Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial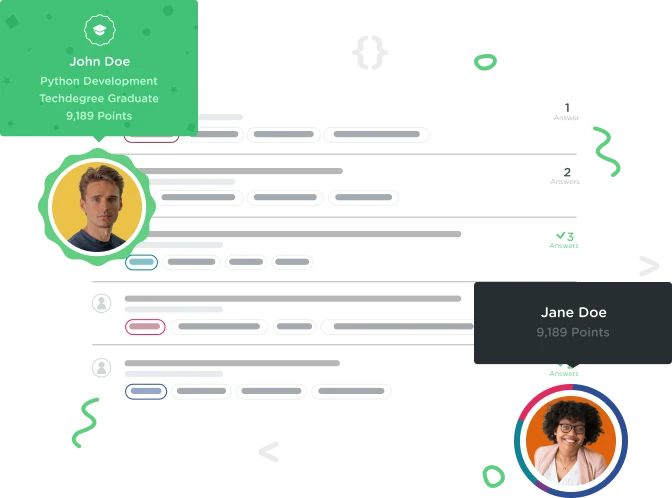

Alexisca hall
23,683 PointsFetch the associative array of $results to loop through, and add an additional "genres" array element to $item. This "ge
Fetch the associative array of $results to loop through, and add an additional "genres" array element to $item. This "genres" element should be an internal associative array with the key of genre_id and the value of genre.
<?php
$item = array("genres" => array(3 => "Comedy", 16 => "Sci-fi"));
echo "<pre>";
var_dump($item);
echo "</pre>";
$row = array("genre_id" => 5, "genre" => "Action");
$item["genres"][$row["genre_id"]] = $row["genre"];
$item["genres"][] = $row["genre_id"] = $row["genre"];
echo "<pre>";
var_dump($item);
echo "</pre>";
1 Answer
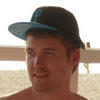
Algirdas Lalys
9,389 PointsHi Alexisca hall,
Ok we will start from begining. We have two variables $item and $results. So if we print_r($item); we can see that there is an array (You can check output in 'Preview') , something like that will show up.
Array (
[title] => Refactoring: Improving the Design of Existing Code
[category] => Books
[img] => img/media/refactoring.jpg
[format] => Hardcover
[year] => 1999
[publisher] => Addison-Wesley Professional
[isbn] => 978-0201485677
)
and we have a variable $results. If we print_r($results);
PDOStatement Object ( [queryString] => SELECT * FROM Media JOIN Media_Genres ON Media.media_id = Media_Genres.media_id JOIN Genres ON Media_Genres.genre_id = Genres.genre_id WHERE Media.media_id=? )
we can see that this is an PDO object which grabs some data from database and stores them inside object. Ok then we have to figure out how to extract that data and how many records do we have, so maybe we could use some PDO methods on $results object, let's try print_r($results->fetchAll(PDO::FETCH_ASSOC));
Array (
[0] => Array (
[media_id] => 3
[title] => Refactoring: Improving the Design of Existing Code
[img] => img/media/refactoring.jpg
[genre_id] => 17
[format] => Hardcover
[year] => 1999
[category] => Books
[genre] => Tech
)
[1] => Array (
[media_id] => 3
[title] => Refactoring: Improving the Design of Existing Code
[img] => img/media/refactoring.jpg
[genre_id] => 51
[format] => Hardcover
[year] => 1999
[category] => Books
[genre] => Business
)
)
oh great we have two arrays in that object. Hmm maybe we could somehow grab one record as long as there is records in that object and store them in temporary variable. Let's try while loop as previous Alena Video example shows us.
while($row = $results->fetch(PDO::FETCH_ASSOC)) {
echo print_r($row) . "<br><br>";
}
You can check preview windows and see that it prints out two records or two arrays. Well then it seems we have everything what we need. Let's check again what challenge asks us Fetch the associative array of $results to loop through, and add an additional "genres" array element to $item. This "genres" element should be an internal associative array with the key of genre_id and the value of genre. Great, then the final code should look something like that.
<?php
// We loop through every record as long as there is one and we will store it in temporary variable $row
while($row = $results->fetch(PDO::FETCH_ASSOC)) {
// We add associative array key 'genres' and inside we add new array with key 'genre_id' and value 'genre'
$item['genres'][$row['genre_id']] = $row['genre'];
}
// Then we can check how our $item array looks now in the preview.
print_r($item);
?>
I tryed as best as I can to describe this challenge as I understand. I hope it helps you.
Mark Ryan Holanda
4,223 PointsMark Ryan Holanda
4,223 PointsHi Algirdas,
Thank you for explaining this to us.. honestly, im a bit lost here as well... Alena's sample on the video is
$item[$row["role"]][] = $row["fullname"];
which is basically, different on the challenge.. (that's why im lost as well..) as far as I understand, the simple syntax on adding an item to an array is this:
$array_var["key"] = "value";
but, if i translate the one you supplied to complete the challenge, it looks something like this:
$array_var["other"]["key"] = "value";
which is for me, is quite new and havent thought that it was possible..
Thank you and appreciate your help.