Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial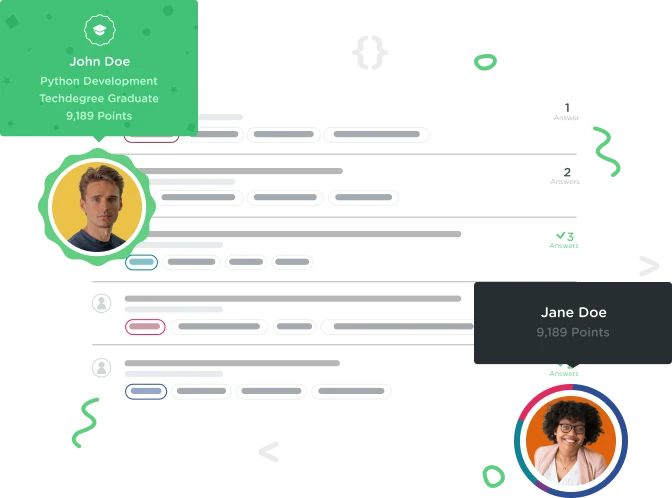
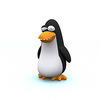
Jan Oberreiter
78,020 Pointsfilter and map in JS
Could someone help me on this Challenge. The question is: Using the filter and map methods on the years array, create an array of display strings for each year in the 21st century (remember: the 21st century starts with the year "2001"). Each display string should be the year followed by "A.D." See the comments below to see the correct result. Store the new array in the variable displayYears. I don't know, what I made wrong. Thanks everyone for help, regards, Jan.
const years = [1989, 2015, 2000, 1999, 2013, 1973, 2012];
let displayYears;
const displayYears = years
.filter(year => year > 2000)
.map(year => year + 'A.D.');
// displayYears should be: ["2015 A.D.", "2013 A.D.", "2012 A.D."]
// Write your code below
6 Answers

Cooper Runstein
11,850 PointsYou declared the same variable "displayYears" twice, once with const, once with let. Also, you'll likely need a space to separate year and "A.D.".
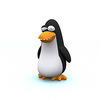
Jan Oberreiter
78,020 Pointsthx Cooper ...

Imre Marton
Full Stack JavaScript Techdegree Graduate 33,928 PointsdisplayYears = years .filter(year => year > 2000) .map(year => year + " A.D.");

Kate C
13,589 PointsWhy can't I use displayYears = years .filter(year => year > 2000) .map(year => year.join("A.D."); console says no such function, why?

Henry Blandon
Full Stack JavaScript Techdegree Graduate 21,521 PointsHere is another way using templet literal.
displayYears = years
.filter(year=> year>2000)
.map(year=> `${year} A.D.`);

Fabricio Policarpo
8,674 PointsdisplayYears = years.filter(yr => yr > 2000).map(yr => ${yr} A.D
);
I did it like this, unfortunately, I have found that code challenges tend to give errors when using backticks.

Darshen Patel
Front End Web Development Techdegree Student 18,928 PointsNoticed the same issue.

Henry Blandon
Full Stack JavaScript Techdegree Graduate 21,521 PointsLewis Sheeran the expression I wrote is using back ticks which it converts the output to a string. It is called Template Literals. If you want to see the type it is returning, just change the code like this.
displayYears = years
.filter(year => year > 2000)
.map(year => typeof (`${year} A.D.`));
console.log(displayYears);
The return should look like:
[ 'string', 'string', 'string']

Lewis Sheeran
Web Development Techdegree Graduate 19,398 PointsCan someone please explain to me why we aren't calling .toString within the map method? How do the years get converted to a string format when the filter initially places the values in the new array as integers?
The solutions posted above work (thanks) but I still don't understand how the year values themselves are automatically becoming strings.
Jan Oberreiter
78,020 PointsJan Oberreiter
78,020 PointsI just managed it myself by having a closer look at the error message, first I declared "displayYears" twice ... and the 'A.D.' ... I forgot a space when concatenating it ... so by dropping the "const" in front of "displayYears" and by writing ' A.D.' ... instead of 'A.D.' ... everything works out fine ... but if I wouldn't have posted my question, I guess I would not have tried harder, but I didn't want to look like an idiot ... so I can just encourage anyone to ask questions ...