Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial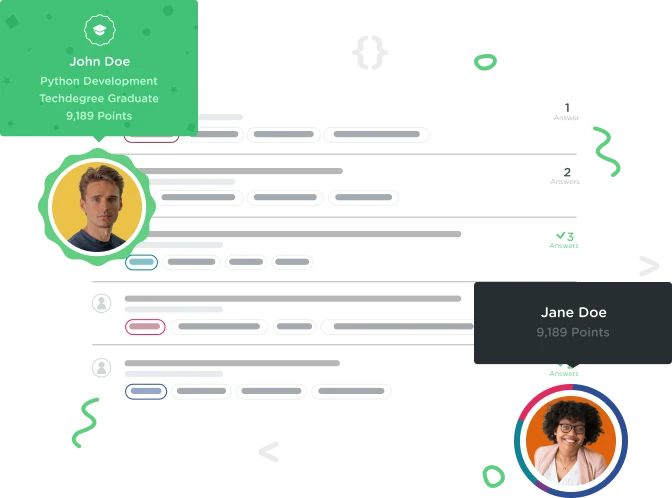
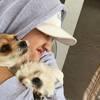
Carlos Marin
8,009 PointsExtra-credit?
I am working on a function to process the guess. Since I am being tracked by my points, it will be a small project I come work on a little day by day. I wanted to get some feedback on my current code. I am wondering if I should have chosen a try: statement? if so, why?
anyways, here is my function. challenge_word = (list('learner')) guess = (list('earn'))
def check_guess(guess):
guess_word_list = list(guess)
challenge_word_list = list(challenge_word)
match_found_string = ''
for index, letter in enumerate(guess_word_list):
print("checking {}, iteration#{}".format(letter, index))
for check in challenge_word_list:
if letter != check:
print("{} == {}? X".format(letter, check))
else:
print("{} == {}? MATCH".format(letter, check))
match_found_string += check
break #ends iteration for letter
if letter not in challenge_word:
print("This letter was not approved.")
print("(Iterations complete): approved letters = {}".format(match_found_string))
2 Answers
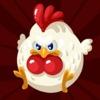
Binyamin Friedman
14,615 PointsI don't know what you mean by using a try statement but I do want to point out one flaw in your code:
Currently, eeeeeennnnnnnnnn as a guess would pass with the challenge word "learn." You could fix this by removing the letter from the challenge word list.
Here is fully working code:
# I removed all the print statements, as they are really only for debugging, and renamed variables to make more sense
challenge_word = "learner" # the list() call was unnecessary
guess = "eee" # the list() call was unnecessary
def check_guess(challenge_word, guess): # challenge_word is now being passed into the function
guess_list = list(guess)
challenge_word_list = list(challenge_word)
valid_letters = ""
for letter in guess_list:
for compared_letter in challenge_word_list:
if letter == compared_letter:
valid_letters += compared_letter
challenge_word_list.remove(letter) # remove letter from the challenge_word_list so that it can't be used again
break
return valid_letters == guess # return true if all the letters are valid
valid_guess = check_guess(challenge_word, guess)
print("Guess is valid" if valid_guess else "Guess is invalid")
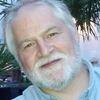
Jeff Muday
Treehouse Moderator 28,716 PointsNice work-- looks like you are getting some good Python experience under your belt.
3 comments:
I like your mastery of the enumerate() function. In my opinion, using the enumeration is cleaner/clearer than using a separate index counter for iteration.
Try/Except blocks are good to know and use. Though they are a little slower than a normal if/then/else block. So I tend to avoid them (if possible) in performance-critical blocks of code
Is the indentation correct on the last if/then? Shouldn't it be nested under the "for loop"?