Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial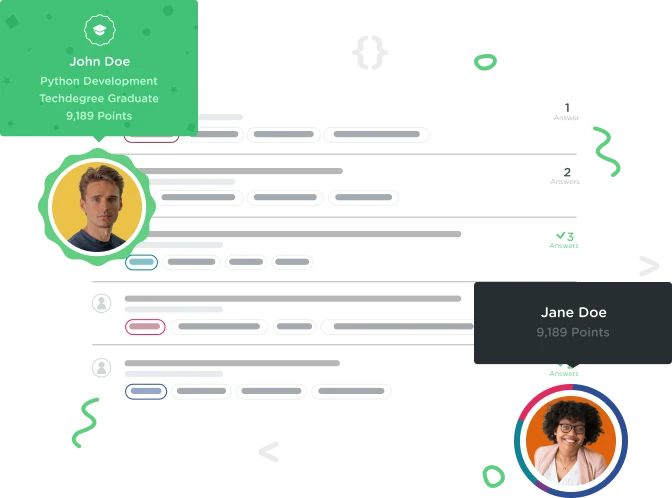

Branden Hunt
2,018 PointsFatal error: Class 'Slim\Slim' not found in C:\...\index.php on line 15
I had a question, but then I answered it, and so I thought it might help someone who may incur the same issue in the future.
I tried creating the $app object just as Hampton does in the video, and I kept getting the error described above. I was able to resolve it once I realized that Slim had transitioned to 3.X since this video was made (Hampton uses a 2.6.X iteration of Slim). According to the readme file that is included with Slim the class is now Slim\App not Slim\Slim. When I used $app = new \Slim\App(); the error was resolved. Also, the echo statement didn't work the same, but a function that does the same thing is also included in the same readme file. I found the file in the vendor/slim/slim folder.
4 Answers

Graeme Campbell
15,594 PointsOk, Slim/Views is also not compatible with Slim 3.0, so to get Slim 3.0 working with twig template files you need to do the following.
From composer:
require Slim/Slim
require Slim/twig-view
if you already added slim/views
remove Slim/Views
Then in the php, you need to use the following code snippets to make your twig files run
// Initalise use of templates
// Ensure debugging
$configuration = [
'settings' => [
'displayErrorDetails' => true,
],
];
// Create container
$container = new \Slim\Container($configuration);
// Register component on container
$container['view'] = function ($c)
{
$view = new \Slim\Views\Twig('templates', [
'cache' => 'cache'
]);
$view->addExtension(new \Slim\Views\TwigExtension
(
$c['router'],
$c['request']->getUri()
));
return $view;
};
// Create app
$app = new \Slim\App($container);
// Render Twig template in route **
$app->get('/', function ($request, $response, $args)
{
return $this->view->render($response, 'login.twig',
[
'name' => "Log In"
]);
})->setName('home');
// Run app
$app->run();
Notes for this code:
** in the documentation, this snippet was originally
$app->get('/hello/{name}', function ($request, $response, $args)
{
return $this->view->render($response, 'profile.html', [
'name' => $args['name']
]);
})->setName('profile');
I changed the code
$app->get('/hello/{name}', function ($request, $response, $args)
to
$app->get('/', function ($request, $response, $args)
as I wanted this to catch requests to my home page, and then I also changed
'name' => $args['name']
to
'name' => "Log In"
As a quick way to kill an error message for the {name} component that I'm not using on my page. As I wasn't passing in any args I decided to just hard code this to a String value as a quick hack to make everything work smoothly :p.
Sources:
Main code: http://www.slimframework.com/docs/
Twig component: http://www.slimframework.com/docs/features/templates.html
Error handling: http://www.slimframework.com/docs/handlers/error.html
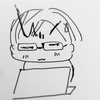
yuehwencheng
29,154 PointsThe point is about finding the file path of Slim.php within installed Slim folder.
Given version differences and where you choose to install composer, Slim.php might be located differently, so try to locate the file and include the file path in your code. For instance: http://postimg.org/image/bm7sbkfc5/

Graeme Campbell
15,594 PointsVery helpful Branden! Thank you!!

osman karataş
17,107 PointsThank you very much.