Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial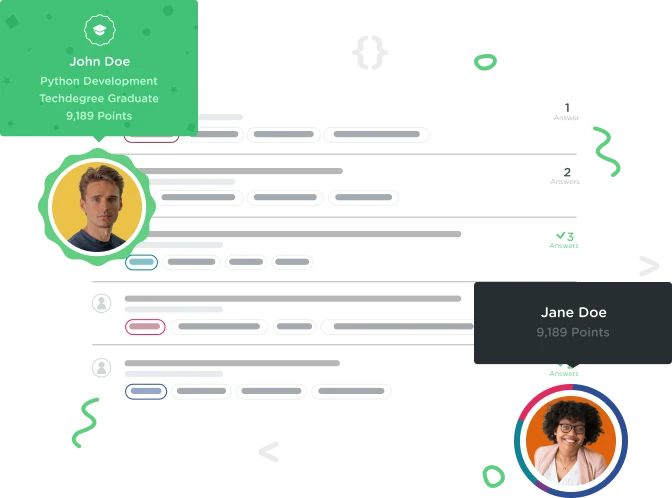

Brandon Johansen
1,439 PointsFigured it out but what's a better way?
So I figured it out and end result looks the same but I'm just curious if there's a better way. I know more concatanation would've probably made this a lot less time consuming. Anyway not urgent or anything because it did the job I'm just wondering.
//Start code
alert('Ready to do math?');
var num = prompt('Put in a number'); var num = parseFloat(num); var numTwo = prompt('Put in another one'); var num = parseFloat(num); var numTwo = parseFloat(numTwo); var add = num + numTwo; var Multiple = num * numTwo; var subtract = num - numTwo; var divide = num/numTwo; var message = 'Math with your numbers ' + num + ' and ' + numTwo; var addition = num + ' '; var additiontwo = numTwo + ' '; addition += ' + ' + additiontwo + '= '; var sub = num + ' '; var subTwo = numTwo + ' '; sub+= ' - ' + subTwo + ' = '; var multiplication = num + ' '; var multiplicationTwo = numTwo + ' '; multiplication+= ' * ' + multiplicationTwo + ' = '; var div = num + ' '; var divTwo = numTwo + ' '; div+= ' / ' + divTwo + ' = '; document.write('<h1>'+message+'</h1>'); document.write(addition + add); document.write("<br>"); document.write(sub + subtract); document.write("<br>"); document.write(multiplication + Multiple); document.write("<br>"); document.write(div + divide);
//End code
Can't wait to share ideas!!!

Kenneth Dubroff
10,612 PointsHere's his code in markdown
alert('Ready to do math?');
var num = prompt('Put in a number');
var num = parseFloat(num);
var numTwo = prompt('Put in another one');
var num = parseFloat(num);
var numTwo = parseFloat(numTwo);
var add = num + numTwo;
var Multiple = num * numTwo;
var subtract = num - numTwo;
var divide = num/numTwo;
var message = 'Math with your numbers ' + num + ' and ' + numTwo;
var addition = num + ' ';
var additiontwo = numTwo + ' ';
addition += ' + ' + additiontwo + '= ';
var sub = num + ' ';
var subTwo = numTwo + ' ';
sub+= ' - ' + subTwo + ' = ';
var multiplication = num + ' ';
var multiplicationTwo = numTwo + ' ';
multiplication+= ' * ' + multiplicationTwo + ' = ';
var div = num + ' ';
var divTwo = numTwo + ' ';
div+= ' / ' + divTwo + ' = ';
document.write('<h1>'+message+'</h1>');
document.write(addition + add);
document.write("<br>");
document.write(sub + subtract);
document.write("<br>");
document.write(multiplication + Multiple);
document.write("<br>");
document.write(div + divide);
What I see off the bat is too many lines of code. As a programmer reading your code, I should be able to easily follow along, but I'm getting lost because it's broken up into very small chunks. It's not wrong, I just think it could be more readable. Using string concatenation you can do something like:
var visitorNum1 = prompt("Please Enter a Number");
var visitorNum2 = prompt("Please Enter a Second Number");
var message = "<h1>Math with the numbers " + visitorNum1 + " and " + visitorNum2 + "</h1>";
//And the first math operation becomes:
message += visitorNum1 + " + " + visitorNum2 + " = " + parseFloat(visitorNum1+visitorNum2);
document.write(message);
What's happening here is:
var message = "Hello ";
var name = "Kenny";
Use this operator to append a variable: +=
message += name;
document.write(message);
//Hello Kenny
When concatenating a string and you're wanting to add a variable, call the variable without strings. Remember to add a "dummy" space between variables unless you want them smashed together.
var lastName = "Dubroff";
//message was "Hello Kenny" from before
message += lastName
document.write(message);
//Hello KennyDubroff
//notice the dummy space after hello and the dummy space between name and lastName
message = "Hello " + name + " " + lastName;
document.write(message);
//Hello Kenny Dubroff
3 Answers
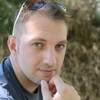
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 Pointsalert("lets do some math!");
var num1 = parseFloat(prompt("please give us your first number"));
var num2 = parseFloat(prompt("please give us your second number"));
var message =
`
<h1> Lets do some math using ${num1} and ${num2} </h1>
Addition ${num1} + ${num2} = ${num1 + num2}<br>
multiplication ${num1} x ${num2} = ${num1 * num2}<br>
division ${num1} / ${num2} = ${num1 / num2}<br>
subtraction ${num1} - ${num2} = ${num1 - num2}
`
document.write(message);
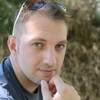
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 PointsThe above method is using template literals just to make it easier to write and read. Notice how my message is encased in backticks.
this makes it a lot easier than trying to concatenate everything.
heres a quick intro to how that works.

Reza Zandi
Full Stack JavaScript Techdegree Student 1,599 PointsHey guys, just adding my code. reading the other codes, I really liked Jesse's code. I didn't think of creating a default message and simply concatinating the math operations in. This would of saved me having to create 4 different variables for each operation. I didn't know how to to a line break, so I did multiple documents.write statements, but I didn't need to do that.
alert("Please enter two numbers and you will see 4 different calculations performed on them");
var number1 = parseInt(prompt("Please enter the first number and make sure it is larger than the second number"));
var number2 = parseInt(prompt("Please enter the second number"));
var message = "<h1> We will perform four math operations on numbers " + number1+ " and " + number2 + "</h1>"
var addition = "<p> Addition: " + number1 + " + " + number2 + "=" +(number1 +number2) + "</p>"; var subtraction = "<p> var Subtraction: " + number1 + " - " + number2 + "=" + (number1-number2) + "</p>"; var multiplication = "<p>
var Multiplication: " + number1 + " * " + number2 + "=" + (number1*number2) + "</p>"; var division = "<p>
var Division : " + number1 + " / " + number2 + "=" + (number1-number2) + "</p>";
document.write(message);
document.write(addition);
document.write(subtraction);
document.write(multiplication);
document.write(division);
'''
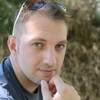
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 PointsHi Reza , nice code my suggestion would be to use parseFloat
rather than parseInt, this will allow people to enter numbers with decimals or whole numbers 3x2 would work in your code but 3.5 x 2.1 wouldnt.
Also for this particular example, you dont need to specify for the first number to be larger than the second as its just basic math questions this would however be important for your random number generation if you dont write code that checks which is the larger number of the 2 when providing a range.
Last small thing which is also not a real issue but best practice, when naming your variables generally start with lower case to allow for camelCase, it also helps with keeping the flow of work the same( again not a big issue but better to get used to early on) your variables are named addition Multiplication and Division. If you always start with lower case, you are less likely to create 2 variables with the same name but different starting case which javascript will see as 2 different things.
Multiplication !== multiplication.
otherwise looks good.

Reza Zandi
Full Stack JavaScript Techdegree Student 1,599 PointsHey Thx for the feedback Doron.Yeah the parseFloat makes sense. Yeah, I did actually name it with lower case, but somehow when I moved it over, things didn't copy paste correctly. The var name is multiplication, but I printed a Multiplication in the string, not sure why var is next to it though.
Jesse Vorvick
6,047 PointsJesse Vorvick
6,047 PointsI am learning this stuff for the first time myself. Perhaps you could use the markdown function of this website to post your code in a more legible format? I clicked the Markdown Cheatsheet link at the bottom of this thread, but it doesn't seem to work. I found out how to do it here:
https://teamtreehouse.com/community/-markdown-cheatsheet-not-working-for-me-when-i-try-to-post-code
Anyway, here is my solution. Repost your solution using the information in the URL above and we can compare!