Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial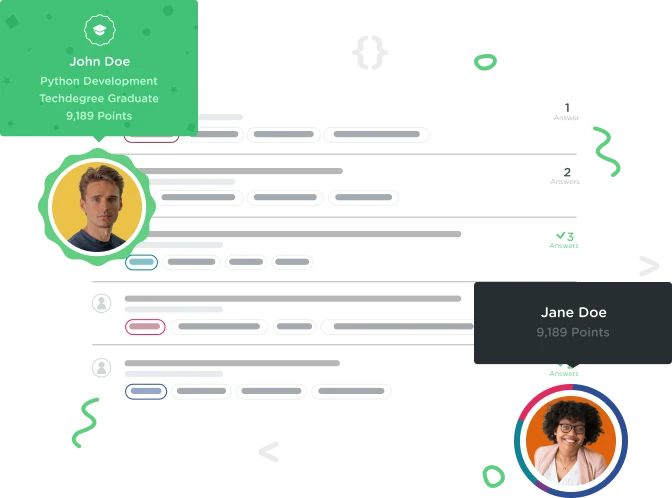

JASON GALWEY
722 PointsFinally, add a # symbol and lastName in uppercase to the end of the userName string. The final value of userName is "
need help finishing this of Ive tried all possible scenarios I can think off
var id = "23188xtr";
var lastName = "Smith";
var userName = id.toUpperCase() += lastName.toUpperCase();
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
1 Answer
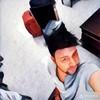
Ari Misha
19,323 PointsHiya Jason! You literally have to add a "#" between upper cased "id" and uppercased "lastName" variable. Here is my code :
var id = "23188xtr";
var lastName = "Smith";
var userName = id.toUpperCase(); //task 1
userName = id.toUpperCase() + "#" + lastName.toUpperCase(); //task 2
//"userName" outputs "23188XTR#SMITH"
saber
13,276 Pointssaber
13,276 PointsHi Jason,
I think I can help you out. I believe your issue lies with the += operator you're using. += is an assignment operator. In other words, JavaScript is expecting whatever value is to the right of += to be assigned to whatever is left of the +=. Whatever is to the left of an assignment operator, must be a variable. So in other words, what's happening above is you're unintentionally assigning the value of lastName.toUpperCase(); to id.toUpperCase(). That will totally cause problems since id.toUpperCase() is a method call on a string and not a variable where you can store things.
One way to solve this is by using concatenation by just using a + instead of a += like this:
var userName = id.toUpperCase() + "#" + lastName.toUpperCase();
This will concatenate id, the # and lastname into a string and assign it to userName.
Alternatively, if you'd like to continue using the assignment operator +=, you just have to do it one piece at a time so that the variable userName is always on the left of the +=.
Like this: