Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial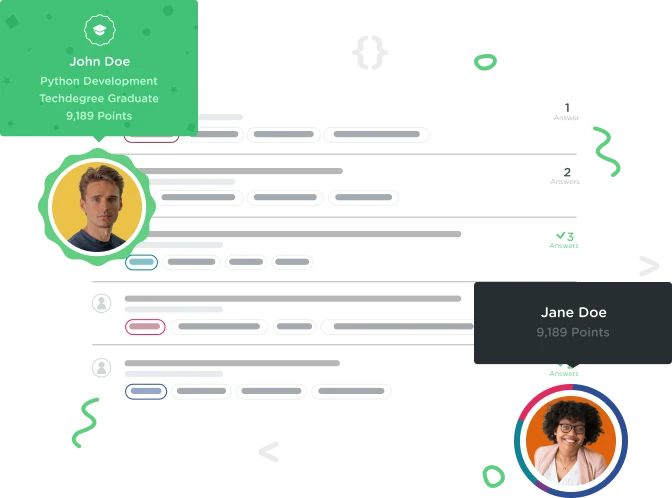
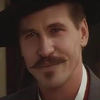
huckleberry
14,636 PointsExtra Credit - Loops: My Versions. Critique please! :)
Hello all,
In getting to the loops extra credit, I wasn't really sure how to go about it as it seemed like you would need to nest the statements and I did recall from years ago when I first attempted to dabble in a language that you could nest the statements so gave it the ol' college try and here's what I came up with.
Oh, and I also had to go looking up operators because at first I couldn't figure out how to get both the % 5 and % 3 things to combine. So one version looks a weeee bit different than the other lol. I know they didn't cover any of that stuff in the videos, but I assumed "Hey, it's extra credit and coding is a rather self driven and auto didactic pursuit so maybe their intention is to have us go out and look stuff up and use it."
Anyway, here you go.
with the weird operators & nested statements
===
for (var eCredit = 1; eCredit <= 100; eCredit = eCredit + 1) {
if (eCredit % 5 === 0 && eCredit % 3 === 0) {
console.log("fizzbuzz")
}
else if (eCredit % 5 === 0) {
console.log("buzz");
}
else if (eCredit % 3 === 0){
console.log("fizz");
}
else {
console.log(eCredit);
}
}
per the video
===
for (var eCredit = 100; eCredit >= 1; eCredit = eCredit - 1) {
if (eCredit % 3){
if (eCredit % 5){
console.log(eCredit);
}
else {
console.log("buzz");
}
}
else {
if(eCredit % 5){
console.log("fizz");
}
else{
console.log("fizbuzz");
}
}
}
They both returned the proper result, but I'm a newb and not 100% sure I did it the best way so please tell me how it could be done better/cleaner or whatever critiques you may have.
Thanks a bunch!
2 Answers
William Li
Courses Plus Student 26,868 PointsI think your solutions are alright, for this particular problem, there really isn't any better way to go about solving it.
One small change I suggest is that
for (var eCredit = 1; eCredit <= 100; eCredit++)
eCredit++
is basically a shorthand of eCredit = eCredit + 1
, people generally prefer eCredit++
cuz it's more concise.
If you ask me which of your solutions is better, I'd probably say the first one, simply because it lacks the nested if
in the 2nd solution, making it easier to read and understand.
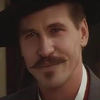
huckleberry
14,636 PointsSweet, I get it! Thanks a ton, William! Much appreciated :).
huckleberry
14,636 Pointshuckleberry
14,636 PointsThank you so much!
Just want to add that when I was doing the extra credit I had only done up to the loops video up until that point and he didn't mention the other operator until that vid. Although, he never mentions ++ but only the += operator.
So now my bit of code looks like so.
And I agree in your other remark, I definitely like the way the first chunk of code looks and flows much better than the 2nd one.
to
+=
, or++
In your experience, which is better/more common and, if you don't mind expanding upon 'why?' and what the inherent differences and uses are between their functionality.
Like, it seems
varName++
would indeed be better but at the same time I'm sure there are instances where using...Oh wait, it just hit me. the
++
is only to increment by one, right? Whereas+=
allows you to define what increment to increase by!So if you're doing a simple +1 increment which is just so common as to be given its own operator, just use the
varName++
format and the other one,varName += #
would be used for any other increments.Correct?
Thanks in advance!
William Li
Courses Plus Student 26,868 PointsWilliam Li
Courses Plus Student 26,868 Pointscorrect.
++
only increment the variable by 1, if you need to increment it by other values, you would have to+=
. You see, when writing javascript, it's very common to increment a variable by 1, especially when writing a for loop, common enough that the language designer gives you a++
shorthand for writing it.So to sum it all.