Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial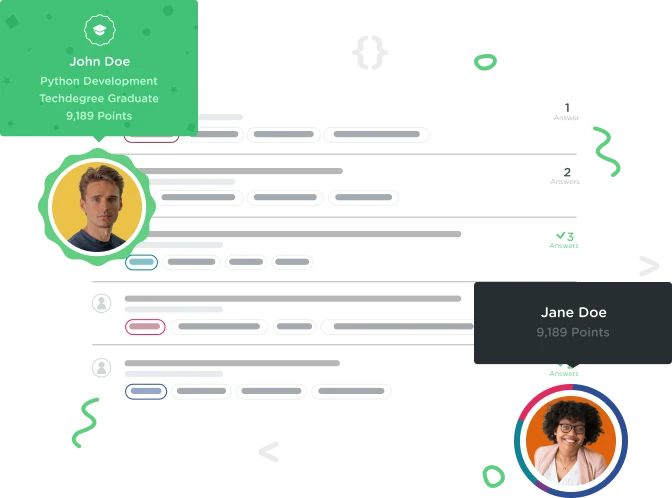

Premkumar Chandrashegaran
1,333 Pointsfacts.length()
Does the method length() for object facts starts from 1 to "length of array" or 0 to 'length of array - 1"?
I am confused about the facts[randomNumber] array. Any help will be much appreciated.
3 Answers
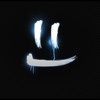
Grigorij Schleifer
10,365 PointsThis is your code:
String[] facts = {"one", "two", "three", "four"};
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt((facts.length));
// the number generator will give you an int between 0 and 4
// thats why you need to tell the generator how many items are inside of the array
But what if the generator gives you number 4 ... this will give you an error. There is no item on index 4 inside of the array. On index 3 is the String "four". So you can modify your code like this:
int randomNumber = randomGenerator.nextInt((facts.length) - 1);
// this will give you a number between 0 - 3
So you can extract a random String from your array using this line:
System.out.println(facts[randomNumber]);
Make sense?

Premkumar Chandrashegaran
1,333 PointsMakes much more sense now when I make that (facts.length) - 1.
Thank you very much!
By the way, Happy New Year!

Trainer Workout
22,341 PointsHope this helps
String[] facts = {"one", "two", "three", "four"};
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt((facts.length));
//facts.length returns 4 because there are 4 elements in the array.
//the randomGenerator will give you an int between 0 and 3 (4 possible values)
//These numbers correspond to each element in the facts array
//"one" = 0
//"two" = 1
//""three"" = 2 etc.
// Displays the fact on the screen.
factTextView.setText(facts[randomNumber]);
// The array index values match exactly the random number values
// For example, if randomGenerator returns 3 (the maximum possible value),
// then facts[3] is selected, which corresponds to "four"
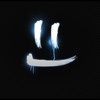
Grigorij Schleifer
10,365 PointsHi Trainer,
thank you for the clarification :) You are totaly right !
this line:
int randomNumber = randomGenerator.nextInt(facts.length);
or this line:
int randomNumber = randomGenerator.nextInt(4);
They both return a number between 0 and 3 !!! I said that they return a number between 0 and 4, so 4 would be also possible and this is not correct. I hope that my explanation, despite of my error, could help understand the logic, Premkumar !
Sorry for the confusion and thanks again to Trainer !
Thumbs up

Premkumar Chandrashegaran
1,333 PointsHi Trainer,
Thank you, now I get it, even though the numbers generated by the random code is from 0-3 and facts.length is 4, but they both represent the same amount of number. So that explains a lot!
Thank you again. Appreciate that.
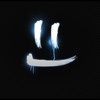
Grigorij Schleifer
10,365 PointsHi Premkumar,
the length method gives you the number of items of an array.
If you have an array like this:
String[] stringArray = {"itemOne", "itenTwo", "itemThree"};
stringArray.length would give you 3. Because there are three elements in the array.
Does it make sense?

Premkumar Chandrashegaran
1,333 PointsHi Grigorij,
Thanks. Now that makes sense. But now if we look at my code:
String[] facts = {"one", "two", "three", "four"}; Random randomGenerator = new Random(); int randomNumber = randomGenerator.nextInt(facts.length); facts[randomNumber];
This facts.length should give me values of 1,2,3,4 where as the array index points to 0,1,2,3. Will that give a bug?
Am I correct?
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsYou are welcome !!!!
See you in the forum ...
Take a look at the java course track here at treehouse ... I am sure you will find a lot cool stuff there ... and Craig is awesome :)