Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial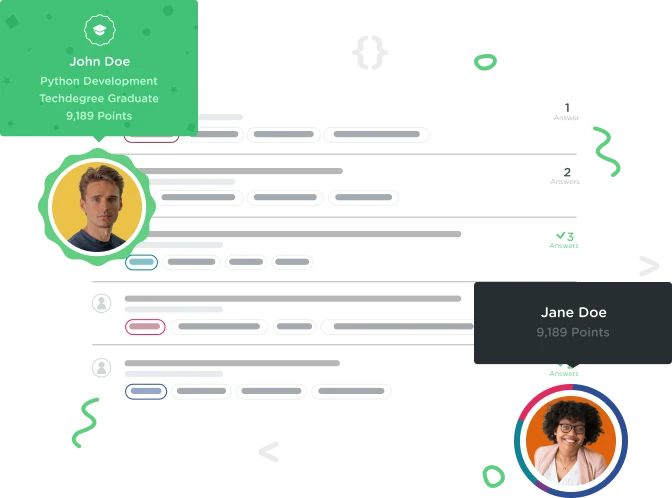
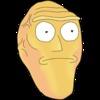
Bryce Archer
Courses Plus Student 1,511 PointsFeedback: this problem was confusing or could be improved upon.
I ended up using the Character.getNumericValue
static class method to convert the input's first character to its integer equivalent. Alternatively, I could have built a hash map that stores the letters and the indices in the English alphabet, and looked up the input. Either way, it is unclear how this problem should be solved from the previous lesson, or requires looking at confusing outside documentation. After rewatching the video to see if I had missed something, none of the classes or methods introduced there follow directly with the problem space in the exercise.
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
1 Answer

andren
28,558 PointsThis challenge has two files in its workspace ConferenceRegistrationAssistant.java
and Example.java
. The Example.java
file contains info that was designed to make this task easier.
It explains that you can compare chars to each other directly without converting them a number first 'a' < 'c'
for example would result in true
. If you combine that with the charAt
method shown off earlier in the course you get a pretty simple solution:
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
if (lastName.charAt(0) <= 'M') {
lineNumber = 1;
} else {
lineNumber = 2;
}
return lineNumber;
}
While I agree that a demonstration of this fact should have been included earlier in the course, instead of just placing it in a file in the challenge. I wanted to clarify that Treehouse did provide a clue to the intended solution, they just placed it in a location that's easy to overlook.
It's also worth pointing out though that this is not the only challenge where large clues as to how to solve it will be found within other files in the challenge, so you should keep this in mind for future challenges.
Bryce Archer
Courses Plus Student 1,511 PointsBryce Archer
Courses Plus Student 1,511 PointsIndeed, it is easy to overlook to the extent that I was unaware of other files in the web IDE until now. Thanks for pointing that out! :)