Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial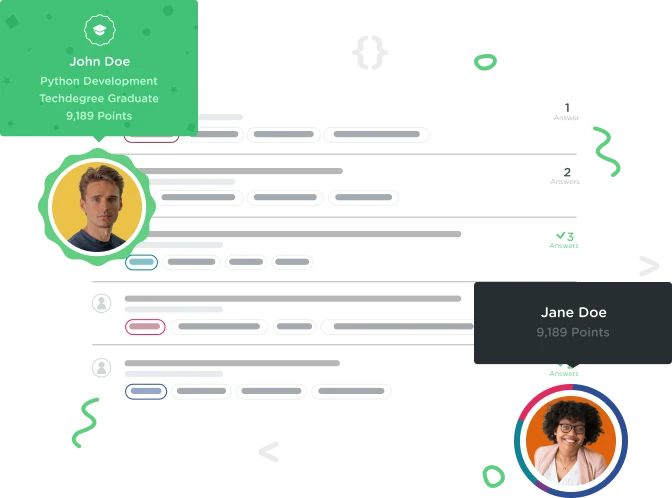
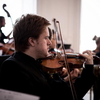
Adam Hansen
Courses Plus Student 4,716 PointsFeedback on my code
I added the last bit of code for the "#username" field. I chose to check if there was entered more than 0 characters into the field, but perhaps there is more direct way of checking? Here is my code from app.js:
//Problem: Hints are shown even when form is valid
//Solution: Hide and show them at appropriate times
var $username = $("#username");
var $password = $("#password");
var $confirmPassword = $("#confirm_password");
//Hide hints
$("form span").hide();
function isUsernamePresent() {
return $username.val().length > 0;
}
function isPasswordValid() {
return $password.val().length > 8;
}
function arePasswordsMatching() {
return $password.val() === $confirmPassword.val();
}
function canSubmit() {
return isPasswordValid() && arePasswordsMatching() && isUsernamePresent();
}
function usernameEvent() {
//Find out if text entered
if(isUsernamePresent()) {
//if valid, hide hint
$username.next().hide();
} else {
//else show hint
$username.next().show();
}
}
function passwordEvent() {
//Find out if password is valid
if(isPasswordValid()) {
//if valid, hide hint
$password.next().hide();
} else {
//else show hint
$password.next().show();
}
}
function confirmPasswordEvent() {
//Find out if password and confirmation match
if(arePasswordsMatching()) {
//if match, hide hint
$confirmPassword.next().hide();
} else {
//else show hint
$confirmPassword.next().show();
}
}
function enableSubmitEvent() {
$("#submit").prop("disabled", !canSubmit());
}
//When event happens on password input
$password.focus(passwordEvent).focus(usernameEvent).keyup(passwordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent);
//When event happens on confirmation input
$confirmPassword.focus(confirmPasswordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent);
enableSubmitEvent();
1 Answer

LaVaughn Haynes
12,397 PointsYour code would verify length just fine. As far as I know that's the best way to do it... but keep in mind that the user would still be able to enter a blank space or even 8 blank spaces if you only check length. You could also validate on other criteria as well. Obviously you don't need to for the task but I thought I'd mention it. Copy and run this in a browser if it doesn't make sense.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Has Length</title>
</head>
<body>
<!-- enter a blank space. it will pass -->
<input type="text" id="username" autocomplete="off">
<button>Submit</button>
<p id="logPresent">...</p>
<p id="logValid">...</p>
<p id="loglettersNums">...</p>
<script src="https://code.jquery.com/jquery-1.11.3.min.js"></script>
<script>
var $username = $('#username'),
$button = $('button'),
$logPresent = $('#logPresent'),
$logValid = $('#logValid'),
$loglettersNums = $('#loglettersNums');
function isUsernamePresent(){
//this allows 1 blank space to return true
return $username.val().length > 0;
}
function isUsernameValid(){
//blank spaces don't count unless it's between other characters...returns false
return $username.val().trim().length > 0;
}
function isUsernameLettersAndNums(){
//a different type of validation...username can only be letters and numbers
return $username.val().match(/[^a-zA-Z0-9]/) === null && isUsernamePresent();
}
$button.on('click', function(){
$logPresent.html( '<strong>Length is present:</strong> ' + isUsernamePresent() );
$logValid.html( '<strong>Length is valid:</strong> ' + isUsernameValid() );
$loglettersNums.html( '<strong>Chars are letters and numbers only:</strong> ' + isUsernameLettersAndNums() );
});
</script>
</body>
</html>