Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial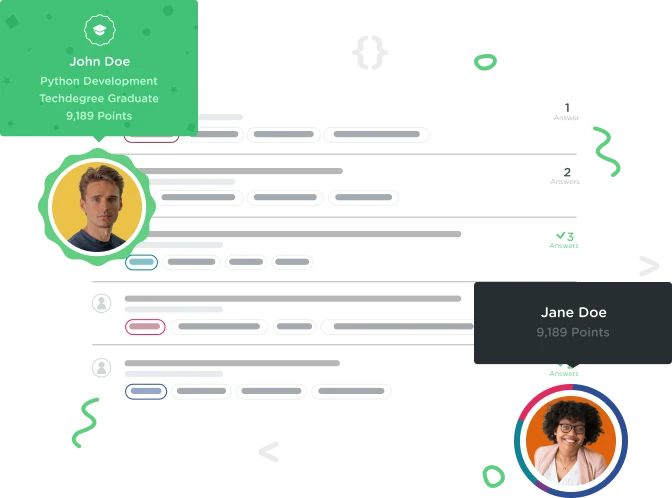

Patrick Duhamel
5,786 PointsFailable initializer task is missing something, but Playground or Preview mode doesn't give me a hint on the issue...
struct Book { let title: String let author: String let price: String? let pubDate: String?
init?(dict: [String: String]) {
if let title = dict["title"],
let author = dict["author"],
let price = dict["price"],
let pubDate = dict["pubDate"] {
self.author = author
self.title = title
self.price = price
self.pubDate = pubDate
} else {
return nil
}
}
}
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dict: [String: String]) {
if let title = dict["title"],
let author = dict["author"],
let price = dict["price"],
let pubDate = dict["pubDate"] {
self.author = author
self.title = title
self.price = price
self.pubDate = pubDate
} else {
return nil
}
}
}
1 Answer

Joshua Hawthorne
18,523 PointsNotice that "price" and "pubDate" in the Book struct are actually optionals. These values are allowed to be nil. In other words, price and pubDate can both be nil, and as long as title and author are valid we can create the instance of the struct. Your code should like like this (note that I am using guard statements, not if-let):
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dict: [String : String]) {
guard let title = dict["title"] else { return nil }
guard let author = dict["author"] else { return nil }
if let p = dict["price"] {
self.price = p
} else {
self.price = nil
}
if let pubDate = dict["pubDate"] {
self.pubDate = pubDate
} else {
self.pubDate = nil
}
self.title = title
self.author = author
}
}