Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial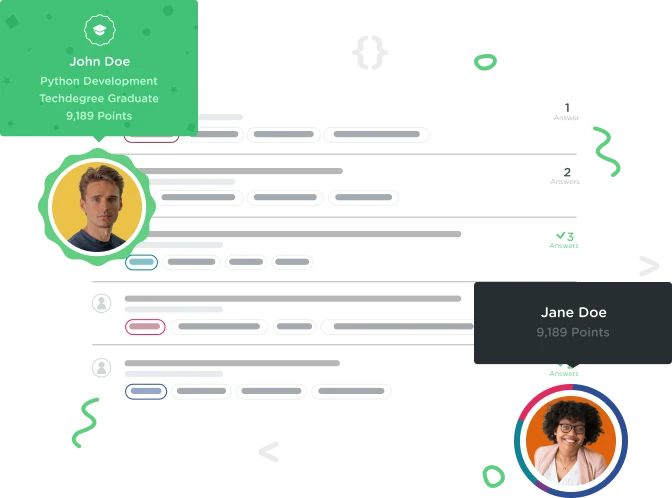
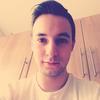
Samuel Catchpole-Radford
8,081 PointsFile import works BUT the random word chosen is only 1 letter??
I'm trying to use the file import, it seems to work however when playing the game the randomly selected word is only 1 letter. What am i doing wrong?
Note: the words.txt file I am using has all the words in separated onto single lines.
Code below:
import sys
import os
import random
file = open('words.txt', 'r')
words = file.read()
#words = [
# 'hotel',
# 'holiday',
# 'sun',
# 'banana',
# 'yummy',
# 'mummy',
# 'anything',
# 'anytime',
# 'random',
# 'time',
# 'curtain',
# 'door',
# 'silly',
# 'agatha',
# 'sam',
# 'baby',
# 'awesome',
# 'average',
# 'science',
# 'degree',
# 'pizza'
#]
def clear():
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
def draw(incorrect_guesses, correct_guesses, random_word):
clear()
print("####################")
print("Guesses: {}/7".format(len(incorrect_guesses)))
print('')
for letter in incorrect_guesses:
print(letter, end=' ')
print('')
print("####################")
print('')
for letter in random_word:
if letter in correct_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
def get_guess(incorrect_guesses, correct_guesses):
while True:
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess a single letter, try again")
elif guess in correct_guesses or guess in incorrect_guesses:
print("You have already guessed that letter, try again")
elif not guess.isalpha():
print("You can only guess letters, try again")
else:
return guess
def play(done):
clear()
random_word = random.choice(words)
correct_guesses = []
incorrect_guesses = []
while True:
draw(incorrect_guesses, correct_guesses, random_word)
guess = get_guess(incorrect_guesses, correct_guesses)
if guess in random_word:
correct_guesses.append(guess)
found = True
for letter in random_word:
if letter not in correct_guesses:
found = False
if found:
draw(incorrect_guesses, correct_guesses, random_word)
print("####################")
print('')
print("You Win!, The word was {}".format(random_word))
print('')
print("####################")
done = True
else:
incorrect_guesses.append(guess)
if len(incorrect_guesses) == 7:
draw(incorrect_guesses, correct_guesses, random_word)
print("####################")
print('')
print("You Lost!, The word was {}".format(random_word))
print('')
print("####################")
done = True
if done:
play_again = input("Do you want to play again? Y/n ").lower()
if play_again != 'n':
return play(done=False)
else:
print("####################")
print('')
print("Thanks for playing!")
print('')
print("####################")
sys.exit()
def welcome():
start = input("Do you want to start the game? Y/n ")
if start != 'n':
return True
else:
print("####################")
print('')
print("Thanks for playing!")
print('')
print("####################")
sys.exit()
done = False
while True:
clear()
welcome()
play(done)
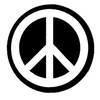
john larson
16,594 PointsIn case anyone else runs into this, using readlines put an extra space at the end of each word making winning the game ...not work properly. I found this fixed that problem.
file = open("words.txt", "r")
words = file.read().splitlines()
1 Answer
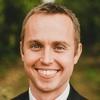
Ryan Oakes
9,787 PointsHey Samuel,
If you check the type (e.g. type(words)) of your words variable after you import the file, you'll notice its a string, not a list. That's causing your problems when you get your random_word.
See if you can figure out how to make sure you import it as a list, not a string! Google is your friend. : )
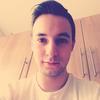
Samuel Catchpole-Radford
8,081 PointsHi Ryan,
Thanks, I have change it from words = file.read() to words = file.readlines() and it works fine :)
Thanks for your help.
john larson
16,594 Pointsjohn larson
16,594 PointsSamuel, I have to say thank you for your post/question. After looking at the documentation I was at a loss. I followed your footsteps and I believe I am headed in the right direction now.