Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial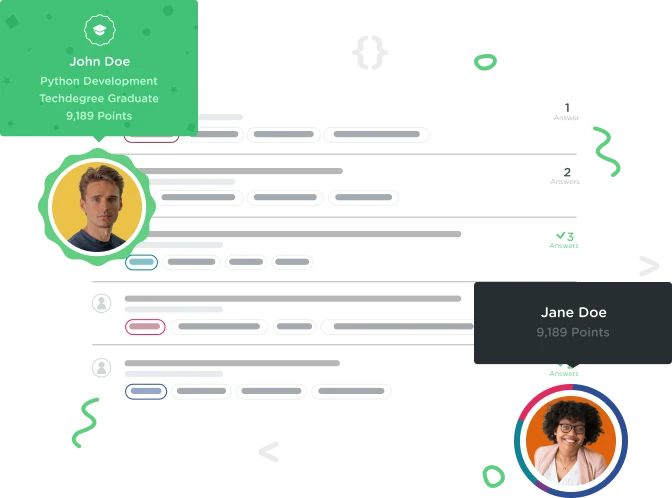

Jo-ann de la Mare
1,036 Pointsfinal code not working with text entry to total or number of people
I got to the end of the module, then ran the code using text to answer the total value, I now get the console display reading
Oh no that's not a valid value, please try again (could not convert string to float: twenty)
why is it doing this?
import math def split_check(bill_total, little_piggies): if little_piggies <=1: raise ValueError("more than one person is required") return math.ceil(bill_total / little_piggies) try: bill_total = float(input("What is the total amount of the bill? ")) little_piggies = int(input("How many people were there? ")) amount_due = split_check(bill_total, little_piggies) except ValueError as more_people: print("oh no that's not a valid value") print("{}.".format(more_people)) else: print("each little piggy owes £{}".format(amount_due))
I'm pretty sure I followed the lesson correctly, thank you

Jo-ann de la Mare
1,036 PointsOk, so after lots of google (and days of tears from me) I found the solution. I should point out that I have Autism so sometimes I struggle to explain myself to other people (and understand when other explain things to me) as what makes sense to me is confusing to a lot people.
so during the module we adjusted the code so that if the user entered word not numbers when asked "how many people were there?" it wouldn't show the traceback message and would display a message saying something like "oh dear, thats not a valid number let's try again" and would start the program again.
That worked fine and I continued the module which then went on to raise the ValueError if the number of people was equal to or less than 1. We did this by raising the ValueError and passing that our unique message "more than one person is required" I understood that and it worked fine when I caused that specific error when checking the code.
When I had got to the end of the module I then ran the code again as a double check to see what happens when the user causes all the errors the module had covered, i.e. entering the number of people or total amount of the bill in words or and entering 1 or 0 as the number of people. I tried entering the total amount due as twenty not 20 as Craig had done earlier in the module, the script ran but gave the following
Oh no that's not a valid value, please try again (could not convert string to float: twenty)
I spent hours trying to work out what I'd done wrong, and hours crying about it. (The same when I got to the masterticket example at the end of the course) I'd done everything Craig had told me but now it wasn't working and I didn't know why.
I eventually (Friday) worked out that I needed to add an "if" block to my except block except ValueError as more_people: if "invalid" in more_people: print("Oh no that's not a valid value please try again") else: print("oh no that's not a valid value") print("{}.".format(more_people)) else: print("each little piggy owes £{}".format(amount_due))
It produced even more tracebacks telling me that more_people wasn't iterable (still unsure what that is exactly) then yesterday I worked out it needed to be:
if "invalid" in str(more_people):
I think I've explained it, I now have the code working and I think I understand why/how even if I've not explained well. I guess the point of this comment is to say (politely of course) that it would have been a huge help if things like this are explained or even referred to in the module or teachers notes, even just something like "now this may throw up another issue but we'll cover that later/check the teachers notes for ways to fix it"
2 Answers
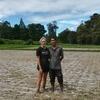
Scott Bailey
13,190 PointsWould you be able to put your code up?

Jo-ann de la Mare
1,036 Pointshere is the code I wrote from the module on raising errors, I thought I'd copied it right (I've not used great English in it as it's just to learn)
import math def split_check(bill_total, little_piggies): if little_piggies <=1: raise ValueError("more than one person is required") return math.ceil(bill_total / little_piggies) try: bill_total = float(input("What is the total amount of the bill? ")) little_piggies = int(input("How many people were there? ")) amount_due = split_check(bill_total, little_piggies) except ValueError as more_people: print("oh no that's not a valid value") print("{}.".format(more_people)) else: print("each little piggy owes £{}".format(amount_due))
I'm pretty sure I followed the lesson correctly, thank you
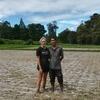
Scott Bailey
13,190 Pointsbill_total = float(input("What is the total amount of the bill? "))
You said you put in "twenty" - python can't convert this string to a floating number.
The way python works in the example you have to use an actual number (int) - ie 20
When you type this for the input it's still a string - but because it's a valid int you can convert it to a float (which you do).
There will be ways that allow you to type "twenty" but that's a course for another time!
Aaron Jorgensen
Python Development Techdegree Graduate 13,658 PointsAaron Jorgensen
Python Development Techdegree Graduate 13,658 PointsCan you post the code here?