Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial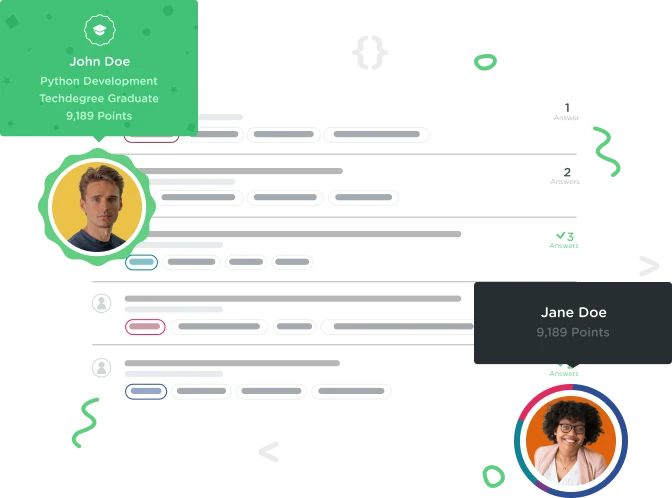

John Weland
42,478 PointsFatal signal 4 (SIGILL), code 2, fault addr
So following along with Ben Jakuben in this lesson I am throwing an error:
01-10 17:05:49.970 1959-1978/johnweland.me.stormy W/EGL_emulation﹕ eglSurfaceAttrib not implemented
01-10 17:05:49.970 1959-1978/johnweland.me.stormy W/OpenGLRenderer﹕ Failed to set EGL_SWAP_BEHAVIOR on surface 0xa46192a0, error=EGL_SUCCESS
01-10 17:05:50.065 1959-1977/johnweland.me.stormy A/libc﹕ Fatal signal 4 (SIGILL), code 2, fault addr 0xb487ad66 in tid 1977 (7.8267,-122.423)
Particularly the last line I notice its not actually taking the full location of (37.8267,-122.423) its only showing (7.8267,-122.423).
I am sure its a simple fix something missing that should be there or something there that shouldn't but I'm not seeing it. I'm having to split screen the Treehouse site and Android Studio so I am fairly certain its just something I'm not seeing.
My MainActivity.java file
package johnweland.me.stormy;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import com.squareup.okhttp.Call;
import com.squareup.okhttp.Callback;
import com.squareup.okhttp.OkHttpClient;
import com.squareup.okhttp.Request;
import com.squareup.okhttp.Response;
import java.io.IOException;
public class MainActivity extends ActionBarActivity {
public static final String TAG = MainActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey = " ######### ";
Double latitude = 37.8267;
Double longitude = -122.423;
String forecastURL = "https://api.forecast.io/forecast/" + apiKey +
"/" + latitude + "," + longitude;
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastURL)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
}
@Override
public void onResponse(Response response) throws IOException {
try {
if (response.isSuccessful()){
Log.v(TAG, response.body().string());
}
} catch (IOException e) {
Log.e(TAG, "Exception caught", e);
}
}
});
}
}
My AndroidManafest.xml file
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="johnweland.me.stormy" >
<uses-permission android:name="android.permission.INTERNET" />
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
1 Answer

Ben Jakuben
Treehouse TeacherHey John! Your code looks okay except that Double
should be lowercase (double
) to use the primitive type. With a capital D it's the full object version of the Double class, but it actually works the same either way.
Can you paste more of the contents of the error in logcat?
John Weland
42,478 PointsJohn Weland
42,478 PointsOk I changed the Double to double in both instances. here is my entire log file. Not this time it ran for a second (showed "hello world", and then crashed with a "Unfortunately, Stormy has stopped").
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherHmmm...no help in the logcat there. Are you using the default emulator, Genymotion, or a device? Can you zip up your project and attach a Dropbox link or something here, or email me the files?
John Weland
42,478 PointsJohn Weland
42,478 PointsOk Ben Jakuben here is the dropbox https://www.dropbox.com/s/n0kdw858fl9fiyx/Stormy.zip?dl=0
I am using Genymotion on a windows 8.1 machine, AMD first gen APU, 8GB DDR1333 if any of that helps.
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherThanks for the code. It works fine on my machine (of course). :-/ I am using Genymotion as well. Can you try it on the default emulator or a device? Or maybe even a different emulator within Genymotion? Which emulator version are you using in Genymotion? I ran mine on "Google Nexus 5 - 5.0.0 - API 21".
John Weland
42,478 PointsJohn Weland
42,478 PointsI am going to try my nexus 5 device running 5.0.1. I was using the same Genymotion emulator you were (the nexus 5 5.0.0 API 21).
What is your setup? Mac? Windows?
John Weland
42,478 PointsJohn Weland
42,478 Pointsupdate. took me a few to get it my device to connect with my PC but Stormy runs fine on my actual nexus 5
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherGlad to hear it! I'm on a Mac. Looks like this might be a Genymotion bug. Their 5.0 image is pretty new (naturally). Maybe you can revert to a 4.4 image on Genymotion for now and use your device for testing 5.0.
John Weland
42,478 PointsJohn Weland
42,478 PointsI'll try that, I'll let you know if it works. Although the whole thing runs faster on using my device over the emulator. I might stick with that after I check this Genymotion thing out. Took a vacation day to sit at home and code. My wife would laugh if she was home because I only have one monitor, so I am using my tablet and her laptop to run webpages/videos so I can display AS on my screen on the PC! (NERD POWER)
Ben Jakuben
Treehouse TeacherBen Jakuben
Treehouse TeacherGood! Testing on a device is generally best anyhow. I go between that and Genymotion depending on what I'm doing.
Multi-screen learning is the only way to go!
John Weland
42,478 PointsJohn Weland
42,478 PointsI'm building the 4.4 Nexus 5 emulator (API 19) now.... and it works, its got to be something with API 21 and I am guessing windows 8. I'll pull it up at work tomorrow and try there using API 21 and windows 7.
Yeah well my wife went to school for graphic design and I'm a web developer/ code junkie. She prefers mac, I've never really used one other than a few minutes here and there. If I had a spare 4 grand or so I'd build a nice new hackintosh and it would last us YEARS and with Chimera I could dual (or tri) boot and still have Windows. AND have that nice display... for 4 grand I'm including one of those nice new LG 21:9 monitors (they function at two screens side by side without the bezel you'd have from having two actual monitors sitting side by side.)
John Weland
42,478 PointsJohn Weland
42,478 PointsNo dice, I tried to run it on the Windows 7 machine with the Nexus 5 5.0.0 emulator (genymotion) and the whole system froze up for about 10 minutes before crashing.