Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial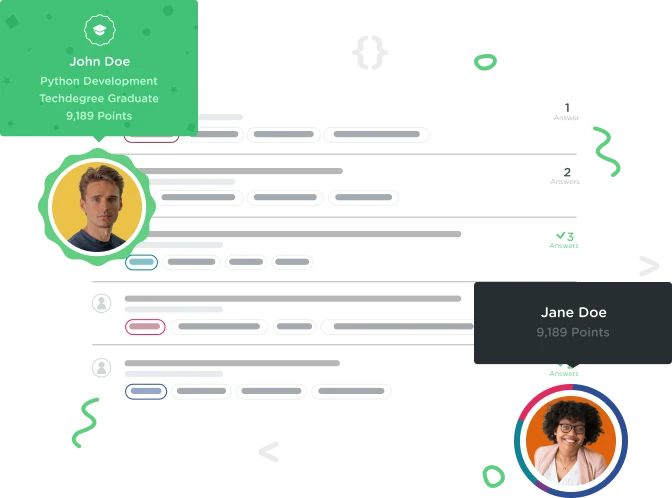

Thomas Weldon
2,718 PointsFails in Treehouse but runs in IntelliJ
The issue seems to be with the IOException requirement of the BufferedReader. Compiles and runs in IntelliJ but not in the treehouse console.
package com.teamtreehouse;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) throws IOException {
// write your code here
Prompter prompter = new Prompter();
BufferedReader storyReader = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Please provide a short story with a few words marked with __ around the word type ... like __noun__:");
String story = storyReader.readLine();
// String story = "Thanks __name__ for helping me out. You are really a __adjective__ __noun__ and I owe you a __noun__.";
Template tmpl = new Template(story);
prompter.run(tmpl);
// List<String> fakeResults = Arrays.asList("friend", "talented", "java programmer", "high five");
/*
String results = tmpl.render(fakeResults);
System.out.printf("Your TreeStory:%n%n%s", results);
*/
}
}
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
String templateResults = tmpl.render(results);
System.out.printf("Your TreeStory:%n%n%s", templateResults);
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) throws IOException{
System.out.print("Please provide a " + phrase + ": ");
String userPhrase = mReader.readLine();
String vPhrase = null;
if(mCensoredWords.contains(userPhrase)){
promptForWord(phrase);
} else {
vPhrase = userPhrase;
}
return vPhrase;
}
}
# This is essentially what I am testing
1. The user is prompted for a new string template (the one with the double underscores in it).
a. The prompter class has a new method that prompts for the story template, and that method is called.
2. The user is then prompted for each word that has been double underscored.
a. The answer is checked to see if it is contained in the censored words.
User is continually prompted until they enter a valid word
3. The user is presented with the completed story