Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial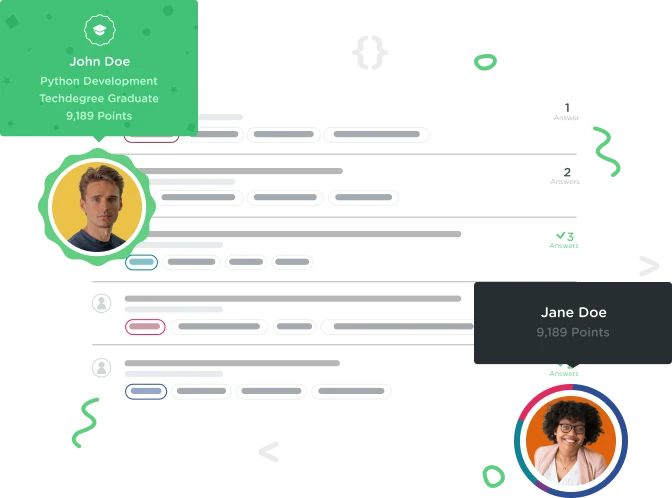

khoreysmith
3,524 Points"factTextView" and "showFactButton" errors
ISSUE:
For some reason I get an error saying "cannot find symbol variable factTextView" and "cannot find symbol variable showFactButton"
I'm not exactly where to start with fixing this problem. I've tried a few quick fixes by pressing alt+Enter. I've even tried to but that doesn't seem to work. PLEASE HELP!
Here is my source code from the funFactActivity.java file:
import android.app.Activity; import android.content.DialogInterface; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.TextView;
import static khoreysmith.funfacts.R.id; import static khoreysmith.funfacts.R.id.*;
public class FunFactActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_fact);
//Declare our view variables and assign the views from the layout file
final TextView factLabel = (TextView)findViewById(factTextView);
Button showFactButton = (Button) findViewById(id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
//The button was clicked, so update the fact label with a new fact
String fact = "Ostriches can run faster than horses";
factLabel.setText(fact);
}
};
showFactButton.setOnClickListener(listener);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.fun_fact, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
1 Answer

Aaron Arkie
5,345 PointsHello your code is missing some of the components it needs to find the id of the button and text view. we will focus on these two following lines.
final TextView factLabel = (TextView)findViewById(factTextView);
Button showFactButton = (Button) findViewById(id.showFactButton);
For the most part everything is correct including the casting, however look at the far right parameter. findViewById() expects you to insert an integer generated by the R class. Since you assigned an id to your button and textView it will now be stored in an identifiable value as an Integer. We want to use this integer id to be able to use it and assign it to the Text View. Furthermore think of the periods " . " as a way to get to something.
So what we are trying to say is I will use the R class . to get to the id variable . that represents my textView.
Okay now look at the next line! I hope this clears everything up let me know if you need any additional information.
final TextView factLabel = (TextView) findViewById(R.id.factLabel);
Button showFactButton = (Button) findViewById(R.id.showFactButton);