Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial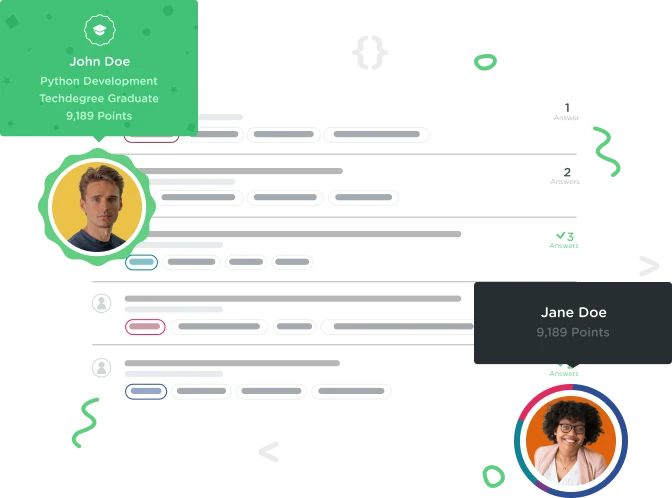

serhiipanchyshyn
2,485 PointsFeedBack on the code for the challenge.
What do you think about my answer? Anything I can change or improve?
/*
This program takes user inputs
and evaluates them against the correct answers
*/
// Collects users answers
const question1 = prompt(`Name a programming language that's also a gem`); // Ruby
const question2 = prompt('What is another word for lexicon?'); // Dictionary
const question3 = prompt('Name the seventh planet from the sun'); // Uranus
const question4 = prompt('Name the worlds largest ocean'); // Pacific
const question5 = prompt('What kind of weapon is a falchion?'); // Sword
let correctAnswers = 0;
// Check if the questions answer is correct
if ( question1.toUpperCase() === 'RUBY' ) {
correctAnswers += 1;
}
if (question2.toUpperCase() === 'DICTIONARY' ) {
correctAnswers += 1;
}
if ( question3.toUpperCase() === 'URANUS' ) {
correctAnswers += 1;
}
if (question4.toUpperCase() === 'PACIFIC' ) {
correctAnswers += 1;
}
if (question5.toUpperCase() === 'SWORD' ) {
correctAnswers += 1;
}
// prints out how many questions were answered right
if ( 5 === correctAnswers ) {
document.write(`<p>You answered 5 out 5 right!<p>`);
} else {
document.write(`<p>Sorry. You answered ${correctAnswers} out 5 right!.<p>`);
}
if (correctAnswers === 5 ) {
document.write(`<p><strong>You earned a gold crown!</strong></p>`);
} else if ( correctAnswers >= 3 ) {
document.write(`<p><strong>You earned a silver crown!</strong></p>`);
} else if ( correctAnswers >= 1 ) {
document.write(`<p><strong>You earned a bronze crown!</strong></p>`);
} else {
document.write(`<p><strong>No crown for you.</strong></p>`);
2 Answers
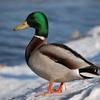
Robert Schaap
19,836 PointsHad to see what you've learned so far... but with what you've done I'd say it's pretty good. You could consider calling the .toUpperCase() method directly on the question like so:
const question4 = prompt('Name the worlds largest ocean').toUpperCase()
That would make the if-statements a bit easier to read. Also, I'd change if ( 5 === correctAnswers )
to if ( correctAnswers === 5 )
, like you did a few lines down. It reads like you would say it out loud normally.
Once you get a bit further in the course, you'll probably figure it's easier to store stuff in arrays or objects, then you just have something loop over it. Good luck :)

Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsHey you have done a good job . I have some suggestions which i think make the program more realistic: 1.
if ( correctAnswers === 5) {
document.write(`<p>You answered 5 out 5 right!<p>`);
}
will look more readable. 2.Instead of just storing the value in "correctAnswers" , you can write something like this:
if( response5.toUpperCase() === "MURALIDHARAN"){
correct_response += 1;
alert("Yep....Thats right!! " + "[ " +correct_response + " ]");
}
This will provide a glimpse of correct answers as you answer corrctly. Happy Coding :)