Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial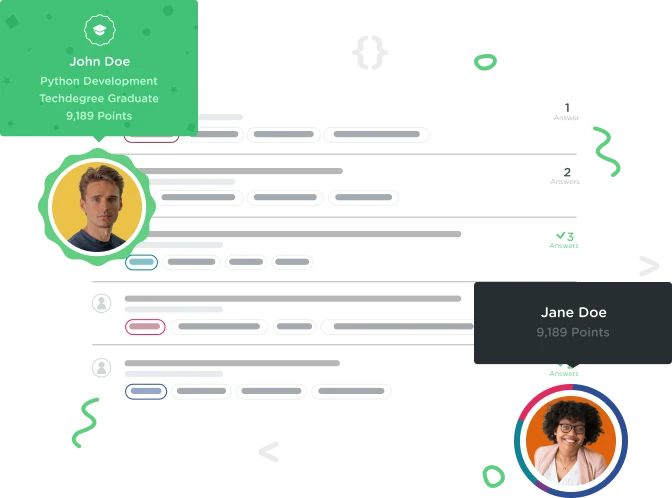
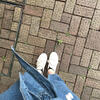
Tani Huang
6,952 PointsFew question about this project
1) Is it better add the";" semicolon the end of "}" ? When do we need it ?
Song.prototype.toHTML = function() {
var htmlString = '<li';
if(this.isPlaying) {
htmlString += ' class="current"';
} //no semicolon
htmlString += '>';
htmlString += this.title;
htmlString += ' - '
htmlString += this.artist;
htmlString += '<span class="duration">'
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};
2) this.nowPlayingIndex = 0; mean every time start at the first song ?
function Playlist() {
this.songs = [];
this.nowPlayingIndex = 0; //start at first song ?
}
3) Why we need to put .renderInElement(playlistElement) again ? refresh something?
var playButton = document.getElementById("play");
playButton.onclick = function() {
playlist.play();
playlist.renderInElement(playlistElement); // Why and what is this for?
}
4) How can we define this.isPlaying is true or false ? seem like it does not show anything but it can add current class...
Song.prototype.toHTML = function() {
var htmlString = '<li';
if(this.isPlaying) {
htmlString += ' class="current"';
}
htmlString += '>';
htmlString += this.title;
htmlString += ' - '
htmlString += this.artist;
htmlString += '<span class="duration">'
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};
5) Constructor functions normally use, when we need to create something that have the same content?
This link of the video:
https://teamtreehouse.com/library/objectoriented-javascript/constructor-functions-and-prototypes/making-the-ui-work
1 Answer
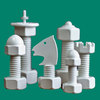
Steven Parker
231,172 Points- Semicolons are optional at the end of a statement if it is also the end of a line, but it is a "best practice".
- This is a constructor function, so the 0 is set only once when the object is created.
- Yes, the screen is updated to show that the item just clicked is currently playing.
- The "play" and "stop" methods change the value of "isPlaying".
- Yes, constructor functions create "instances" of similar objects.
Tani Huang
6,952 PointsTani Huang
6,952 Points1) base on question NO.2 and NO.3 - I guest I have wrong understanding of these two step..if click button what will happen to the renderInElement
-This is a constructor function, so the 0 is set only once when the object is created.
-Yes, the screen is updated to show that the item just clicked is currently playing.
2) base on question NO.4 - if(this.isPlaying) equal to something ??
Steven Parker
231,172 PointsSteven Parker
231,172 Pointstrue
orfalse
. So when it is true, the the code block after the "if" will run.