Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial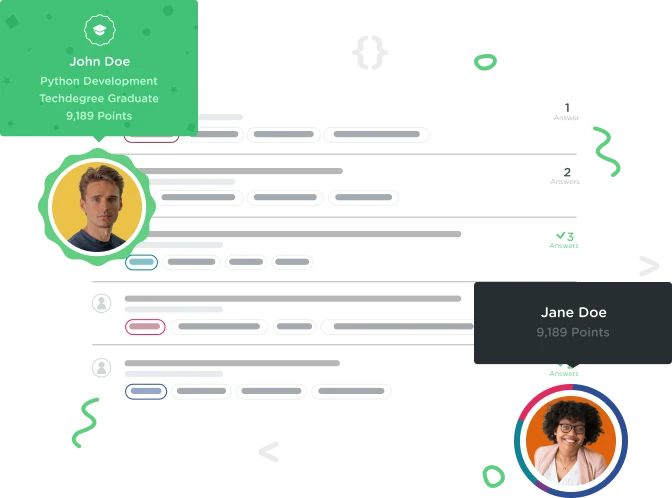
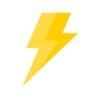
Refael Ozeri
18,274 Pointsfile storage code challenge
can't seem to figure this one out, "The following code is similar to what you just wrote. Modify this code to only write the file if it does not already exist. Hint: Use the method from the File class that checks to see if a file exists or not."
public class FileUtilities {
public static boolean copyResult;
public static void saveAssetImage(Context context, String assetName) {
File fileToWrite = new File(context.getFilesDir(), assetName);
AssetManager assetManager = context.getAssets();
try {
InputStream in = assetManager.open(assetName);
FileOutputStream out = new FileOutputStream(fileToWrite);
copyResult = copyFile(in, out);
} catch(FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
private static boolean copyFile(InputStream in, FileOutputStream out) {
// Copy magic intentionally omitted
return true;
}
}
what am I missing here?
4 Answers
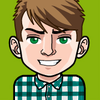
Harry James
14,780 PointsGlad to hear you got it fixed!
I'll mark this post as resolved now so that anyone else browsing this topic will know that this question has been answered :)
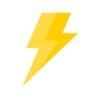
Refael Ozeri
18,274 PointsGood idea, thank you :)

Anthony Attard
43,915 PointsA more concise option.
if (! fileToWrite.exists()) { copyResult = copyFile(in, out); }
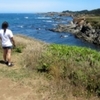
Nancy Melucci
Courses Plus Student 36,158 PointsIf anyone is out there, the code posted above is NOT working for me. I am getting this error:
./FileUtilities.java:28: error: cannot find symbol if (fileToWrite.exists() == false) { copyResult = copyFile(in, out); } ^ symbol: variable fileToWrite location: class FileUtilities 1 error
Posting my code below.
public class FileUtilities {
public static boolean copyResult;
public static void saveAssetImage(Context context, String assetName) {
File fileToWrite = new File(context.getFilesDir(), assetName);
AssetManager assetManager = context.getAssets();
try {
InputStream in = assetManager.open(assetName);
FileOutputStream out = new FileOutputStream(fileToWrite);
copyResult = copyFile(in, out);
} catch(FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
private static boolean copyFile(InputStream in, FileOutputStream out) {
// Copy magic intentionally omitted
byte[] buffer = new byte[1024];
int read;
if (fileToWrite.exists() == false) { copyResult = copyFile(in, out); }
}
}
Thanks.
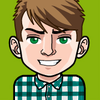
Harry James
14,780 PointsHey Nancy!
fileToWrite is out of scope in that method, meaning you're unable to access it. Therefore, we need a different method of only running the copyFile() method when the fileToWrite does not exist.
Hint: Take a look at where you're calling the method from - can you run some sort of check to only call the method when this condition passes?

Alwin Lazar V
5,267 PointsI have solved and it works for me
public class FileUtilities {
public static boolean copyResult;
public static void saveAssetImage(Context context, String assetName) {
File fileToWrite = new File(context.getFilesDir(), assetName);
AssetManager assetManager = context.getAssets();
try {
InputStream in = assetManager.open(assetName);
FileOutputStream out = new FileOutputStream(fileToWrite);
// here I changed
if (fileToWrite.exists() == false) { copyResult = copyFile(in, out); }
} catch(FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
private static boolean copyFile(InputStream in, FileOutputStream out) { // Copy magic intentionally omitted return true; } }
Happy coding
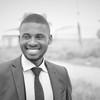
James Chakanyuka
4,916 PointsYou saved me a whole lot of time, Thank You
Refael Ozeri
18,274 PointsRefael Ozeri
18,274 PointsNevermind, solved it!
if anyone else gets stuck in this one, the answer is :
if (fileToWrite.exists() == false) { copyResult = copyFile(in, out); }