Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial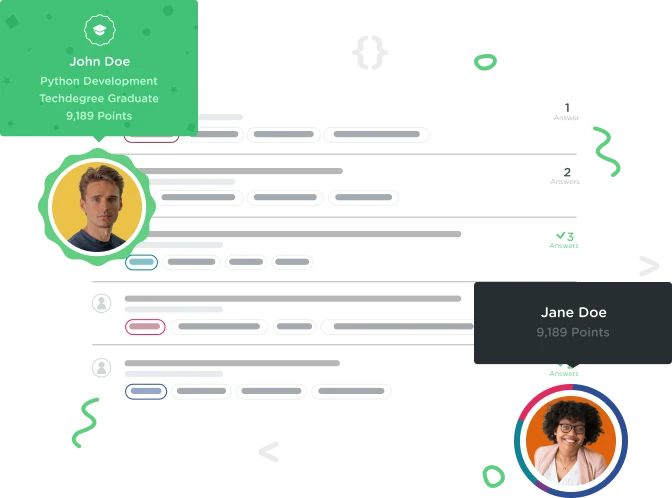

Andrew Walters
8,876 PointsFinal Challenge PHP OOP
This is my code and the question is asking me to add a getInfo method which will return the common_name/flavor/record_weight/and species properties for the Trout class. I am doing exactly that; however, I am getting the try again error. I even tried using the same styling as the prewritten $output variable and still get the bummer. I am truly stuck and would like some help. Also, can anyone provide good documentation for PHP OOP? Other than the manual? It's so technical that a portion of it feels lost on me. Thanks!
<?php
class Fish
{
public $common_name;
public $flavor;
public $record_weight;
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo() {
$output = "The {$this->common_name} is an awesome fish. ";
$output .= "It is very {$this->flavor} when eaten. ";
$output .= "Currently the world record {$this->common_name} weighed {$this->record_weight}.";
return $output;
}
}
class Trout extends Fish {
public $species;
function __construct($name, $flavor, $record,$species){
parent::__construct($name, $flavor, $record);
$this->species = $species;
}
$brook_trout = new Trout("Trout", "Delicious", "14 pounds 8 ounces", "Brook");
public function getInfo(){
return {self::common_name}.{self::flavor}.{self::record_weight}.{self::species};
}
}
?>

Andrew Walters
8,876 PointsSo I ended up solving my problem by rewritting my code from scratch. It went through on the first try. While I couldn't identify exactly what was causing the issue, I do know that it was most likely syntax error where I was perhaps misplacing the getInfo method (as in above or below a curly brace) or perhaps the slightest extra key somewhere I was completely blind to. Either way, let this be a lesson to those out there doing the challenges. If you are failing the challenges but you know that you're code is right, then check your syntax from top to bottom - and it never hurts to take a break so that you can return with fresh eyes. Thanks everyone!
2 Answers

Joe Hartman
20,881 PointsHey, glad you figured it out. One of my favorite resources is W3Schools. They have tons of stuff on web and programming languages in addition to PHP: HTML, CSS, Javascript, etc. Their explanations are just as comprehensive but written more simply and clearly.

masterpowers
3,061 Pointsclass Trout extends Fish {
public $species;
function __construct($name, $flavor, $record,$species){
parent::__construct($name, $flavor, $record);
$this->species = $species;
}
public function getInfo(){
return $this->common_name . $this->flavor . $this->record_weight . $this->species;
}
} // end of Trout Class
$brook_trout = new Trout("Trout", "Delicious", "14 pounds 8 ounces", "Brook");
//This should be Outside Your Class when you are doing a new Object Instance
echo $brook_trout->getInfo();
//Call the Function
Andrew Walters
8,876 PointsAndrew Walters
8,876 Pointsself::* was a mistake, I'm using $this->common_name...etc to return the variables, and the curly braces were just something that I was trying in the moment. Thanks