible. The common golden rule is to keep code efficient, DRY, and as short as possible AS LONG as it is ALSO readable, and maintainable. You don't want to refactor some code so much that you forget what the code does. So try to use comments to document code that is not as obvious.
And this wouldn't affect a minimizer. It would still work.