Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial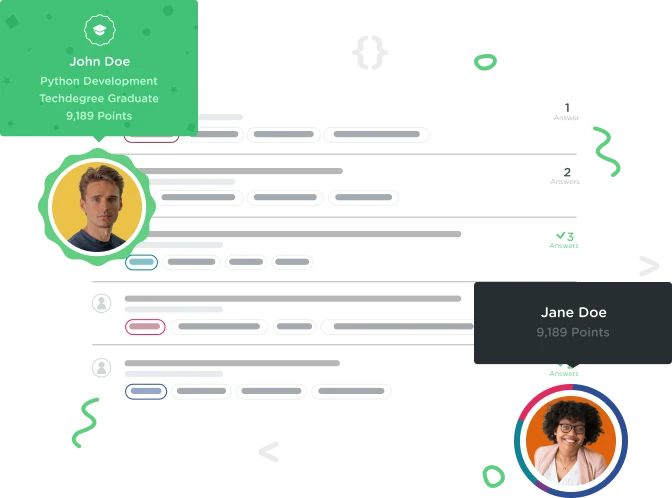

Y B
14,136 PointsFailing POSIX timestamp challenge
I can't get past this challenge. I'm assuming I'm doing the conversion of POSIX to datetime correctly? (unsure as we haven't done timezones at this point).
I've also had to assume the incoming arguments are in the past i.e. older than now - will this make it fail, or is it fine?
# If you need help, look up datetime.datetime.fromtimestamp()
# Also, remember that you *will not* know how many timestamps
# are coming in.
def timestamp_oldest(*args):
oldest = datetime.datetime.now()
for arg in args:
if oldest - datetime.datetime.fromtimestamp(arg) > 0:
oldest = datetime.datetime.fromtimestamp(arg)
return oldest
7 Answers
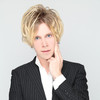
Mikael Enarsson
7,056 PointsFirst of all, you need to import datetime
Secondly, you can't compare a timedelta, which is you get with:
oldest - datetime.datetime.fromtimestamp(arg)
with an int.
You can however compare a datetime to a datetime:
oldest > datetime.datetime.fromtimestamp(arg)

Y B
14,136 Pointsthanks, that did it, good to know I wasn't ridicously far away!
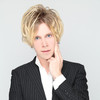
Mikael Enarsson
7,056 PointsIndeed you weren't! A note though, error messages are your friends. Try to understand them, they are really helpful!

Y B
14,136 PointsThanks, I do try to use them , a lot of the time though it just says try again! - which isn't too helpful
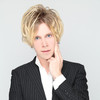
Mikael Enarsson
7,056 PointsIndeed, although that's mostly for semantic errors, which are a huge pain in the ass ><
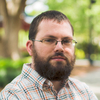
Kenneth Love
Treehouse Guest TeacherPOSIX timestamps are just floats. Floats can be compared to each other or, in a list, sorted.
There's your big hint for the day. :)

Y B
14,136 PointsThanks that leads me on to a side question to understand the unpacking better. Are the *args effectively passed in as a list so that one can do args.sort() . Or would a list have to be created from the args i.e. list(args).sort()?
Are the oldest Posix timestamps the smallest floats?
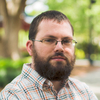
Kenneth Love
Treehouse Guest Teacher*args
is always a tuple, not a list, so it'll have to be converted to a list. Conversely, **kwargs
is always a dict.
And, yes, POSIX timestamps tick up one whole number per second, so older ones are smaller.
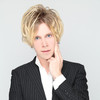
Mikael Enarsson
7,056 PointsSo, like this?
def timestamp_oldest(*args):
args_list = list(args)
args_list.sort()
return datetime.datetime.fromtimestamp(args_list[0])
A note, it seems that you get the correct result even without sorting the list, which means that in this list the item at index 0 was the correct answer from the beginning. That may be something you want to do something about?
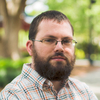
Kenneth Love
Treehouse Guest TeacherThe timestamps are randomized each time, but I've added in a check to make sure that the first one isn't the oldest before they're sent to your code.
And, yes, that should be a good solution.

Y B
14,136 Pointsok they are a tuple, that makes more sense with the arguments to a function being comma separated.