Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial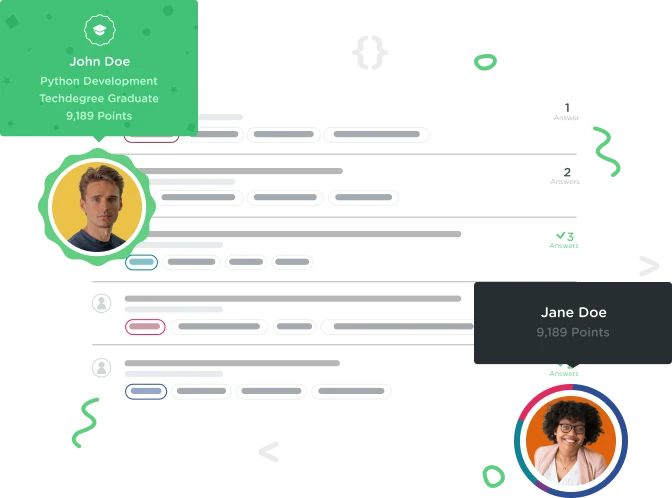

Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 PointsFind the most common words, any hints and suggestions are welcome thank you for your help.
There is an error in my code, and I don't know what it is:
Task:
Print to console the first three most common words that appear in a given text
Feedback Hint: you can use the built-in function for sorting and slicing arrays.
Checks Custom TestIncomplete Find the 3 most common words
JS CODE:
// This script will run when the page is loaded window.onload = () => { let result = countWords(); console.log(result); };
function countWords() { // The text variable contains the long string of words let text = "Some of the biggest and most expensive transportation projects in the world have involved building bridges. Bridges are crucial links that carry cars, trucks and trains across bodies of water, mountain gorges or other roads. As a result, they are one of the most important aspects of civil engineering and are subject to intense scrutiny, especially when they collapse. Bridge collapses can be tragic events, leading to loss of life and serious property damage. That is why bridge engineers, designers and builders must always take their jobs very seriously. The best way for them to prevent these accidents is to understand why bridges collapse in the first place. Understanding bridge collapses can lead to major changes in the design, construction and safety of future building projects. The following are main reasons why bridges fall.";
// TODO: add code here to remove the periods and commas and transform all words to lowercase letters text = text.replace(/[.,]/g, ""); text = text.toLocaleLowerCase(); const wordArray = text.split(" "); console.log(wordArray); // The wordArray array will contains a key value pair for words and their occurences in the text const wordArray = text.split(" ");
// This loop goes through the wordArray and creates the key value pair objects const wordCount = {}; wordArray.forEach((item) => { if (wordCount[item] == null) wordCount[item] = 1; else { wordCount[item] += 1; } }); // turn objects into an array of arrays let myArray = Object.entries(wordCount);
// Turn objects into an array of arrays let myArray = Object.entries(wordCount);
// TODO: sort the arrays based on the count number and store the result in a variable called bArray let bArray = myArray .sort((a, b) => (a[1] < b[1] ? 1 : a[1] > b[1] ? -1 : 0)) .slice(0, 3); // return the first three most common words. return bArray; }
//don't change this line if (typeof module !== "undefined") { module.exports = countWords; }
ERRORS:
FAIL ./nt-test.js ● Test suite failed to run
SyntaxError: /home/nt-user/workspace/wordcount.js: Identifier 'wordArray' has already been declared (18:8)
16 | console.log(wordArray);
17 | // The wordArray array will contains a key value pair for words and their occurences in the text
> 18 | const wordArray = text.split(" ");
| ^
19 |
20 | // This loop goes through the wordArray and creates the key value pair objects
21 | const wordCount = {};
at Parser._raise (../../../usr/local/lib/node_modules/jest/node_modules/@babel/parser/src/parser/error.js:60:45)
Test Suites: 1 failed, 1 total Tests: 0 total Snapshots: 0 total Time: 8.557 s Ran all test suites matching /nt-test.js/i. (node:1295) ExperimentalWarning: The fs.promises API is experimental
import countWords from './wordcount';
describe('Testing sliceElements', function() { test('should return the top 3 words', () => { let result = countWords(); expect(result.length).toEqual(3) }); test('should return the top 1 word', () => { let result = countWords(); expect(result[0][0]).toEqual("the") }); test('should return the numer 2 word', () => { let result = countWords(); expect(result[1][0]).toEqual("and") }); test('should return the numer 3 word', () => { let result = countWords(); expect(result[2][0]).toEqual("of") }); })
Again thank you in advance for your help