Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial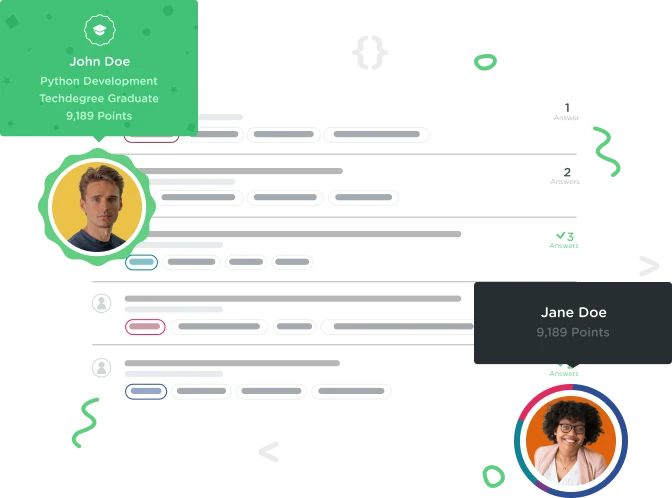
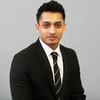
Mahmud Hussain
1,287 PointsFailure/Error: expect(page).to have_content("error")
require 'spec_helper'
describe "Creating todo lists" do
def create_todo_list(options={})
options[:title] ||= "My todo list"
options[:description] ||= "This is my todo list."
visit "/todo_lists"
click_link "New Todo list"
expect(page).to have_content("New todo_list")
fill_in "Title", with: options[:title]
fill_in "Description", with: options[:description]
click_button "Create Todo list"
end
it "redirects to the todo list index page on success" do
create_todo_list
expect(page).to have_content("My todo list")
end
it "displays an error when the todo list has no title" do
expect(TodoList.count).to eq(0)
create_todo_list title: ""
expect(page).to have_content("error")
expect(TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("This is what I'm doing today.")
end
it "displays an error when the todo list has a title less than 3 characters" do
expect(TodoList.count).to eq(0)
create_todo_list title: "Hi"
expect(page).to have_content("error")
expect(TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("This is what I'm doing today.")
end
it "displays an error when the todo list has no description" do
expect(TodoList.count).to eq(0)
create_todo_list title: "Grocery list", description: ""
expect(page).to have_content("error")
expect(TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("Grocery list")
end
it "displays an error when the todo list has no description" do
expect(TodoList.count).to eq(0)
create_todo_list title: "Grocery list", description: "Food"
expect(page).to have_content("error")
expect(TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("Grocery list")
end
end
this is my code but i still get...
Failures:
1) Creating todo lists displays an error when the todo list has no description
Failure/Error: expect(page).to have_content("error")
expected to find text "error" in "Todo list was successfully created. Title: Grocery list Description: Food Edit | Back"
# ./spec/features/todo_lists/create_spec.rb:63:in `block (2 levels) in <top (required)>'
Finished in 0.25165 seconds
5 examples, 1 failure
Failed examples:
rspec ./spec/features/todo_lists/create_spec.rb:58 # Creating todo lists displays an error when the todo list has no description
Randomized with seed 9141
2 Answers
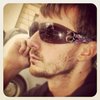
Adam Sackfield
Courses Plus Student 19,663 PointsFrom looking at your test-spec, I can see that the final 2 tests have the same content? I would delete the final test as it says testing no description but you have a description. See below an excerpt of your code.
it "displays an error when the todo list has no description" do
expect(TodoList.count).to eq(0)
create_todo_list title: "Grocery list", description: ""
expect(page).to have_content("error")
expect(TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("Grocery list")
end
it "displays an error when the todo list has no description" do
expect(TodoList.count).to eq(0)
create_todo_list title: "Grocery list", description: "Food"
expect(page).to have_content("error")
expect(TodoList.count).to eq(0)
visit "/todo_lists"
expect(page).to_not have_content("Grocery list")
end
See they are both the same test.
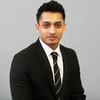
Mahmud Hussain
1,287 Pointsi go through the videos and then download the project files. removing it solved the problem, thanks a bunch!
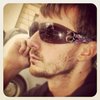
Adam Sackfield
Courses Plus Student 19,663 PointsYour welcome and anytime! Good luck for the rest of the course :)
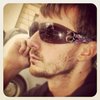
Adam Sackfield
Courses Plus Student 19,663 PointsAre you sure they don't fix it later in the video. As with TDD(Test Driven Development) you first write a failing test then add the code to make it pass. So if you carry on with the video it will add the code shortly
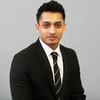
Mahmud Hussain
1,287 Pointsin the video it shows 0 failures but i get 1
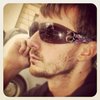
Adam Sackfield
Courses Plus Student 19,663 PointsOk give me a minute I will have a look now
Mahmud Hussain
1,287 PointsMahmud Hussain
1,287 Pointsi copy the tutorial but these types of errors are so frustrating, which holds me back from learning Rails :(