Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial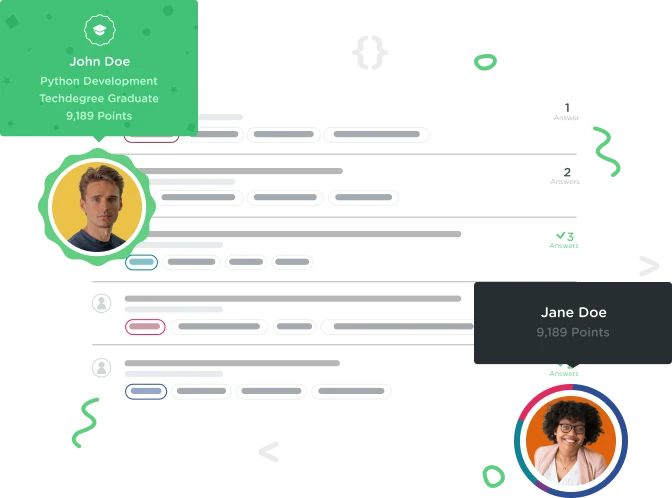
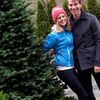
Jennifer Hughes
11,421 PointsFinding average, minimum, and maximum value in an array.
Hi!
Could someone help me solve this problem? It isn't from a Treehouse course, just some outside practice I am doing.
Given an array, create an algorithm, that finds the maximum number in the array, the minimum number in the array, and the average of the numbers in the array.
var myArray = [1, 34, 23, 12, 6, 3];
Initially, I did:
// var myArray = [1, 34, 23, 12, 6, 3]; var min = 0; var max = 0; var total = 0;
for (var i = 0; i <myArray.length; i++) { if (myArray[i] < min) { min = myArray[i]; } else if (myArray[i] > max) { max = myArray[i]; }
total += myArray[i]; }
console.log(max); console.log(min); console.log(total/myArray.length);
//
The problem with the above is that because there is no value less than 0 in the array, the value of min stays 0. However, I want to change the value of min to the smallest number in myArray, that being 1.
Any help is appreciated.
Thanks!!
5 Answers
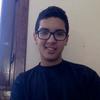
Mohammed Khalil Ait Brahim
9,539 PointsThere is a little tweek you can do to make your algorithm handle negative values (And by the ways if all values were negative you would get same issue with max as it will stay 0)
Here is the code :
var arr = [-1, 2, -10, 8];
var min = arr[0];
var max = arr[0];
var average = 0;
for(var i = 0; i < arr.length; i++) {
if(arr[i] < min) min = arr[i];
if(arr[i] > max) max = arr[i];
average += arr[i];
}
average /= arr.length;
console.log("Min is : " + min);
console.log("Max is : " + max);
console.log("Average is : " + average);
Now Since Min and Max are part of the array they will always reflect the correct values of min and max. You can also use another algorithm :
- Sort Array
- Then min = arr[0] and max = arr[arr.length - 1];
- Use Same algorithm for average
Try to implement it If you have any Question let me know
William Li
Courses Plus Student 26,868 PointsHi, Jennifer Hughes , since the code isn't formatted using Markdown code block, it's a bit hard to read.
You are asking about min right? It's not a good idea to set it manually to some arbitrary number, because no matter how small the number you set it to be, there's chance your array will contain number smaller than that.
One way to do it is set min to be the first item of your array array[0]
, and then loop through the array, if there's an item smaller than the current min value, you reassign min to that value, when the loop ends, your min will contains the smallest number in the array; same goes true for the max.
This should get the job done.
var myArray = [1, 34, 23, 12, 6, 3];
var min = myArray[0];
var max = myArray[0];
var total = 0;
for (var i = 0; i < myArray.length; i++) {
if (myArray[i] < min) {min = myArray[i];}
if (myArray[i] > max) {max = myArray[i];}
total += myArray[i]
}
console.log(max);
console.log(min);
console.log(total/myArray.length);
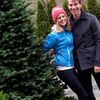
Jennifer Hughes
11,421 PointsThank you, William! Sorry about my formatting; I will fix that for future posts. (I have no idea what I even posted; it was not what the code I have written in my notes; strange). What I initially had was:
var x = [1, 3, 4, 23, 12, 6, 3];
var max = 0;
var min = 0;
var total =0;
for (var i = 0; i < x.length; i++) {
if (x[i] > max) {
max = x[i];
}
else if (x[i] < min) {
min = x[i];
}
total += x[i];
}
console.log(max);
console.log(min);
console.log(total/x.length);
So, with my above code, I ran into the problem of my min never being less than 0, and my max never greater than 0 if my array was made up of completely negative numbers. I see how you fixed that!
A follow up: I noticed both you and Mohammed removed the if/else statements and instead contained everything in the for statement. I don't believe I've learned this component of coding yet; do you mind expanding why you were able to do:
if (myArray[i] < min) {min = myArray[i];}
without any cod between the () and {}?
Thanks!
Jenn
William Li
Courses Plus Student 26,868 PointsHi, Jenn
if (myArray[i] < min) {min = myArray[i];}
// same as
if (myArray[i] < min) {
min = myArray[i];
}
You can even do it without {} if it's just one line statement
if (myArray[i] < min)
min = myArray[i];
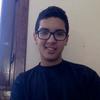
Mohammed Khalil Ait Brahim
9,539 PointsWilliam Li is right If your if statement only has one line you can omit the curly braces. It also works with for/while loops if you have one statement like :
for(var i = 0; i < 10; i++)
console.log(i);
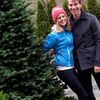
Jennifer Hughes
11,421 PointsExcellent, thanks for your help! Do you mind helping me through one more problem?
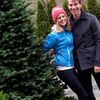
Jennifer Hughes
11,421 PointsIf I have an array with negative values, how do I remove the negative values?
Simply put:
var x = [-3, 5, 1, 3, 2, 10];
I want to remove -3 , and I don't want to create a temporary array.
Thanks again. Jenn
William Li
Courses Plus Student 26,868 Pointsx.splice(x.indexOf(-3), 1);
should get the job done.
William Li
Courses Plus Student 26,868 PointsI'd like to add that, if you have an array of numbers, and you wanna get rid of all negative numbers, the simplest way is still using for loop to loop over each item in the array, and append it to a new array if it's NOT negative.
If you are just doing so for the purpose of learning javascript, it's fine; but if you're building for a real web app, you generally don't wanna write such tedious JavaScript codes for such simple task, instead you should be using a JavaScript library, such as Underscore.js to do the job.
_.filter([-3, 5, 1, 3, 2, 10, -9], function(number) {return num>=0;});
// => [5, 1, 3, 2, 10]
That's Underscore's way of doing it, very clean and concise.
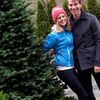
Jennifer Hughes
11,421 PointsI am doing this for learning Javascript; I am heading off to a bootcamp in a few weeks, and this is pre course material. I've resolved all of the problems but two, this being one of them. The exact directions read:
Given an array X of multiple values (e.g [-3, 5, 1, 3, 2, 10]), write a program that removes any negative values in the array. Do this without creating a temporary array and only using pop method to remove any values in the array.
Thank you again for your help, William!
Jennifer Hughes
11,421 PointsJennifer Hughes
11,421 PointsHi Mohammed.
Thanks for your help! Are you able to see what I responded to William? That is my follow-up question...
Cheers, Jenn