Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial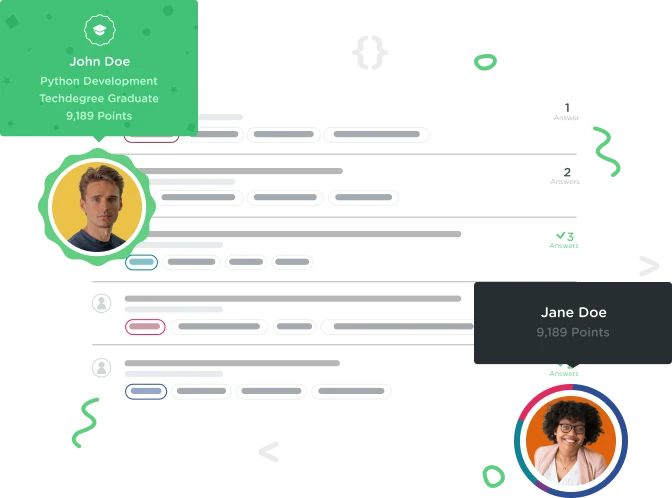
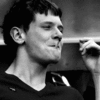
Derek Dawes
4,944 PointsExtra Credit Fizz Buzz (is this correct)?
Just would like to know if I used the correct syntax or if there is a better way to do this. It tested out on the console. Thank you
var x = 1
var divideByThree = 0
var divideByFive = 0
console.log("Start of loop");
while (x < 101) {
divideByThree = x % 3;
divideByFive = x % 5;
if(divideByThree === 0) {
console.log("fizz");
}
if (divideByFive === 0) {
console.log("buzz");
}
if (divideByThree === 0 && divideByFive === 0) {
console.log("fizzbuzz");
} else {
console.log(x);
}
x += 1
}
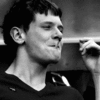
Derek Dawes
4,944 PointsDang space bars. Man; thanks guys for all your help. It worked when I used else if rather than elseif. I remember using cout a while back I think it was on C or something. Anyhow thank you for all your help. Sean, Ill study your blocks tomorrow. Again thanks!
2 Answers
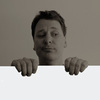
Sean T. Unwin
28,690 PointsGood effort, Derek Dawes . :)
Going the else if
route as suggested is a good way to go, just be sure they are separate words as there is no elseif
in JavaScript.
As Shawn Flanigan has also suggested, be sure to keep the most unique cases at the top of the conditional chain to ensure they will be considered.
I have included a few different ways to write the same thing below. The conditional logic is placed in a function called DivisibleBy3Or5
passing in a Number
as an argument. I have also created a function called cout
which takes a String
so it's quicker and easier to type out console.log
more than once:
Using if else
:
var x = 1;
function DivisibleBy3Or5(num) {
if (num % 3 === 0 && num % 5 === 0) {
return 'fizzbuzz';
} else if (num % 3 === 0) {
return 'fizz';
} else if (num % 5 === 0) {
return 'buzz';
} else {
return num.toString();
}
}
function cout(str) {
console.log(str);
}
cout('Start of loop');
while (x <= 100) {
cout(isDivisibleBy3Or5(x));
x += 1;
}
- For the rest of the examples I have only included the
DivisibleBy3Or5
function.
Using if
only for a little less screen space:
function DivisibleBy3Or5(num) {
if (num % 3 === 0 && num % 5 === 0) return 'fizzbuzz';
if (num % 3 === 0) return 'fizz';
if (num % 5 === 0) return 'buzz';
if (!num % 3 === 0 || !num % 5 === 0) return num.toString();
}
Same as the if else
example, but slightly less code using a ternary operator conditional statement:
function DivisibleBy3Or5(num) {
// This could, technically, be all on one line,
// but separated for readability
return (num % 3 === 0 && num % 5 === 0)
? 'fizzbuzz'
: (num % 3 === 0)
? 'fizz'
: (num % 5 === 0)
? 'buzz'
: num.toString();
}
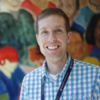
Brandon Barrette
20,485 PointsLooks pretty good. My only critique would be that with your conditional statements, you are going to check each if statement. I would think of a logical way of ordering these using if and elseif statements. That way, if it's divisible by one number, you don't need to check the others.
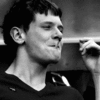
Derek Dawes
4,944 PointsThanks Brandon; I am familiar with elseif from Qbasic but Im using notepad++ and it doesnt seem to highlight that keyword. Ill give it a shot to see if it gives me an error.
Update
entered this and it gave me syntax error
var x = 1
var divideByThree = 0
var divideByFive = 0
console.log("Start of loop");
while (x < 101) {
divideByThree = x % 3;
divideByFive = x % 5;
if(divideByThree === 0) {
console.log("fizz");
} elseif (divideByFive === 0) {
console.log("buzz");
} elseif (divideByThree === 0 && divideByFive === 0) {
console.log("fizzbuzz");
} else {
console.log(x);
}
x += 1
}
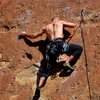
Shawn Flanigan
Courses Plus Student 15,815 PointsLooks like you're missing a semicolon at the end of your x += 1
statement and in all of your variable declarations up top. You may want to fix those. Hopefully this will fix your syntax error.
Also, you'll probably need to move the following conditional to the top of your logic:
(divideByThree === 0 && divideByFive === 0)
As it's written right now, a number like 15, which is divisible by both 5 and 3 (and should be a fizzbuzz) will pass the first conditional (divideByThree === 0
) and your script will output fizz and move on to the next number.
Hope this helps.
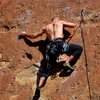
Shawn Flanigan
Courses Plus Student 15,815 PointsAhh...I didn't notice it before, but you need a space between else
and if
in JavaScript. Try that out and let us know how it goes.
Derek Dawes
4,944 PointsDerek Dawes
4,944 PointsThank you very much Shawn. I made the corrections you suggested. I think its an notepad++ issue because I am still getting the same error. I attached a screen shot link. Thank you for your help.
https://app.box.com/s/c996xa8fwg2a8fdq94y4tv2dlygeo2iu