Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial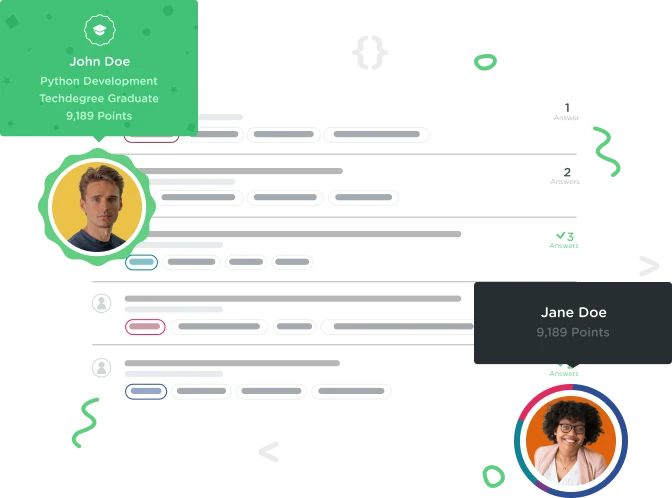

Samuel Piecz
8,143 PointsFinished Django Track. Trying to build my first project. What do I need to go back and learn?
I am attempting to upload an image via django admin and then display it within a template. The upload works, I think, however the displaying in the template part does not.
I receive the following error even though the files are in the directory the error mentions..
Not Found: /media/shrimp_sam_site.jpg Not Found: /media/shrimp_sam_screenshot.jpg
First project since completing the Django Track so I'm sure it doesn't look pretty. A push in the right direction would be immensely appreciated.
Here is my model:
from django.db import models
class Project(models.Model):
number = models.TextField(max_length="255", default='')
title = models.TextField(max_length="255", default='')
icon = models.ImageField(upload_to='')
description = models.TextField(max_length="255", default='')
picture = models.ImageField(upload_to='')
website = models.TextField(max_length="255", default='')
date_finished = models.TextField(max_length="255", default='')
service = models.TextField(max_length="255", default='')
def __str__(self):
return self.title
This from my understanding, is working. If I check my media directory the files are present.
However, referencing the {{ project.icon.url }} or {{ project.picture.url }} within my template does not work. The template looks inside the project apps directory instead of my media directory. So I attempted to click and drag the files in the directory it was searching for, then hard code the path in. That still didn't work so I'm assuming I may have to include a save method in my model?
Here is my template.
{% for project in projects %}
<div class="col-sm-4 portfolio-item">
<a href="#portfolioModal{{ project.number }}" class="portfolio-link" data-toggle="modal">
<div class="caption">
<div class="caption-content">
<i class="fa fa-search-plus fa-3x"></i>
</div>
</div>
<img src="{{ project.icon }}" class="img-responsive" alt="{{ project.title }}">
</a>
</div>
{% endfor %}
I have another for loop that also references the rest of projects attributes to popup a Modal, this works, and all the description and text based fields generate.
The images are the only things that don't.
I have also registered my models with the admin.
from django.contrib import admin
from .models import Project
admin.site.register(Project)
As well as made a url for my projects
from django.conf.urls import url
from . import views
urlpatterns = [
url(r'^$', views.projects),
]
Which references my view. That points to the broken directory.
from django.shortcuts import render
from .models import Project
def projects(request):
project = Project.objects.all()
return render(request, 'projects/project.html', {'projects': project})
Apologies in advance for the extra long post. I just am not quite sure what to do at this point. I've been stuck here for a few days.
Any help would be greatly appreciated.