Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial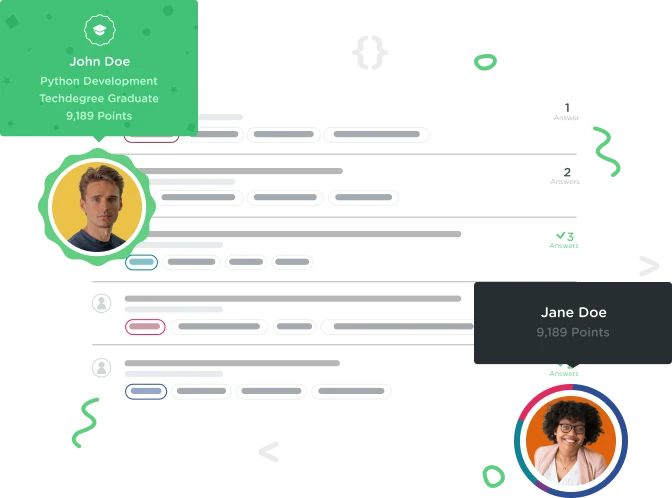

Daniel Merrill
2,888 PointsFinding the specific venue at a current location using foursquare API in swift
I'm newer to coding in swift and I'm working on finding a specific venue that corresponds to my current location using the foursquare API in the swift language. I've got it down to a cluster of venues around my location, but it only works sometimes when I run the simulator. Can someone please help me out? I have two ViewControllers. The first one:
import Foundation
import UIKit
import CoreLocation
let API_URL = "https://api.foursquare.com/v2/"
let CLIENT_ID = ""
let CLIENT_SECRET = ""
class FourSquareRequest: NSObject {
class func requestVenuesWithLocation(location: CLLocation) -> [AnyObject] {
let requestString = "\(API_URL)venues/search?client_id=\(CLIENT_ID)&client_secret=\(CLIENT_SECRET)&v=20130815&ll=\(location.coordinate.latitude),\(location.coordinate.longitude)"
println(requestString)
if let url = NSURL(string: requestString) {
let request = NSURLRequest(URL: url)
if let data = NSURLConnection.sendSynchronousRequest(request, returningResponse: nil, error: nil) {
if let returnInfo = NSJSONSerialization.JSONObjectWithData(data, options: .MutableContainers, error: nil) as? [String:AnyObject] {
let responseInfo = returnInfo["response"] as [String:AnyObject]
let venues = responseInfo["venues"] as [[String:AnyObject]]
return venues
}
}
}
return []
} }
And the second ViewController:
import UIKit
import MapKit
import CoreLocation
var onceToken: dispatch_once_t = 0
class VenueViewController: UIViewController, CLLocationManagerDelegate {
var lManager = CLLocationManager() var mapView = MKMapView()
override func viewDidLoad() { super.viewDidLoad()
mapView.frame = view.frame
view.addSubview(mapView)
lManager.requestWhenInUseAuthorization()
lManager.delegate = self
lManager.desiredAccuracy = kCLLocationAccuracyBest
lManager.distanceFilter = kCLDistanceFilterNone
lManager.startUpdatingLocation()
}
func locationManager(manager: CLLocationManager!, didUpdateLocations locations: [AnyObject]!) {
dispatch_once(&onceToken) { () -> Void in
println(locations.last)
if let location = locations.last as? CLLocation {
let span = MKCoordinateSpanMake(0.1, 0.1)
let region = MKCoordinateRegion(center: location.coordinate, span: span)
self.mapView.setRegion(region, animated: true)
let venues = FourSquareRequest.requestVenuesWithLocation(location)
println(venues)
self.createAnnotationsWithVenues(venues)
// request to foursquare for venues with location
}
}
lManager.stopUpdatingLocation()
}
func createAnnotationsWithVenues(venues: [AnyObject]) {
for venue in venues as [[String:AnyObject]] {
let locationInfo = venue["location"] as [String:AnyObject]
let lat = locationInfo["lat"] as CLLocationDegrees
let lng = locationInfo["lng"] as CLLocationDegrees
let coordinate = CLLocationCoordinate2DMake(lat, lng)
let annotation = MKPointAnnotation()
annotation.setCoordinate(coordinate)
mapView.addAnnotation(annotation)
}
} }