Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial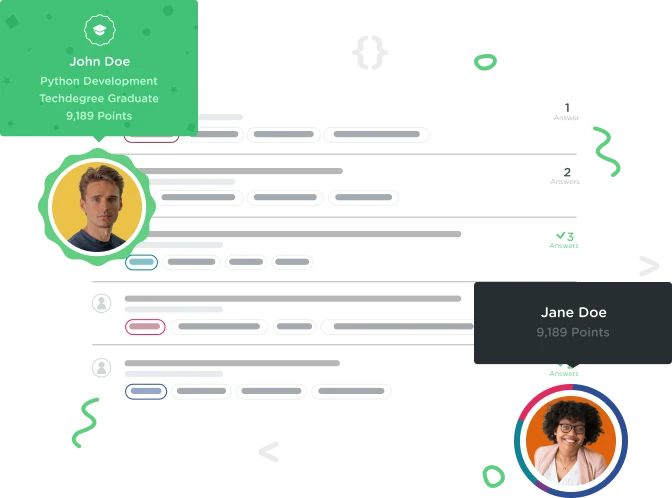

Elizabeth McInerney
3,175 Pointsfind_words
I can get this code to work if I cheat and put the number 6 into the findall, in place of a_count, but I cannot get it to work with a_count.
import re
# EXAMPLE:
# >>> find_words(4, "dog, cat, baby, balloon, me")
# ['baby', 'balloon']
def find_words(a_count, a_string):
return re.findall(r'\w{a_count,}',a_string)
3 Answers
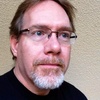
Chris Freeman
Treehouse Moderator 68,423 PointsIn the context of a regex string "6" will mean the range you wish, but "a_count" is just string characters and not the variable you think it is. The solution is to construct the string, or use format to pass a_count
into the regex:
# method 1:
def find_words(a_count, a_string):
return re.findall(r'\w{' + a_count + ',}', a_string)
# method 2 with format(). Need to escape '{' as '{{' and '}' as '}}'.
def find_words(a_count, a_string):
return re.findall(r'\w{{{0},}}'.format(a_count), a_string)

Elizabeth McInerney
3,175 PointsGlad you like my questions! Understanding why something works is the only way I can how it works.

Elizabeth McInerney
3,175 PointsOk, I just read your answer. Let's stick to the first example for now. What does ' + acount + ' mean?
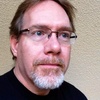
Chris Freeman
Treehouse Moderator 68,423 PointsIn the first answer, the quotes are around '\w{' and ',}' highlighted in a rust color , and not the a_count
highlighted in white.
These three parts (the two quoted bits and the variable a_count
) are combined using string concatenation ("adding") to form the string used as the regex.
Update: If a_count
is not already a string, then it would need to be converted using str(a_count)
as below:
def find_words(a_count, a_string):
return re.findall(r'\w{' + str(a_count) + ',}', a_string)
Elizabeth McInerney
3,175 PointsElizabeth McInerney
3,175 PointsOn the second answer, why is there a zero? Also, there seems to be an extra set of brackets. I don't understand
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsIn the first answer, counting quotes can be tricky. The quotes are around
'\w{'
and',}'
highlighted in a rust color , and not thea_count
highlighted in white.For the second answer, you have the collision of using curly brackets in regex and curly brackets for the format statement. To differentiate between the two, you need to double the curly brackets intended for the regex.
format()
treats a single curly bracket as a field marker, and a double-curly bracket as an escaped-curly bracket which gets returned as a regular single curly bracket in the output for the regex to use.The
0' in the
{0}.format()` field names the field as the first (0-th) item to be filled in. It is not necessary, since numbering the fields is optional. However it helps the field stand out from the crowd of curly brackets. A "named field" could have also been used:
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsBTW, Elizabeth McInerney , You ask great questions! More than "this doesn't work", but why a solution fixes the issue. It gets me out of using boilerplate answers and in to including the "why" of the solution.