Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial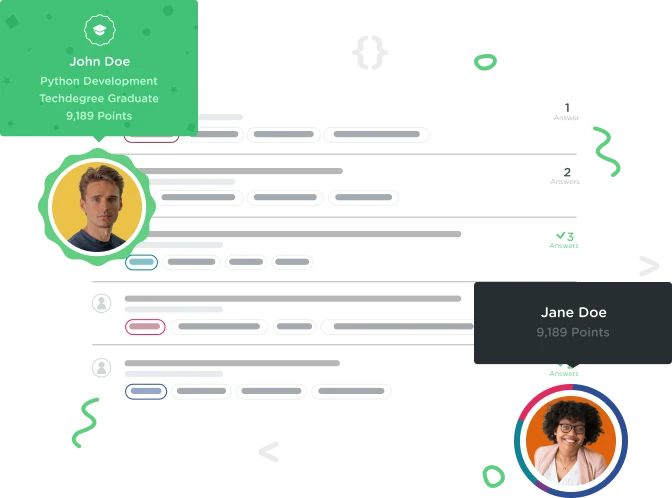

Roderick Kar
8,456 Pointsfatal error with numberOfRowsInSection written in Swift
i am trying to follow the lesson using Swift. i got a "fatal error: unexpectedly found nil while unwrapping an Optional value" for "let count". i am certain self.allUsers!.count is getting returning a value inside videDidLoad() but i think the way i am doing now in numberOfRowsInSection function to return the same value as integer is incorrect. I would be most grateful if help can be provided.
Thanks.
class EditFriendsViewController: UITableViewController{
var allUsers : [PFUser]?
override func viewDidLoad() {
super.viewDidLoad()
var query = PFUser.query()
query.orderByAscending("username")
query.findObjectsInBackgroundWithBlock {
(objects: [AnyObject]!, error: NSError!) -> Void in
if error == nil {
self.allUsers = objects as? [PFUser]
println("Successfully retrieved \(self.allUsers!.count) users.")
self.tableView.reloadData()
} else {
println("Error: \(error.userInfo)")
}
}
}
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return 1
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
let count = self.allUsers!.count as Int
return (count)
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let row = indexPath.row
let cell = tableView.dequeueReusableCellWithIdentifier("cell", forIndexPath: indexPath) as UITableViewCell
let user = self.allUsers![row]
cell.textLabel!.text! = "Hello"
return cell
}
}
2 Answers

Stone Preston
42,016 Pointsyour allUsers variable is probably nil at first until the network request comes back and it gets set. So when the tableView first appears allUsers is nil, and you are explicitly unwrapping it (basically saying, I know this is not nil so go ahead and use it) and getting this error. you probably need to actually unwrap it and return the count if its not nil, and return some other number if it is nil.
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
if let count = self.allUsers?.count as Int {
return (count)
} else {
//allUsers is nil, so just return 0
return 0
}
}
then whenever you get your allUsers back from parse, you reload the tableView which will cause the delegate methods to get called again and this time around it wont be nil, so it will actually return the count of the users

Chris Bracewell
34 PointsRoderick, how did you get the username of each object to print to each table cell? I'm covering it to Swift too and can't seem to find a way of doing it.
Any help would be great.
The code snippet is: pfuser *user = [self.allusers objectAtIndex:indexPath.row]; celll.textlabel.text = user.username;
Roderick Kar
8,456 PointsRoderick Kar
8,456 PointsHello Stone,
Thanks a lot for your help and suggestion. It makes a lot of sense and it's working now. Just a note, apparently my original code of downcasting allUsers.count as Int generates an error if used as a condition, removing the downcast solves the problem. I can move on to the rest of the lessons.
Many thanks again!
Regards,
Rod
Chris Bracewell
34 PointsChris Bracewell
34 PointsHi Stone, how would you convert the text that sets the cell label to the username into Swift? The way that is used in the tutorial is:
Any help would be great, thanks