Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial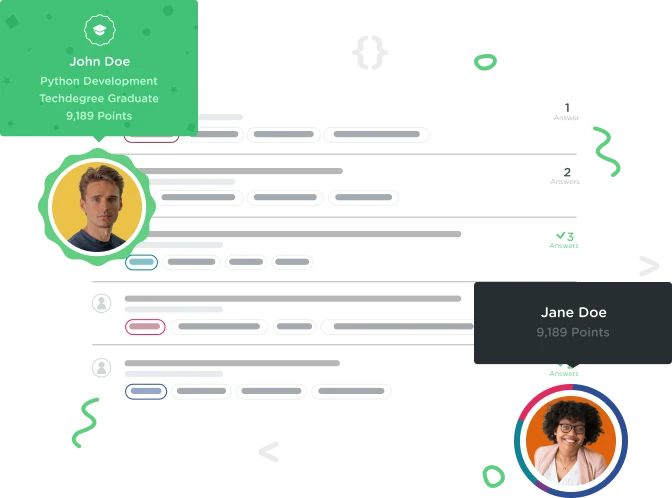
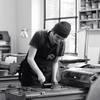
Unsubscribed User
3,325 PointsFeedback would be appreciated!
I got my solution to work, I wasn't sure how to move my questions from one array to another without them turning into single variable strings. So I checked the MDN and found the splice(); method which worked very well. However, since my solution is different than the one shown in the solution video, I am wondering if my solution is okay or should be improved upon.
function print(message) {
document.write(message);
}
var questions = [
['What is the first thing any programmer writes?', 'hello world', 'hello world!'],
['How many states are in the United States?', '50', 'fifty'],
['What is the best pet?', 'cat', 'cats']
];
var correctAnswers = [];
var wrongAnswers = [];
// create an ordered list of answered questions
function printQuestions ( answers ) {
var listHTML = '<ol>';
for (var i = 0; i < answers.length; i++ ) {
listHTML += '<li>' + answers[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML)
}
// ask the user questions and stores them
for (var i = 0; i < questions.length; i++) {
var answer = prompt(questions[i][0]);
if (answer.toLowerCase() === questions[i][1] || answer.toLowerCase() === questions[i][2]) {
correctAnswers.splice(0, 0, questions[i].shift() );
} else {
wrongAnswers.splice(0, 0, questions[i].shift() );
}
}
// print the amount of correctly answered questions
print ('You answered ' + correctAnswers.length + ' question(s) correctly.<br /><br />');
// print correctly answered questions
print('<strong>You answered these questions correctly:</strong>')
printQuestions( correctAnswers );
// print incorrectly answered questions
print('<strong>You answered these questions incorrectly:</strong>')
printQuestions( wrongAnswers );
Thank you for your help and feedback! Miles
1 Answer
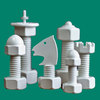
Steven Parker
231,269 PointsOne of "Parker's Rules of Programming" is "The more complex the task, the more ways there will be to legitimately arrive at a solution".
Using a different method to create a solution should not be cause for concern. In fact, as the assignments become more involved, it is quite possible to create a solution that is more efficient and/or concise than the course example. And it will always be possible to expand on the functionality.
The best learning opportunity will be to compare the differences between your approach and the one shown, and be sure you understand them. Sometimes you may learn something you overlooked, but may times it will simply be a case of "designer's choice".
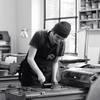
Unsubscribed User
3,325 PointsThank you, this gave me a lot to think about :)
Unsubscribed User
3,325 PointsUnsubscribed User
3,325 PointsI just finished watching the solution video and decided to tidy up the list printing section of the code: