Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial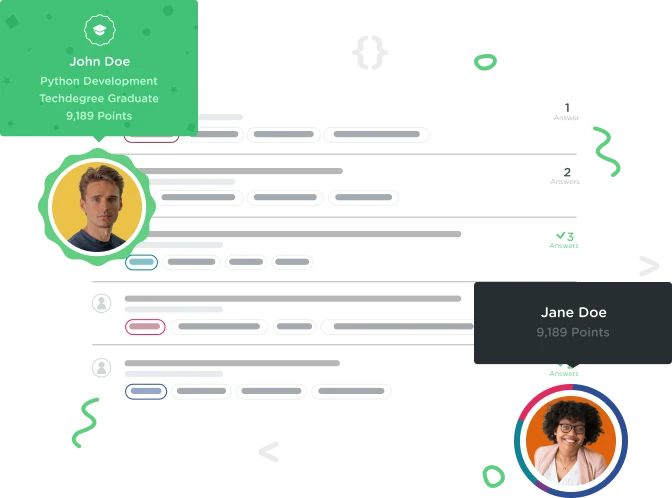

Joseph Tavinor
8,397 PointsFeedback on solution please
//Array of student objects
let students = [
{
name: "Manuel",
track: "Python",
acheivements: 30,
points: 1560,
},
{
name: "Jose",
track: "Javascript",
acheivements: 23,
points: 450,
},
{
name: "Sarah",
track: "HTML",
acheivements: 53,
points: 1890,
},
{
name: "Tess",
track: "C+",
acheivements: 2,
points: 67,
},
{
name: "Coolio",
track: "PHP",
acheivements: 56,
points: 2013,
},
];
//Sets output as empty string and selects <div> with id="output"
let output = ''
let html = document.querySelector("#output");
//Iterates through each student object in the students array
for ( let student in students ) {
// Iterates through each key-value pair in the current student object
for ( let key in students[student] ) {
// Puts current value in a variable for readability and capitalises first letter of the key
let value = students[student][key];
let keyCapitalized = key.charAt(0).toUpperCase() + key.slice(1);
// Puts each name key-value pair as a <h2> and all other pairs as <p> and adds to output string
if ( key === 'name' ) {
output += `<h2><strong>${keyCapitalized}: ${value}</strong></h2>`;
} else {
output += `<p>${keyCapitalized}: ${value}</p>`;
}
}
// Line break after each student object
output += '<br>'
}
// Writes the output string into the HTML
html.innerHTML = output;
2 Answers
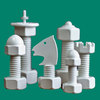
Steven Parker
231,275 PointsIterating through the properties can be a useful technique But you should be aware of some behavioral differences from the original code that will occur:
- if a property is missing, it will be silently omitted where the original will show the property as "undefined"
- if any extra properties are added, they will be listed where the original would show only the intended ones
- the order that the properties are listed is no longer guaranteed

Victor Mercier
14,667 PointsNice way to see the problem!
Joseph Tavinor
8,397 PointsJoseph Tavinor
8,397 PointsThanks for the feedback, do I understand correctly?:
Also can you explain why the order is not guaranteed? I thought a for .. in loop always iterated from first to last
Steven Parker
231,275 PointsSteven Parker
231,275 PointsWhat I meant was that the original method could output the properties in a specific order each time regardless of what the class structure was like.