Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial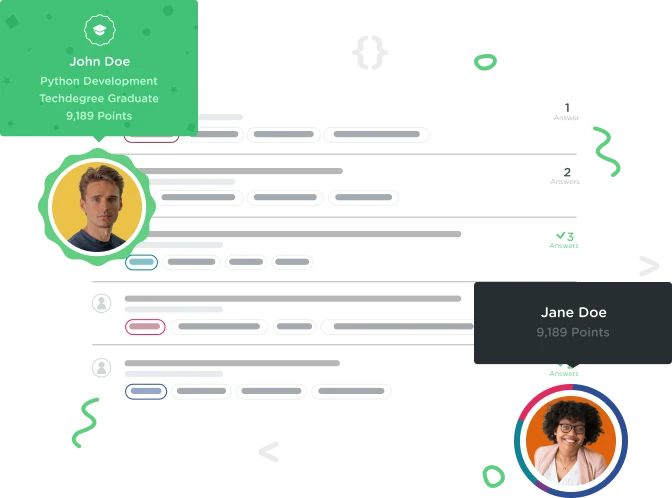
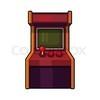
cyber voyager
8,859 PointsFeedback on my script
Hello, I just created my own version of the shopping list, a little differently tho. I would love if you could give me some feedback on it (Things I should avoid, Refactoring tips) I would also like to ask how to make an if statement to check if both strings exist in a list, I will highlight the spots on the code. The script will create a txt file at the location of the py script.
# Ask for an item to add to the list
# Move them to another function which shows the first item with an (1) position
# Add a help menu
# Clearing the screen
# A way to exit the program
# Auto updating list (When ever they add an item append it to the list clear the screen and display the items)
import os
import sys
import time
li_items = []
def clear(): # <--- Clears the screen
os.system("cls" if os.name == "nt" else "clear")
def intro(): # <--- Introduce the program to the user
clear()
print("\nTo-Do List!")
print("""
If you feel lost with the commands just
type ('Help') to see all the commands available
with information on what they do
""")
move = input("Should I proceed? ('Y/n) ").lower()
if move == 'y':
main()
else:
exit = input("\nWould you want to exit? ('Y/n) ").lower()
if exit == 'y':
print("\nGood Byee!")
time.sleep(1.5)
sys.exit()
else:
clear()
intro()
def main(): # <--- Where all the work is done!
clear()
index = 1
print("\nAdd your items here!")
for item in li_items:
print("\n{}. {}".format(index, item))
index += 1
print('\n' + '=' * 20)
items = input("\n> ")
if 'Help' in items:
helper()
input("Whenever you are done press ('Enter') to continue ")
main()
if 'help' in items:
helper()
input("Whenever you are done press ('Enter') to continue ")
main()
if 'Exit' in items:
exit = input("\nAre you sure that you want to exit the Script? ('Y/n) ").lower()
if exit == 'y':
print("\nGood byee..")
time.sleep(0.5)
clear()
sys.exit()
else:
main()
if 'exit' in items:
exit = input("\nAre you sure that you want to exit the Script? ('Y/n) ").lower()
if exit == 'y':
print("\nGood byee..")
time.sleep(0.5)
clear()
sys.exit()
else:
main()
if 'Rmv' in items:
delete = input("\nWhich item should I remove? ")
if delete in li_items:
li_items.remove(delete)
clear()
main()
if len(delete) == 0:
input("\nYou forgot to type something.. Press ('Enter') to proceed ")
main()
elif delete not in li_items:
input("\nSorry but this item doesn't exist! Press ('Enter') to proceed ")
clear()
main()
if 'rmv' in items:
delete = input("\nWhich item should I remove? ")
if delete in li_items:
li_items.remove(delete)
clear()
main()
if len(delete) == 0:
input("\nYou forgot to type something.. Press ('Enter') to proceed ")
main()
elif delete not in li_items:
input("\nSorry but this item doesn't exist! Press ('Enter') to proceed ")
clear()
main()
if 'Done' in items:
x = li_items
readyTxt = '\n'.join(str(y) for y in x)
print("\nCreating your file...!")
print("\nCreated!")
file = open('ShoppingList.txt', 'w')
file.write(readyTxt)
file.close()
time.sleep(1)
clear()
main()
if 'done' in items: #(HERE I would like to know how to do something like the bellow)
x = li_items #(If 'done', 'Done' in items: ) By the way I tried or and it dosn't do what I
readyTxt = '\n'.join(str(y) for y in x) # need
print("\nCreating your file...!")
print("\nCreated!")
file = open('To-Do List.txt', 'w')
file.write(readyTxt)
file.close()
time.sleep(1)
clear()
main()
if len(items) > 0:
li_items.append(items)
main()
else:
print("Please type something!")
time.sleep(0.5)
main()
def helper():
print("""\nAvailable Commands:\n
-> Help ('Shows all the commands available in the Script')
-> Done (Creates your file)
-> Rmv ('Removes the item that you provided')
-> Exit ('Exits the Script')
""")
intro()