Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial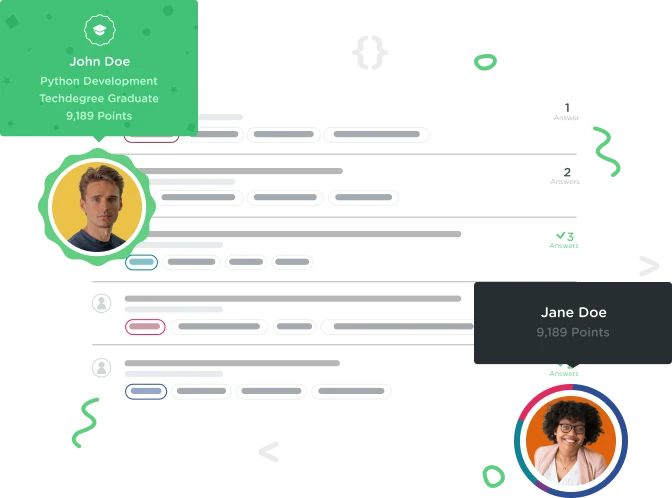
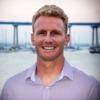
BRIAN WEBER
21,570 PointsExtra Credit Help Lists Redux (Move Items from One Position to Another)
Hello everyone. I am attempting the extra credit for the Lists Redux section under Python Collections. I have completed the CLEAR command part, but I am having trouble with moving items from one position to another. First off, when I prompt for user input for which item number the user wants to move I receive an error at idx_new. I am not sure how to format the input method to place an item at a certain index within a list to display. As for the "MOVE" code, I think it may be right, but I haven't got past the idx_new error. Below is the code I have (for some reason I cannot post the code to be formatted correctly like the other posts that I have seen):
Any help would be greatly appreciated!!!
Thanks,
Brian

Devin Bartley
2,800 PointsHi Brian,
I can at least help you at least with the formatting. if you scroll to the bottom of the comments there is a Markdown Cheatsheet that shows you how to do the markups to separate the code. You should add 3 back ticks at the top line and 3 back ticks on the bottom line. If you add "python" after the back ticks at the top line it will highlight correctly.
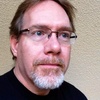
Chris Freeman
Treehouse Moderator 68,423 PointsThe type of error you received is important. Is it an IndexError
meaning the index used for the shopping_list
prompt is causing the error? Do you bounds check the old_index to be sure it is a valid value.
Along with fixing your formatting, please include the error thrown.
2 Answers
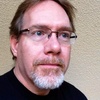
Chris Freeman
Treehouse Moderator 68,423 PointsFormatting your code:
shopping_list = []
def remove_item(idx):
index = idx - 1
item = shopping_list.pop(index)
print("Removed {}.".format(item))
def clear_list():
shopping_list.clear()
print("\nShopping list is cleared!")
def move_item(old_index,new_index):
item_to_move = shopping_list[old_index]
item = shopping_list.insert(new_index, item_to_move)
def show_help():
print("\nSeparate each item with a comma.")
print("Type DONE to quit, SHOW to see the current list, REMOVE to delete an item, MOVE an item and HELP to get this message.")
def show_list():
count = 1
for item in shopping_list:
print("{}: {}".format(count, item))
count += 1
print("Give me a list of things you want to shop for.")
show_help()
while True:
new_stuff = input("> ")
if new_stuff == "DONE":
print("\nHere's your list: ")
show_list()
break
elif new_stuff == "HELP":
show_help()
continue
elif new_stuff == "SHOW":
show_list()
continue
elif new_stuff == "REMOVE":
show_list()
idx = input("Which item? Tell me the number.")
remove_item(int(idx))
elif new_stuff == "CLEAR":
clear_list()
continue
elif new_stuff == "MOVE":
if len(shopping_list) == 0:
print("\nThere are no items in your shopping list.")
show_help()
else:
show_list()
idx_old = input("\nGive me the number of the item you want to move: ")
old_index = idx_old - 1
idx_new = input("\nWhere do you want to place the ", shopping_list[old_index], "?")
new_index = idx_new - 1
move_item(old_index, new_index)
else:
new_list = new_stuff.split(",")
index = input("Add this at a certain spot? Press enter for the end of the list, or give me a number, Currently {} items in the list.".format(len(shopping_list)))
if index:
try:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
except:
print("\nCan only accept a number. Currently there are {} items in the shopping list".format(len(shopping_list)))
else:
for item in new_list:
shopping_list.append(item.strip())
When running in a local file shop.py
, I get the error:
Give me the number of the item you want to move: 1
Traceback (most recent call last):
File "shop.py", line 56, in <module>
old_index = idx_old - 1
TypeError: unsupported operand type(s) for -: 'str' and 'int'
input()
returns a string. You need to wrap idx_old
and idx_new
in an int()
function:
old_index = int(idx_old) - 1
...
new_index = int(idx_new) - 1
Once this was fixed, I notice that your second input prompt threw an error. input()
takes a single argument. Using comma give it three. Change the commas to pluses +
, or better yet, use a formatted string:
idx_new = input("\nWhere do you want to place the {}?".format(shopping_list[old_index]))
One other error I noticed, after a MOVE, the item was in the new location, but also still in the old location.
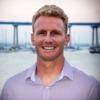
BRIAN WEBER
21,570 PointsDevin and Chris thank you for your help regarding formatting the code and fixing my code for the aforementioned errors. It seems to be working fine now.
shopping_list = []
def remove_item(idx):
index = idx - 1
item = shopping_list.pop(index)
print("Removed {}.".format(item))
def clear_list():
shopping_list.clear()
print("\nShopping list is cleared!")
def move_item(old_index,new_index):
item = shopping_list.insert(new_index, shopping_list.pop(old_index))
def show_help():
print("\nSeparate each item with a comma.")
print("Type DONE to quit, SHOW to see the current list, REMOVE to delete an item, MOVE an item and HELP to get this message.")
def show_list():
count = 1
for item in shopping_list:
print("{}: {}".format(count, item))
count += 1
print("Give me a list of things you want to shop for.")
show_help()
while True:
new_stuff = input("> ")
if new_stuff == "DONE":
print("\nHere's your list: ")
show_list()
break
elif new_stuff == "HELP":
show_help()
continue
elif new_stuff == "SHOW":
show_list()
continue
elif new_stuff == "REMOVE":
show_list()
idx = input("Which item? Tell me the number.")
remove_item(int(idx))
elif new_stuff == "CLEAR":
clear_list()
continue
elif new_stuff == "MOVE":
if len(shopping_list) == 0:
print("\nThere are no items in your shopping list.")
show_help()
else:
show_list()
idx_old = input("\nGive me the number of the item you want to move: ")
old_index = int(idx_old) - 1
idx_new = input("\nWhere do you want to place the " + shopping_list[old_index] + "?")
# or idx_new = input("\nWhere do you want to place the {}?".format(shopping_list[old_index]))
new_index = int(idx_new) - 1
move_item(old_index, new_index)
else:
new_list = new_stuff.split(",")
index = input("Add this at a certain spot? Press enter for the end of the list, or give me a number, Currently {} items in the list.".format(len(shopping_list)))
if index:
try:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
except:
print("\nCan only accept a number. Currently there are {} items in the shopping list".format(len(shopping_list)))
else:
for item in new_list:
shopping_list.append(item.strip())
To fix the problem where after a MOVE, the item was in the new location, but also still in the old location: I forgot to add the pop method for the item to insert. The correct code is below.
def move_item(old_index,new_index):
item = shopping_list.insert(new_index, shopping_list.pop(old_index))
Thanks again guys for your speedy responses!!
BRIAN WEBER
21,570 PointsBRIAN WEBER
21,570 PointsI went ahead and formatted the code here in my previous post.