Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial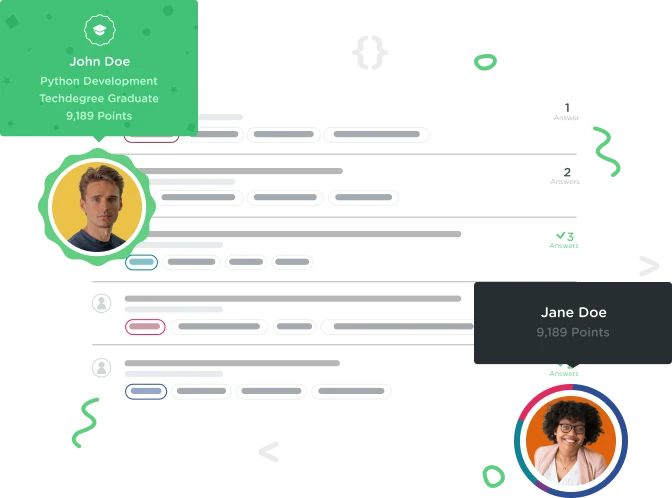

Danny Hartley
5,655 PointsFinishing TreeStory
This is the error I am receiving: "Did you prompt the user for the story (1)? I didn't see the first double underscored word prompted (2)."
package com.teamtreehouse;
import java.io.IOException; import java.util.Arrays; import java.util.List;
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
String story = null;
try {
story = prompter.promptForTemplate();
} catch (IOException ioe) {
ioe.printStackTrace();
System.exit(0);
}
Template tmpl = new Template(story);
prompter.run(tmpl);
}
}
package com.teamtreehouse;
import java.io.*; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.util.ArrayList; import java.util.HashSet; import java.util.List; import java.util.Set;
public class Prompter { private BufferedReader mReader; private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
System.out.println(tmpl.render(results));
}
public String promptForTemplate() throws IOException {
System.out.printf("Enter your template: ");
String template = mReader.readLine();
while (mCensoredWords.contains(template)) {
System.out.printf("%s is censored. Please enter another placeholder: ", template);
template = mReader.readLine();
}
return template;
}
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
public String promptForWord(String phrase) throws IOException {
System.out.printf("Enter the placeholder: ");
String word = mReader.readLine();
while (mCensoredWords.contains(word)) {
System.out.printf("%s is censored. Please enter another placeholder: ", word);
word = mReader.readLine();
}
return word;
}
}
Help Please?
2 Answers
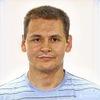
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsTwo things:
1. Don't print censored word when you censor it, otherwise Craig tests consider you failing in censoring:
// fails
// System.out.printf("%s is censored. Please enter another placeholder: ", word);
// should pass
System.out.println("word is censored. Please enter another word ");
2. You have to print placeholder, i.e. Please enter __name__
, and not just Please enter placeholder
// System.out.printf("Enter the placeholder: ");
System.out.printf("Enter the %s: ", phrase);

Eugenio Villarreal
8,041 PointsWhy does the code crash if I don't include in the promptForWord method :
word = mReader.readLine();
public String promptForWord(String phrase) throws IOException {
System.out.printf("Enter the %s: ", phrase);
String word = mReader.readLine();
while (mCensoredWords.contains(word)) {
System.out.println("word is censored. Please enter another word ");
word = mReader.readLine();
}
return word;
Haley Bengtson
Front End Web Development Techdegree Student 14,334 PointsHaley Bengtson
Front End Web Development Techdegree Student 14,334 PointsThank you for posting this! I originally had an issue with getting it to pass because I was printing "Enter the response: " rather than using a printf statement, which actually works very well since it loads in the type of response you should be giving.