Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial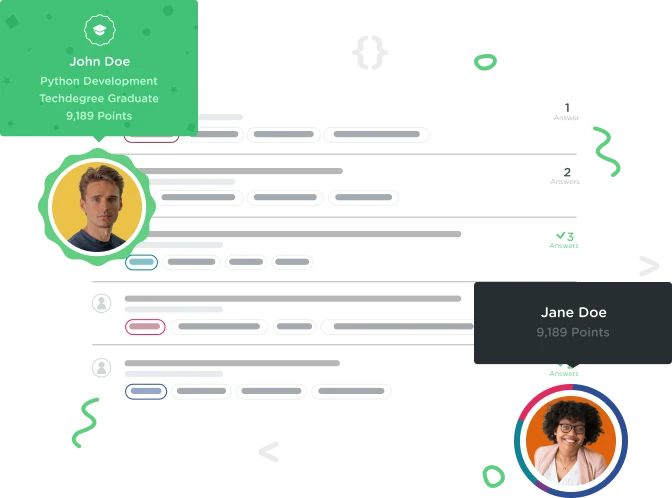

cbob
3,233 PointsFill in the find_index
I'm not sure what i'm doing wrong, can someone please explain?
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def find_index(name)
index = 0
found = false
@todo_items.each do |todo_item|
if todo_item == name
found = true
end
if found
break
else
index += 1
end
if found
return true
else
return nil
end
end
end
end
4 Answers
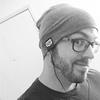
Jesse James
6,079 PointsI'm about to head to bed (but I'll check-in in the morning at work) but I think the culprit may be that you have the final "if...else" inside the @todolist.each iteration. Additionally, you might be able to combine a bit of the code like this:
def find_index(name)
index = 0
found = nil
@todo_items.each do |todo_item|
if todo_item == name
found = true
break
else
index += 1
end
end
found
end
If I'm reading what you're going for correctly, this will iterate through the @todo_items and if the todo_item == name then it will assign true to found and then break out of the loop. If it does not, it will increment the index by 1. After the loop breaks due to a true value or runs todo_items.length number of times, it will return the value of found which would either be true or nil, depending on if any iteration of the loop was found to be true.
Make sense? Give it a whirl and let us know what you find/get!
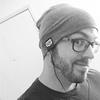
Jesse James
6,079 PointsAhhh! I just read the challenge and it actually wants you to return the index, if it was found. So in this case you'd want it to look like this (maybe lol):
def find_index(name)
index = 0
found = nil
@todo_items.each do |todo_item|
if todo_item == name
found = true
break
else
index += 1
end
end
if found == true
index
else
nil
end
end

cbob
3,233 Pointsit doesn't seem to be working for me, any suggestions? Thank you

nlitwin
3,505 PointsTry this: if todo_item.name == name