Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial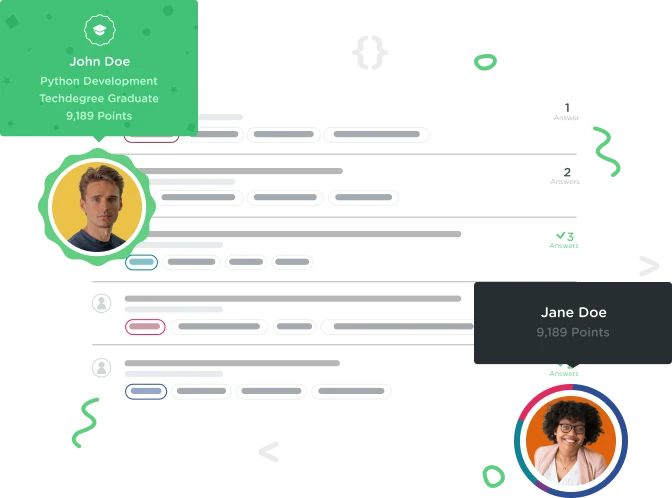
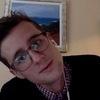
Patrick Mockridge
Full Stack JavaScript Techdegree Graduate 45,611 PointsFinal Challenge: What's wrong with my code here?
I'm being told that I'm not printing the hash values to the screen. Can anyone tell me what I'm doing wrong with this code?
contact_list = [
{"name" => "Jason", "phone_number" => "123"},
{"name" => "Nick", "phone_number" => "456"}
]
contact_list.each do |i|
i.each do |j , k|
print "#{k}"
end
end
1 Answer
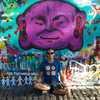
Andrew Stelmach
12,583 PointsStrange one this. Your code works if you use puts instead of print:
contact_list = [
{"name" => "Jason", "phone_number" => "123"},
{"name" => "Nick", "phone_number" => "456"}
]
contact_list.each do |i|
i.each do |j , k|
puts "#{k}"
end
end
Why? I don't know, but I'd like to find out. I'll have a little google.
In the meantime, I think this code is a bit better like this:
contact_list = [
{"name" => "Jason", "phone_number" => "123"},
{"name" => "Nick", "phone_number" => "456"}
]
contact_list.each do |hash|
hash.each{ |key, value| puts "#{value}" }
end
Ok, actually I wasn't looking closely enough at my terminal when I was experimenting. Your code actually performs as expected. It returns:
Jason123Nick456 => [{"name" => "Jason", "phone_number" => "123"}, {"name" => "Nick", "phone_number" => "456"}]
See, it prints out the values as expected and then returns the whole array. I'm not sure why it returns the whole array, but it's probably not all that important for this exercise, and puts
also returns the whole array, except that puts adds a new line after each value. You can google for further reading. Using puts
returns:
Jason
123
Nick
456
=> [{"name" => "Jason", "phone_number" => "123"}, {"name" => "Nick", "phone_number" => "456"}]
I expect that would probably pass the test.
EDIT: You actually need to use |contact|
instead of |hash|
in the challenge. But anyway, it ACTUALLY turns out that this doesn't pass the test, even though it returns the exact same result as what they want to be returned. They probably need to amend this.
To pass the test, you have to specifically declare "name" and "phone_number" even though, in this example, it's not necessary to get the required output:
contact_list.each do |contact|
puts contact["name"]
puts contact["phone_number"]
end
It is more readable and understandable though, to be fair, which is important.
fngr
11,179 Pointsfngr
11,179 PointsI tried this in the workspace and it works fine, but that didn't passes.
but your last code
let me pass. Don't know why.