Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial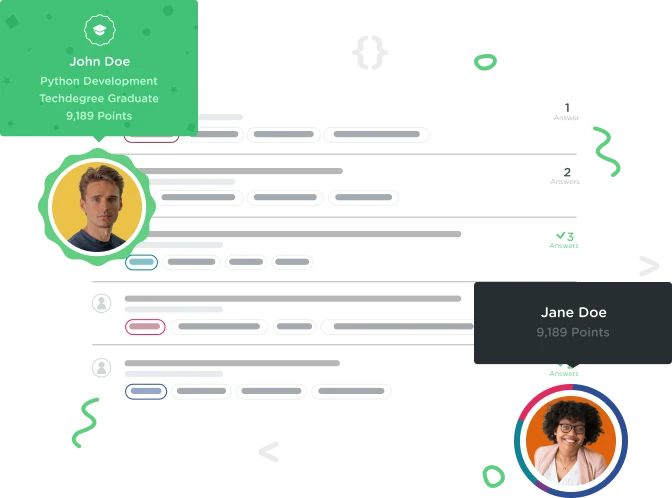
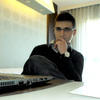
Daniel Nitu
13,272 PointsFinal code example
This is my code for the Title Case script. I added an array with words that should be in lowercase (unless it's the first word). If you have a better way of doing this, please let me know.
var lowercase = ['the', 'a', 'an', 'and', 'but', 'or', 'on', 'in', 'with', 'to', 'at', 'under', 'near', 'for'];
function titleCase(title) {
var words = title.split(' ');
var titleCaseWords = words.map(function(word) {
return word[0].toUpperCase() + word.substring(1).toLowerCase();
});
for(var i = 1; i < titleCaseWords.length; i++) {
for(var j = 0; j < lowercase.length; j++) {
if(titleCaseWords[i].toLowerCase() === lowercase[j]) {
titleCaseWords[i] = titleCaseWords[i].toLowerCase();
}
}
}
console.log(titleCaseWords.join(' '));
}
titleCase('the CALL fOR help At the airPORt');
3 Answers

Adam Fields
Full Stack JavaScript Techdegree Graduate 37,838 PointsNice job handling the lowercase words.
Here's my one liner (just for titlecasing every word):
const titleCase = title => title.toLowerCase().split(' ').map(word => `${word[0].toUpperCase()}${word.substring(1)}`).join(' ');

Caroline olijve
Courses Plus Student 14,499 PointsNice, thanks for the post, I also did the array for connection-words but instead of the loop I used an if-else statement
var expect = require('chai').expect
function titleCase(title){
connectionWords =["of", "and", "from", "in", "on", "a", "the"]
words = title.split(" ")
titleCasedWords = words.map(function(word, index){
if (connectionWords.includes(word.toLowerCase())
&&
index!=0)
{
return word.toLowerCase()
} else {
return word[0].toUpperCase() + word.substring(1).toLowerCase()
}
})
return titleCasedWords.join(" ")
}
expect(titleCase('the towers of the city of London')).to.equal('The Towers of the City of London')
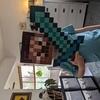
Gabbie Metheny
33,778 PointsNice examples! For mine, I added some logic to uppercase the connector words if they're at the beginning of the title ('i.e. The Squid and the Whale') by adding the index
parameter to the map()
call.
const {expect} = require('chai');
const connectorWords = ['a', 'an', 'and', 'at', 'but', 'for', 'in', 'near', 'on', 'or', 'the', 'to', 'under'];
function titleCase(title) {
// lowercase entire title and split into words
const words = title.toLowerCase().split(' ');
// include index parameter in map()
const titleCasedWords = words.map((word, index) => {
// if word isn't in connectorWords array OR it's the first word in the title
if (!connectorWords.includes(word) ||
index === 0) {
// uppercase the first letter
word = word[0].toUpperCase() + word.substring(1);
}
return word;
});
return titleCasedWords.join(' ');
}
expect(titleCase('the great mouse detective')).to.be.a('string');
expect(titleCase('a')).to.equal('A');
expect(titleCase('vertigo')).to.equal('Vertigo');
expect(titleCase('the great mouse detective')).to.equal('The Great Mouse Detective');
// unit test for connector words
expect(titleCase('marley and me')).to.equal('Marley and Me');
// unit test for connector words at beginning of title
expect(titleCase('the squid and the whale')).to.equal('The Squid and the Whale');