Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial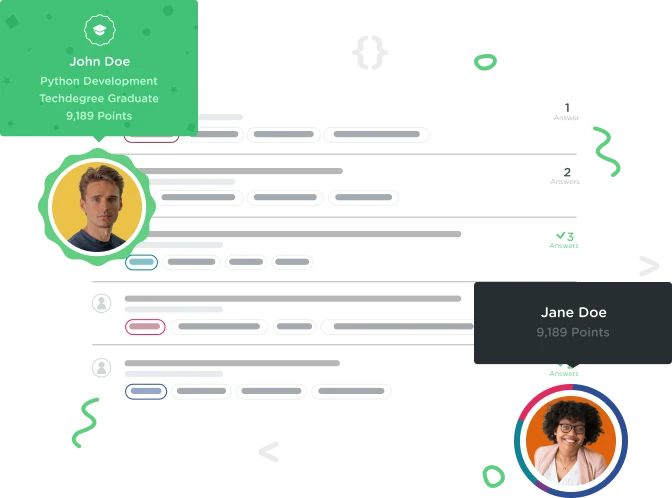

Benjamin Bell
4,364 PointsFading an image in & out
Hi guys,
instead of having an alertview display "liked!" when an image is tapped, I wanted an image to fade in and out like the actual instagram app. Here is the code I came up with:
- (void)like {
if (self.likeImage == nil) {
self.likeImage = [[UIImageView alloc] init];
self.likeImage.image = [UIImage imageNamed:@"like_icon"];
CGRect screen = [[UIScreen mainScreen] bounds];
[self.likeImage setFrame:CGRectMake((screen.size.width / 2.0) - 25, (screen.size.height / 2.0) - 22.5 - 66, 50, 45)];
}
if (![self.likeImage isDescendantOfView:self.superview]){
self.likeImage.alpha = 0;
[self.superview addSubview:self.likeImage];
}
[UIView animateWithDuration:1.0f animations:^{
self.likeImage.alpha = 1.0f;
}];
dispatch_after(dispatch_time(DISPATCH_TIME_NOW, (int64_t)(1.0 * NSEC_PER_SEC)), dispatch_get_main_queue(), ^{
[UIView animateWithDuration:1.0 animations:^{
self.likeImage.alpha = 0;
}];
});
}
I have a problem with this code, if I scroll down and tap on a photo the like does not appear (it does but out of my view). How can I make this responsive to me scrolling down? It also has to add he subview again every time I scroll.
6 Answers

Thomas Nilsen
14,957 Pointsok got it.
The code now looks like this:
- (void)showLikeCompletion {
if (self.likeImage == nil) {
self.likeImage = [[UIImageView alloc] init];
self.likeImage.image = [UIImage imageNamed:@"Treehouse"];
CGRect screen = [[UIScreen mainScreen] bounds];
[self.likeImage setFrame:CGRectMake((screen.size.width / 2.0) - 25,
(screen.size.height / 2.0) - 22.5,
50,
45)];
NSLog(@"%.0f", [[UIScreen mainScreen] bounds].size.width);
NSLog(@"%.0f", [[UIScreen mainScreen] bounds].size.height);
}
if (![self.likeImage isDescendantOfView:self.superview]){
NSLog(@"Subview added");
self.likeImage.alpha = 0;
[self.superview.superview addSubview:self.likeImage];
}
[UIView animateWithDuration:1.0 animations:^{
self.likeImage.alpha = 1;
}];
dispatch_after(dispatch_time(DISPATCH_TIME_NOW, (int64_t)(5 * NSEC_PER_SEC)), dispatch_get_main_queue(), ^{
[UIView animateWithDuration:1.0 animations:^{
self.likeImage.alpha = 0;
}];
});
}
the key line is this:
[self.superview.superview addSubview:self.likeImage];
Because when you're talking about the cells superview you're actually referring to the collection view. You need to address the collection view's superview to always get it centered :) (It's been a while since I did IOS related stuff).
Hope this was helpful!

Thomas Nilsen
14,957 PointsInstead of using bounds like this:
CGRect screen = [[UIScreen mainScreen] bounds];
do this:
CGRect screen = [[UIScreen mainScreen] frame];
If that's not it - you can share the project through dropbox or something so I can have a look.
Also, this has nothing to do with you problem, but write it like this:
[UIView animateWithDuration:1.0f animations:^{
self.likeImage.alpha = 1.0f;
} completion:^(BOOL finished) {
self.likeImage.alpha = 0;
}];
and NOT like this:
[UIView animateWithDuration:1.0f animations:^{
self.likeImage.alpha = 1.0f;
}];
dispatch_after(dispatch_time(DISPATCH_TIME_NOW, (int64_t)(1.0 * NSEC_PER_SEC)), dispatch_get_main_queue(), ^{
[UIView animateWithDuration:1.0 animations:^{
self.likeImage.alpha = 0;
}];
});

Benjamin Bell
4,364 PointsHi there,
UIScreen does not have the frame property it has applicationFrame instead. And unfortunately that gives me exactly the same values as bounds property.
Also I could use the completion block but id still have to do the following to include the fade out (your method makes the likeimage disappear instantaneously.
[UIView animateWithDuration:1.0f animations:^{
self.likeImage.alpha = 1.0f;
} completion:^(BOOL finished) {
[UIView animateWithDuration:1.0 animations:^{
self.likeImage.alpha = 0;
}
}];
Thanks for taking the time to look at this.

Thomas Nilsen
14,957 PointsNo problem, but would you mind sharing the project over dropbox or something. That way it would be a lot easier for me to find the mistake. Unless you've already figured it out :)

Benjamin Bell
4,364 PointsSure give me your dropbox email address and ill send you the project

Thomas Nilsen
14,957 Points..------...

Benjamin Bell
4,364 PointsDid you get the files?

Thomas Nilsen
14,957 PointsI did. I'll have a look very soon :)

Thomas Nilsen
14,957 PointsAwesome! :)
As for your question, If you write this:
NSLog(@"%@", self.superview.superclass);
It gives you UIScrollView
NSLog(@"%@", self.superview.superview.superclass);
gives you UIView, which is the top most class.

Benjamin Bell
4,364 PointsThanks again Thomas!
Benjamin Bell
4,364 PointsBenjamin Bell
4,364 PointsYou absolute hero!!
The superview of the collectionView is what? scrollView?