Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial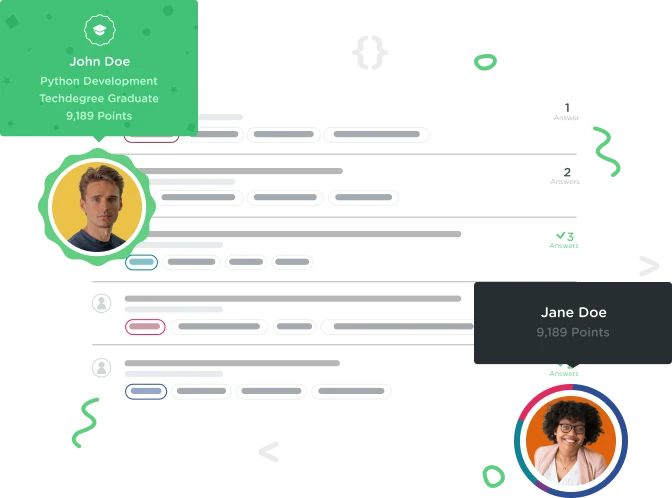
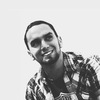
Matias Nunes
7,748 PointsFatalErrorException in TodoListController.php
Hi,
I was following Hampton's steps on "Linking Routes to Controllers" , but when i did , I got this exception :
FatalErrorException in TodoListController.php line 19: Class 'App \ Http \ Controllers \ View ' not found
Now , I know there is no " View" folder, because it's name is actually "Views", and also it's not on that path, but inside 'resources' folder.
How do I fix this? Or why it is looking in the wrong folder ?
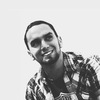
Matias Nunes
7,748 PointsHi Darryn,
I've just posted the code on the answer below. Thanks in advance.
5 Answers

Christopher Rebacz
10,904 PointsIt definitely looks like laravel 5, which is what you will get if you downloaded laravel using comiser with the --prefer-dist flag.
As has been mentioned, the error is due to the fact that you are inside a name spaced directory so PHP is looking for a View class in your controllers directory.
There are a couple of ways to fix the error. You could just precede the facade with a backward slash:
\View::make('todos.index');
In Laravel 5, it is often simpler to use the helper function view (). For example,
view ('todos.index');
With the helper, you don't need the declare the make method.
That said, Hamptons course is using Laravel 4.2 and there are enough differences between 4.2 and 5 that you'll get a lot of similar errors as you move through the course. I would recommend using the 4.2 version as you take the course. Most of the information you will learn is still applicable to Laravel 5.
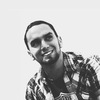
Matias Nunes
7,748 PointsThanks, Christopher. I'm on my way to reinstall the software from the top :)
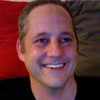
Darryn Smith
32,043 PointsYeah you're probably right, it's probably the version, because I can see in the video that Artisan didn't put all that namespace stuff in the controller file like it does now.
Wish I could help you further, but I haven't cracked open 5 yet myself. I'm betting re-doing the lesson in 4 would fix the problem, but if you don't want to do that (and I can't blame you), it'd take someone more experienced than me to conform it to whatever L5 does now.
Good luck!
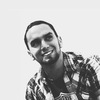
Matias Nunes
7,748 PointsHere goes the code if TodoListController.php:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Requests;
use App\Http\Controllers\Controller;
class TodoListController extends Controller
{
/**
* Display a listing of the resource.
*
* @return Response
*/
public function index()
{
return View::make('todos.index');
}
And this is the code from routes.php:
<?php
Route::get('/', 'TodoListController@index');
Route::get('/todos', 'TodoListController@index');
Route::get('/todos/{id}', function ($id)
{
return View::make('todos.show')->withId($id);
});
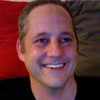
Darryn Smith
32,043 PointsStill digging around a bit, as I did this course recently.
Some clues I'm following though:
I don't think this error you're getting is telling you that there's a problem with the path to the 'Views' directory, rather, it's saying that it can't find the 'View' class in the 'App \ Http \ Controllers \ ' namespace.
I'm looking at my code for this course, and I don't see anywhere the namespace declarations that you have at the head of your ToDoListController file:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Requests;
use App\Http\Controllers\Controller;
Nor does my class say it extends 'Controller', but instead extends \BaseController"
Where did all of that opening namespacing code come from? I don't see it in Hampton's code in the video, nor in his sample project files, and In my project, I simply have:
<?php
class TodoListController extends \BaseController {
/**
* Display a listing of the resource.
*
* @return Response
*/
public function index()
{
return View::make('todos.index');
}
// etc. etc. etc.
As an experiment, you might try replacing the beginning declarations in your TodoListController class from the beginning up to the first line of the class definition ( so that it begins like this:)
<?php
class TodoListController extends \BaseController {
// etc. etc. etc.
and see if it works?
I hope that makes a little sense...
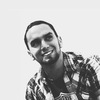
Matias Nunes
7,748 PointsThe code was created automatically when I executed "php artisan controller:make TodoListController". I've tried with your code just in case, but it didn't worked either.
I don't know what else could be wrong because I just followed every step on the video. Could it be a version problem with Laravel?
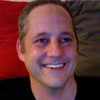
Darryn Smith
32,043 PointsThat's a possibility. The course uses Laravel 4, are you using 5?
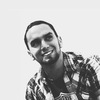
Matias Nunes
7,748 PointsYep. I'll look into it from there. Maybe reinstalling Laravel or something. Thank you again.
Darryn Smith
32,043 PointsDarryn Smith
32,043 PointsIf you post the code it may be possible to determine what's going wrong with it.