Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial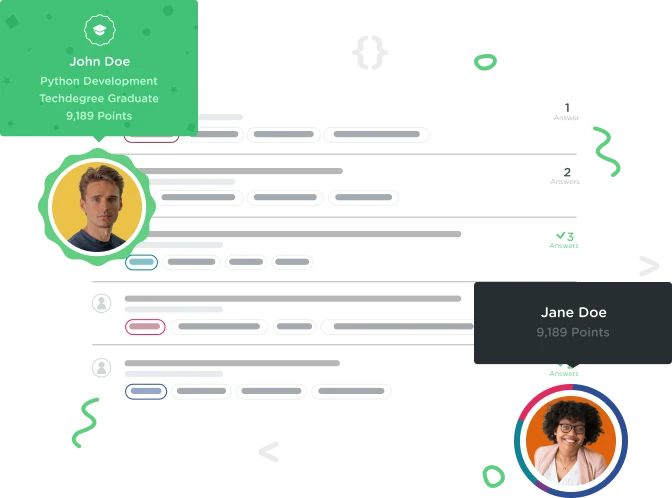
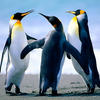
Toby Emmanuel
6,782 Pointsfinding it hard to complete this task
The code below logs all of the even numbers from 2 to 24 to the JavaScript console. However, there's a lot of redundant code here. Re-write this using a loop.
console.log(2);
console.log(4);
console.log(6);
console.log(8);
console.log(10);
console.log(12);
console.log(14);
console.log(16);
console.log(18);
console.log(20);
console.log(22);
console.log(24);
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
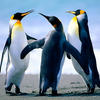
Toby Emmanuel
6,782 Points // Not sure if i have to declear a empty variable and then add it into the loop.. help :(
var argument;
for ( var i = 2; i <= 24; i += 2 ) {
argument += i;
return argument
}
console.log( parseInt(argument) );
/*
console.log(2);
console.log(4);
console.log(6);
console.log(8);
console.log(10);
console.log(12);
console.log(14);
console.log(16);
console.log(18);
console.log(20);
console.log(22);
console.log(24);
*/
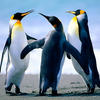
Toby Emmanuel
6,782 PointsI've been trying to work it out in workspace and I'm still stuck : s
5 Answers
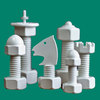
Steven Parker
231,269 PointsYou do appear to have the right idea, and your loop is good, but you have a few issues yet.
- you need to perform the console.log function inside the loop
- you'll want to pass the console.log function your "i" variable
- you can't use return when you're not inside a function
- you don't need the variable named argument for anything anyway
I'll bet you can get it now.
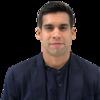
Johnny Garces
Courses Plus Student 8,551 Pointsthe break
statement is used to jump out of any loop. In this code, the program will console log 0, instead of printing 0-9.
for(var i = 0; i < 10; i++){
console.log(i);
break;
//will console log out 0
}
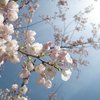
Kandance Ferguson
6,271 PointsYou declared a variable argument, however argument is undefined -- meaning that you did not assign argument to anything. The first time through the loop, argument = undefined. If you add argument + i --or-- undefined + 2, it will still be undefined, and undefined is not a number.
Return is illegal inside of a loop. It is used with functions.
Think of what you want to do with i. You can write exactly what you want to do with i inside of the loop so that the action happens each time through the loop.
Check out this repl.it link for an example of logging 1 - 10: https://repl.it/C9TZ
Good luck!
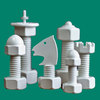
Steven Parker
231,269 Points The use of the return statement doesn't stop the loop, it causes an error.
Outside of a function, return is an illegal statement.
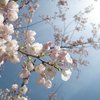
Kandance Ferguson
6,271 PointsThanks Steven, updated my answer!
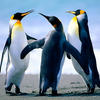
Toby Emmanuel
6,782 PointsThanks guys both have great answers and helped me a lot really appreciate the help!
Steven Parker
231,269 PointsSteven Parker
231,269 PointsIt doesn't look like you've written any code yet.
If you really have no idea where to start, you might want to re-watch the video on For Loops.