Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial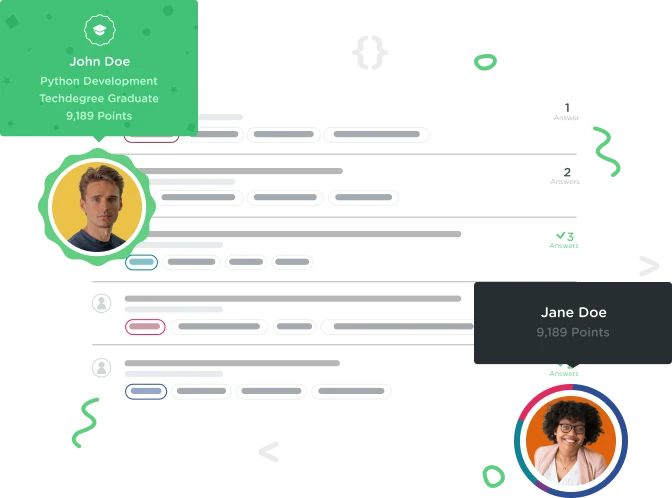
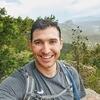
Justin Hein
14,811 PointsExtra Credit - Intro to Prog
This is the Extra Credit: Write a program that loops through the numbers 1 through 100. Each number should be printed to the console, using console.log(). However, if the number is a multiple of 3, don't print the number, instead print the word "fizz". If the number is a multiple of 5, print "buzz" instead of the number. If it is a multiple of 3 and a multiple of 5, print "fizzbuzz" instead of the number.
Hint. Use loops and if/else statments. In javascript the % is the modulo, or remainder operator. a % b evaluates to the remainder of a divided by b. 11 % 3 is equal to 2.
for (var i = 1; i <= 100; i + 1) {
if (i % 3 = 0) {
console.log("Fizz")
}
else if (i % 5 = 0) {
console.log("Buzz")
}
else if (i % 5 = 0, i % 3 = 0) {
console.log("fizzbuzz")
}
else {
console.log(i)
}
}
All I get from the browser is, '''Uncaught ReferenceError: Invalid left-hand side in assignment '''
What am I doing wrong? Or at least what is a better way to do this?
3 Answers

Dino Paลกkvan
Courses Plus Student 44,108 PointsFirst of all, your for
loop doesn't increase the value of i
. You have to assign the value of incremented i
to i
(i = i + 1
). You basically have an endless loop there.
Secondly, in your if
statements, you're using the assignment operator (=
) instead of using one of the equality operators (==
or ===
).
Also, you can't list additional conditions inside an if
statement with commas. You need to use logical operators, in this case the logical AND operator (&&
).
That's on the side of the syntax.
As far as the logic of your code goes, the check if a number is divisible both by 3
and 5
should be the first one. Otherwise you'll end up outputting fizz
for such numbers.
Also, a number that is divisible both by 3
and 5
is actually also divisible by 15
, so you can reduce that to only one check.
The end result:
for (var i = 1; i <= 100; i = i + 1) { // fixing the endless loop here
if (i % 15 === 0) { // first check if the number is divisible by 15 (both 3 and 5)
console.log("fizzbuzz");
}
else if (i % 3 === 0) { // use the equality (identity) operator
console.log("fizz");
}
else if (i % 5 === 0) { // use the equality (identity) operator
console.log("buzz");
}
else {
console.log(i);
}
}

Scott Fletcher
236 PointsYou've got a few problems.
First, to check for equality in your if
statements, you want to use the javascript logical operator ===
rather than the assignment operator =
.
Second, in your for
loop you need to actually increase the value of i
. You can do this with this assignment operator i += 1
.
Finally, you've got both a syntax error on your second else if
as well as a logic error in the placement of this condition. To fix the former, you'll want to use one of the javascript logical operators (I'm guessing either & or ||). To fix the latter, think about the order in which your if statement conditions are being asked and executed, and remember that if statements stop advancing after the first truthy expression is found (and executes the statement therein).
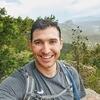
Justin Hein
14,811 PointsHey Scott, just wondering, could you explain how i += 1 works and the difference in &&, & and ||?
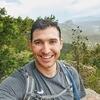
Justin Hein
14,811 PointsThanks guys, really appreciate it. Makes a lot of sense now. Just got onto JS yesterday, starting to learn the syntax just takes a bit of getting used to!