Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial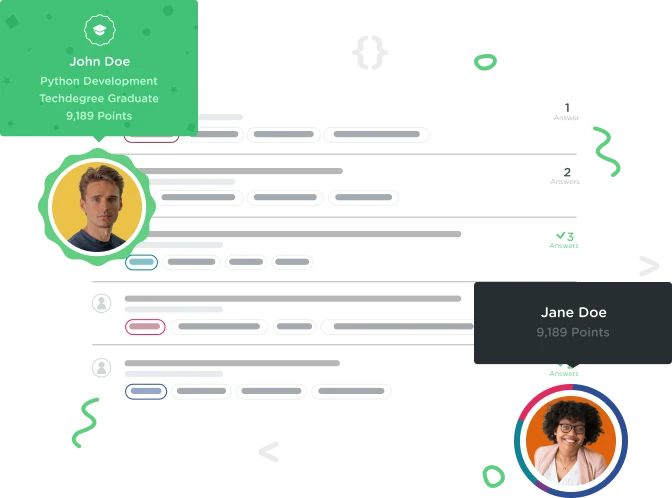

Joe Worthington
1,329 PointsFeedback on my code for The Build an Object Challenge, Part 2
I did write the program using a for in loop to begin with but I have been watching a youtube channel called FunFunFunction and he shows a different method to loop through arrays. I wanted to try and use a functional approach and managed to get it working.
//Build an object challenge
//Instructions and steps
/*
1. Write a script that creates a collection of student records
2. Then print the students and their details to the webpage
Steps:
Create a data structure to hold information about a group of students
Create an array named students
This will hold a list of objects
Each object represent a student
Each object will contain the following properties.
name
track
achievements - number value
points - number value
at least 5 student objects
Print out all the students along with their info to the webpage
*/
var html = ''
var students = [
{
name : 'Joe Worthington',
track : "JavaScript Full Stack",
achievements : 9,
points : 1089
},
{
name : "Henrik Christensen",
track : "JavaScript Full Stack",
achievements : 48,
points : 4141
},
{
name : "Rory Baxter",
track : "Game Develoment",
achievements : 39,
points : 3035
},
{
name : "Steven Parker",
track : "Ruby",
achievements : 256,
points : 24164
},
{
name : "Sarah Augustine",
track : "Ruby",
achievements : 83,
points : 5937
}
];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
var studentClass = students.map(function(student) {
return '<p><strong>' + student.name + '</strong></p>' + '<p>Track: ' + student.track + '</p>' + '<p>Achievements: ' + student.achievements + '</p>' + '<p>Points: ' + student.points + '</p>'
})
print(studentClass.join(" "));
3 Answers
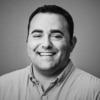
mikes02
Courses Plus Student 16,968 PointsSo I changed my mind, I wanted to make this more flexible, so removed the self invoking function and made this accept parameters:
var students = [
{
name : 'Joe Worthington',
track : "JavaScript Full Stack",
achievements : 9,
points : 1089
},
{
name : "Henrik Christensen",
track : "JavaScript Full Stack",
achievements : 48,
points : 4141
},
{
name : "Rory Baxter",
track : "Game Develoment",
achievements : 39,
points : 3035
},
{
name : "Steven Parker",
track : "Ruby",
achievements : 256,
points : 24164
},
{
name : "Sarah Augustine",
track : "Ruby",
achievements : 83,
points : 5937
}
];
function getStudentInfo(group, output) {
var people = group.map(function(student) {
return '<p><strong>' + student.name + '</strong></p>' + '<p>Track: ' + student.track + '</p>' + '<p>Achievements: ' + student.achievements + '</p>' + '<p>Points: ' + student.points + '</p>';
});
return document.querySelector(output).innerHTML = people.join(" ");
}
getStudentInfo(students, '#output');
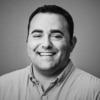
mikes02
Courses Plus Student 16,968 PointsYou can clean this up a bit by consolidating your variables and then just using a single self invoking function to display results, not sure what the empty html variable was for so I removed it,
var outputDiv = document.getElementById('output'),
students = [
{
name : 'Joe Worthington',
track : "JavaScript Full Stack",
achievements : 9,
points : 1089
},
{
name : "Henrik Christensen",
track : "JavaScript Full Stack",
achievements : 48,
points : 4141
},
{
name : "Rory Baxter",
track : "Game Develoment",
achievements : 39,
points : 3035
},
{
name : "Steven Parker",
track : "Ruby",
achievements : 256,
points : 24164
},
{
name : "Sarah Augustine",
track : "Ruby",
achievements : 83,
points : 5937
}
],
displayMessage = (function() {
var studentClass = students.map(function(student) {
return '<p><strong>' + student.name + '</strong></p>' + '<p>Track: ' + student.track + '</p>' + '<p>Achievements: ' + student.achievements + '</p>' + '<p>Points: ' + student.points + '</p>';
});
return outputDiv.innerHTML = studentClass.join(" ");
}());

Joe Worthington
1,329 PointsWow, mikes02! Thanks for the feedback.
I will take the time to go through what you have done and truly understand it.
Really appreciate the effort, as it all goes towards helping me improve!
Cheers
Joe