Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial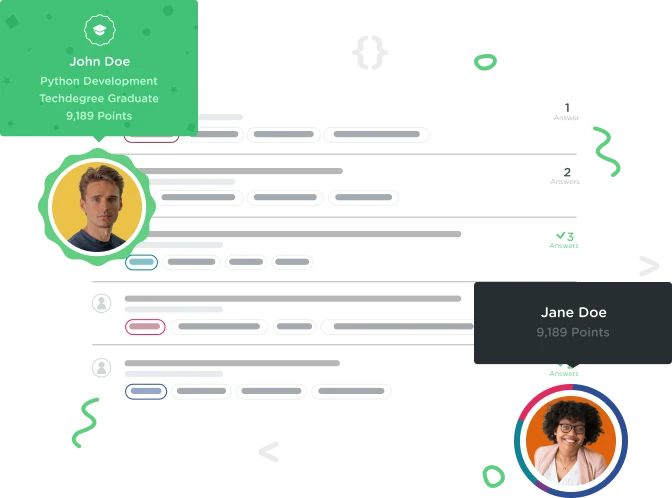

Erik Nuber
20,629 PointsFinally got Extra Credit code done. Here it is...
Was determined to figure this out so here is the code I used and, it works.
first my student list
var students = [
{name: "Ramon", track: "iOS Development With Swift", achievements: 175, points: 16375},
{name: "Michael", track:"Front End Development", achievements: 158, points: 16375},
{name: "Ramon", track:"PHP Development", achievements: 25, points: 2250},
{name: "Kenny", track:"Learn WordPress", achievements: 40, points: 1950},
{name: "Jacob", track:"Rails Development", achievements: 5, points: 350}
];
Here is the rest, I will break it down into smaller areas so I can explain.
Here are my variables and the print function. I added in an empty array which will be used to store the index numbers of all the students that match a specific name.
var message = "";
var student;
var search;
var listOfStudents = [ ];
function print(message) {
var xDiv = document.getElementById("output");
xDiv.innerHTML = message;
}
here is my function to create a report of anyone captured, whether they have the same name or not. Here I had to create a new variable that will store a completed list. I also created a variable called position that stores the index number from the array listOfStudents. This is than used to access the array students that is the actual list of names.
function getStudentReport(student) {
var finalList;
for (var j = 0; j < listOfStudents.length; j++) {
var position = listOfStudents[j];
var report = "<h2>Student: " + students[position].name + "</h2>";
report += "<p>Track: " + students[position].track + "</p>";
report += "<p>Achievments: " + students[position].achievements + "</p>";
report += "<p>Points: " + students[position].points + "</p>";
finalList += report;
}
return finalList;
}
Here is the most involved area which took me some time to figure out. I used console.log() to try an catch my errors so I could figure out what was actually happening as, more often than not, nothing happened at all....
while (true) {
search = prompt('Search student records: type a name (or type "quit" to end)');
search = search.toLowerCase();
var notInList = false;
if (search === "quit" || search === null) {
break;
}
for (var i = 0; i < students.length; i++) {
student = students[i];
if (student.name.toLowerCase() === search) {
listOfStudents.push(i);
notInList = true;
}
if ((i + 1) == students.length) {
message = getStudentReport(listOfStudents);
print(message);
}
}
if (!notInList) {
message = ("<p> The student " + search + " is not on the list.</p>");
print(message);
}
}
The notInList variable is used to determine whether or not a student is actually in the list being search. In the first if statement, if the names are a match, we than push the variable "i" that actually indicates the index number in the list of students. As it is a match, we also have to make sure that the notInList is set to true so that the message stating the student is not on the list won't print.
Next, the code tests to see if there are still more students to search for before actually calling the print function. If there are no more students, it goes ahead and sends the array of index numbers to the print function as explained above.
Hope this helps if you are stuck.
3 Answers
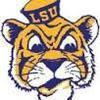
Cena Mayo
55,236 PointsThis is really awesome, Erik. Not only for your own education, but that you showed your code, and also took the time to explain what each piece was doing. That shows real mastery of the material.
Super impressed (not just by the code, but the explanation and the thoughtfulness that went into it.) Great job, and hope to see more posts like this from you in the future!
Best, Cena

Allen Liff
5,003 PointsThanks! I was stumped and this helped. And now for one small tweak...
I kept getting an "undefined" at the beginning of the student roster. I went into the getStudentReport function: I changed :
var finalList;
to:
var finalList = '';
That did the trick.

Erik Nuber
20,629 PointsTo answer PabloAguilera, sorry for the delay, I get lots of messages from Treehouse and missed this one.
I used the variable notInList variable simply for my own naming convention. I realize the statement if read with the ! mark makes no sense. Not Not In List which with the two Not's negates it. However, I prefer reading it as to what it is actually being used for while ignoring the !. You can name it however eases your own mind which is the great part of variables. Whatever makes you most comfortable. The way you named it actually makes more sense if read properly.
pabloaguilera
4,196 Pointspabloaguilera
4,196 PointsHello I'm kinda confused with this variable " notInList". Did you mean to say " onTheList" instead?
I changed that variale to - "onTheList" and that makes more sense to me. For example:
on line 54, Andrew is obviously not on the list, so (!onTheList) = not on the list. I might be wrong, a clarification (from anyone else too) is greatly appreciated.
thanks.